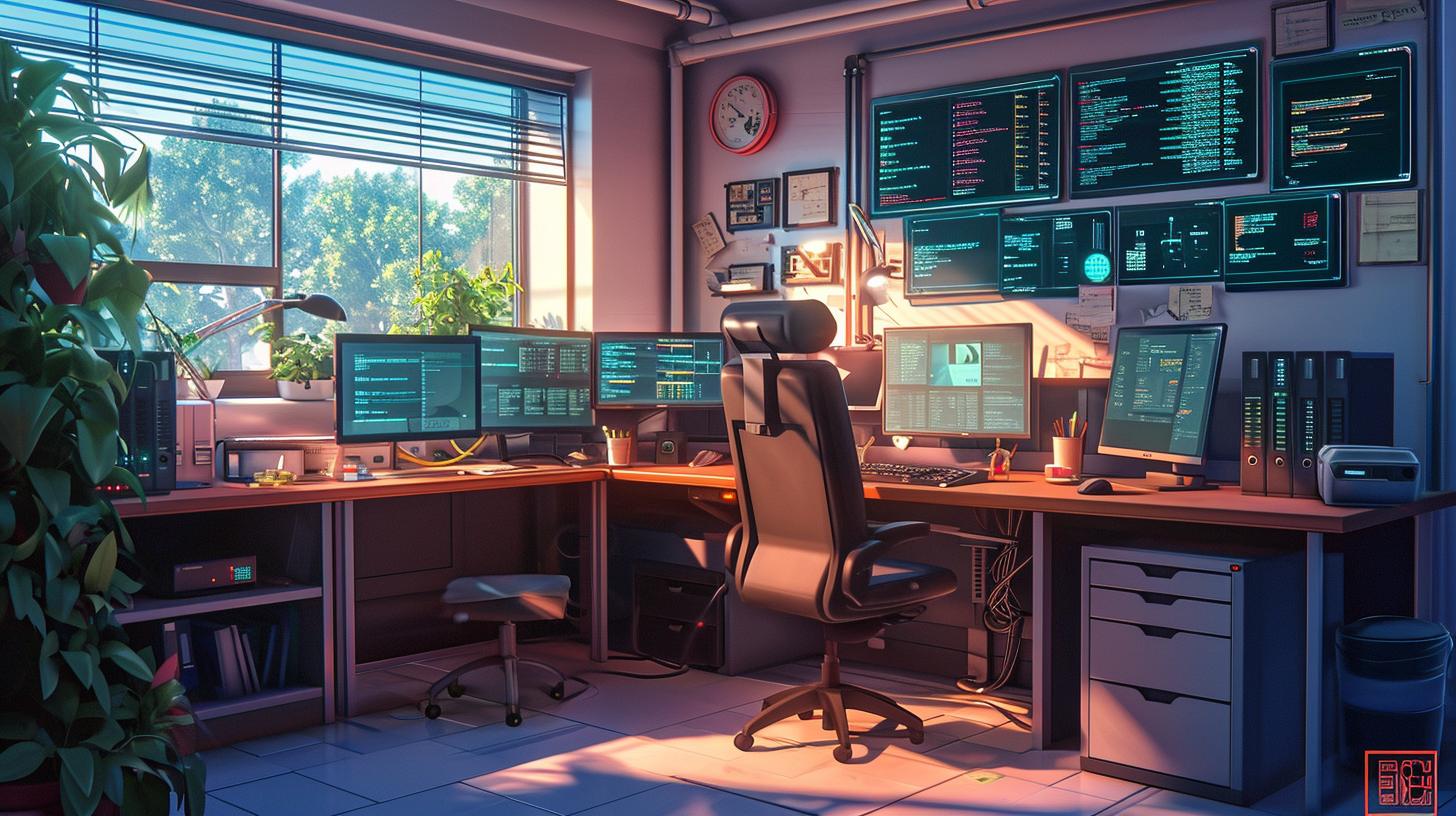
SQLite3 is a popular lightweight database engine that comes bundled with Python. When working with SQLite3 in Python, one of the powerful features available is the Row Factory. The Row Factory allows developers to customize how result rows are returned from database queries, providing enhanced flexibility and convenience in data handling.
By default, SQLite3 returns query results as tuples. While tuples are efficient, they can be less intuitive to work with, especially when dealing with complex queries or large datasets. That’s where the Row Factory comes into play, offering a more versatile approach to accessing and manipulating query results.
The basic usage of SQLite3’s Row Factory involves setting the row_factory
attribute of a database connection to sqlite3.Row
. This simple change transforms the way data is returned, which will allow you to access columns by name rather than just by index.
Here’s a simple example demonstrating the difference:
import sqlite3 # Create a connection and cursor conn = sqlite3.connect(':memory:') cursor = conn.cursor() # Create a sample table and insert data cursor.execute("CREATE TABLE users (id INTEGER, name TEXT, email TEXT)") cursor.execute("INSERT INTO users VALUES (1, 'Albert Lee', '[email protected]')") conn.commit() # Without Row Factory cursor.execute("SELECT * FROM users") row = cursor.fetchone() print(f"Without Row Factory: {row[1]}") # Access by index # With Row Factory conn.row_factory = sqlite3.Row cursor = conn.cursor() cursor.execute("SELECT * FROM users") row = cursor.fetchone() print(f"With Row Factory: {row['name']}") # Access by column name conn.close()
In this example, you can see how the Row Factory allows us to access the ‘name’ column directly by its name, making the code more readable and less prone to errors that might occur when relying on column positions.
The Row Factory is not limited to just using sqlite3.Row
. It provides a foundation for creating custom row objects, allowing developers to define their own classes or functions to handle query results. This extensibility opens up numerous possibilities for data processing, validation, and integration with other parts of your application.
Understanding and using the SQLite3 Row Factory is an important step towards writing more efficient and maintainable database-driven Python applications. It serves as a bridge between raw database results and more Pythonic data structures, paving the way for advanced data manipulation techniques.
Overview of Custom Row Objects
Custom row objects in SQLite3 provide a powerful way to transform database query results into more meaningful and convenient Python objects. These objects can encapsulate data and behavior, making it easier to work with database records in a more Pythonic and object-oriented manner.
When using custom row objects, each row returned from a database query is instantiated as an object of a user-defined class. This class can define properties, methods, and even implement custom logic for data validation or transformation.
The key components of custom row objects include:
- A custom class that represents a database row, typically with attributes corresponding to table columns.
- A function that creates instances of the custom class from database query results.
- Setting the connection’s row_factory attribute to use the custom factory function.
Here’s a basic example of how a custom row object might be structured:
class User: def __init__(self, id, name, email): self.id = id self.name = name self.email = email def __repr__(self): return f"User(id={self.id}, name='{self.name}', email='{self.email}')" def get_email_domain(self): return self.email.split('@')[1] def user_factory(cursor, row): return User(*row) # Usage conn.row_factory = user_factory cursor = conn.cursor() cursor.execute("SELECT * FROM users") user = cursor.fetchone() print(user) # User(id=1, name='Neil Hamilton', email='[email protected]') print(user.get_email_domain()) # example.com
In this example, the User class represents a row from the ‘users’ table. It includes a custom method get_email_domain() that extracts the domain from the email address. The user_factory function creates User instances from database rows.
Custom row objects offer several advantages:
- You can enforce types and add validation to ensure data integrity.
- Related data and behavior are bundled together in a single object.
- It’s easy to add new methods or properties to enhance functionality.
- Custom objects can seamlessly integrate with other parts of your application.
When designing custom row objects, think the following best practices:
- Keep the class focused on representing the database row, avoiding complex business logic.
- Use property decorators for computed attributes that don’t need to be stored in the database.
- Implement __repr__ and __str__ methods for better debugging and logging.
- Think using dataclasses or namedtuples for simple row representations.
Custom row objects are particularly useful in larger applications where you need to work with database records across multiple modules or when you want to add domain-specific behavior to your data models.
Creating Custom Row Objects
1. Define the Custom Row Class:
Start by creating a class that represents a row in your database table. This class should have attributes corresponding to the columns in your table and can include additional methods for data manipulation or validation.
class Product: def __init__(self, id, name, price, category): self.id = id self.name = name self.price = price self.category = category def __repr__(self): return f"Product(id={self.id}, name='{self.name}', price={self.price}, category='{self.category}')" def apply_discount(self, percentage): self.price *= (1 - percentage / 100) return self.price @property def is_expensive(self): return self.price > 1000
In this example, we’ve created a Product class with attributes matching our table columns. We’ve also added an apply_discount method and an is_expensive property to demonstrate how custom functionality can be included.
2. Create the Factory Function:
Next, define a factory function that will create instances of your custom class from database rows. This function will be used by SQLite3’s Row Factory.
def product_factory(cursor, row): columns = [column[0] for column in cursor.description] return Product(**dict(zip(columns, row)))
This factory function creates a dictionary from the column names and row values, then uses it to instantiate a Product object. This approach is flexible and will work even if the order or number of columns in your query changes.
3. Set Up the Database Connection:
Now, set up your database connection and assign the factory function to the row_factory attribute:
import sqlite3 conn = sqlite3.connect('store.db') conn.row_factory = product_factory cursor = conn.cursor()
4. Use the Custom Row Objects:
With everything set up, you can now query your database and work with the results as custom Product objects:
# Create a sample table and insert data cursor.execute(''' CREATE TABLE IF NOT EXISTS products (id INTEGER PRIMARY KEY, name TEXT, price REAL, category TEXT) ''') cursor.execute("INSERT INTO products VALUES (1, 'Laptop', 1200.00, 'Electronics')") cursor.execute("INSERT INTO products VALUES (2, 'Book', 15.99, 'Books')") conn.commit() # Query the database cursor.execute("SELECT * FROM products") products = cursor.fetchall() # Work with custom Product objects for product in products: print(product) if product.is_expensive: print(f"{product.name} is expensive!") new_price = product.apply_discount(10) print(f"New price after 10% discount: {new_price:.2f}") print() conn.close()
This code demonstrates how to use the custom Product objects. You can access attributes directly, use custom methods like apply_discount(), and utilize properties like is_expensive.
Additional Considerations:
- In the product class, you might want to ensure that id is an integer and price is a float. You can add type conversion in the __init__ method.
- You can add validation logic in the __init__ method or create separate validation methods.
- For frequently accessed computed properties, consider caching the results to improve performance.
- If your database has relationships between tables, you can add methods to fetch related objects.
By creating custom row objects, you can significantly enhance the way you work with database results in your Python applications, making your code more intuitive, maintainable, and aligned with object-oriented principles.
Implementing Custom Row Objects with SQLite3
Now that we have covered the basics of custom row objects, let’s dive into implementing them with SQLite3. This process involves integrating our custom objects with SQLite3’s row factory mechanism.
To implement custom row objects with SQLite3, we’ll follow these steps:
- Define the custom row class
- Create a factory function
- Set up the database connection with the custom row factory
- Execute queries and work with the custom objects
Let’s go through each step with a practical example:
1. Define the custom row class:
We’ll create a Customer class to represent rows from a customers table:
class Customer: def __init__(self, id, name, email, balance): self.id = id self.name = name self.email = email self.balance = float(balance) # Ensure balance is a float def __repr__(self): return f"Customer(id={self.id}, name='{self.name}', email='{self.email}', balance={self.balance:.2f})" def add_funds(self, amount): self.balance += amount return self.balance @property def is_premium(self): return self.balance > 1000
2. Create a factory function:
Next, we’ll define a factory function that creates Customer objects from database rows:
def customer_factory(cursor, row): columns = [column[0] for column in cursor.description] return Customer(**dict(zip(columns, row)))
3. Set up the database connection:
Now, we’ll create a database connection and set the row_factory to our custom factory function:
import sqlite3 conn = sqlite3.connect(':memory:') # Using in-memory database for this example conn.row_factory = customer_factory cursor = conn.cursor() # Create the customers table and insert sample data cursor.execute(''' CREATE TABLE customers (id INTEGER PRIMARY KEY, name TEXT, email TEXT, balance REAL) ''') cursor.executemany(''' INSERT INTO customers (name, email, balance) VALUES (?, ?, ?) ''', [ ('Alice Johnson', '[email protected]', 1500.00), ('Bob Smith', '[email protected]', 750.50), ('Charlie Brown', '[email protected]', 250.75) ]) conn.commit()
4. Execute queries and work with custom objects:
Finally, we can execute queries and work with the returned Customer objects:
# Fetch all customers cursor.execute("SELECT * FROM customers") customers = cursor.fetchall() for customer in customers: print(customer) if customer.is_premium: print(f"{customer.name} is a premium customer!") new_balance = customer.add_funds(100) print(f"New balance for {customer.name}: ${new_balance:.2f}") print() # Fetch a single customer cursor.execute("SELECT * FROM customers WHERE name = ?", ('Bob Smith',)) bob = cursor.fetchone() print(f"Bob's current balance: ${bob.balance:.2f}") bob.add_funds(300) print(f"Bob's new balance: ${bob.balance:.2f}") conn.close()
This implementation demonstrates how to fully integrate custom row objects with SQLite3. The Customer objects returned by our queries now have all the methods and properties we defined, allowing for more intuitive and powerful data manipulation.
Some key benefits of this approach include:
- The balance is automatically converted to a float in the Customer constructor.
- Related data and behavior (like add_funds) are bundled together.
- The is_premium property is calculated on-the-fly.
- You can easily add more methods or properties to the Customer class as needed.
When implementing custom row objects with SQLite3, ponder the following tips:
- Use type hints for better code readability and IDE support.
- Implement __eq__ and __hash__ methods if you need to use your objects in sets or as dictionary keys.
- Consider using dataclasses or attrs for more concise class definitions, especially for simpler objects.
- For large result sets, you might want to use cursor.fetchmany() instead of fetchall() to process rows in batches.
By using custom row objects with SQLite3, you can create a more robust and maintainable codebase, especially for larger applications or when working with complex data models.
Benefits of Using Custom Row Objects
1. Intuitive Data Access: Custom row objects allow you to access data using meaningful attribute names instead of numeric indices. This makes your code more readable and less prone to errors.
# With custom row objects print(user.name) # Without custom row objects (using tuples) print(user[1]) # Less intuitive, prone to errors if column order changes
2. Type Safety: You can enforce types and add validation in your custom row objects, ensuring data integrity and catching errors early.
class User: def __init__(self, id, name, age): self.id = int(id) self.name = str(name) self.age = int(age) if self.age < 0: raise ValueError("Age cannot be negative")
3. Encapsulation of Behavior: Custom row objects can include methods that operate on the data, encapsulating related functionality with the data it pertains to.
class Product: def __init__(self, id, name, price): self.id = id self.name = name self.price = price def apply_discount(self, percentage): self.price *= (1 - percentage / 100)
4. Computed Properties: You can add properties that compute values on-the-fly, reducing the need for additional database queries.
class Order: def __init__(self, id, items): self.id = id self.items = items @property def total_price(self): return sum(item.price for item in self.items)
5. Improved Code Organization: Custom row objects help in organizing code by grouping related data and behavior together, adhering to the principles of object-oriented programming.
6. Integration with Application Logic: Custom objects can seamlessly integrate with other parts of your application, making it easier to use database data throughout your codebase.
7. Flexibility and Extensibility: You can easily add new methods or properties to your custom row objects as your application’s needs evolve, without changing the underlying database schema.
8. Better Debugging: By implementing custom __repr__
or __str__
methods, you can make debugging easier with more informative object representations.
class User: def __repr__(self): return f"User(id={self.id}, name='{self.name}', email='{self.email}')"
9. Reduction in Boilerplate Code: Once set up, custom row objects can reduce the amount of repetitive code needed to work with database results.
10. Abstraction of Database Details: Custom row objects can provide a layer of abstraction over the raw database results, which will allow you to change the underlying database schema with minimal impact on the rest of your application.
By using these benefits, you can create more maintainable, readable, and robust database-driven applications. Custom row objects bridge the gap between relational database tables and object-oriented programming, allowing for a more natural and Pythonic way of working with your data.
Examples and Use Cases
1. E-commerce Product Catalog:
import sqlite3 class Product: def __init__(self, id, name, price, stock): self.id = id self.name = name self.price = float(price) self.stock = int(stock) def is_in_stock(self): return self.stock > 0 def apply_discount(self, percentage): self.price *= (1 - percentage / 100) def product_factory(cursor, row): columns = [column[0] for column in cursor.description] return Product(**dict(zip(columns, row))) conn = sqlite3.connect('ecommerce.db') conn.row_factory = product_factory cursor = conn.cursor() cursor.execute("SELECT * FROM products WHERE category = 'Electronics'") electronics = cursor.fetchall() for product in electronics: print(f"{product.name}: ${product.price:.2f}") if product.is_in_stock(): print("In stock") else: print("Out of stock") if product.price > 1000: product.apply_discount(10) print(f"Discounted price: ${product.price:.2f}") print()
2. User Management System:
import sqlite3 from datetime import datetime class User: def __init__(self, id, username, email, last_login): self.id = id self.username = username self.email = email self.last_login = datetime.fromisoformat(last_login) if last_login else None def days_since_last_login(self): if self.last_login: return (datetime.now() - self.last_login).days return None def is_active(self): return self.days_since_last_login() is not None and self.days_since_last_login() < 30 def user_factory(cursor, row): columns = [column[0] for column in cursor.description] return User(**dict(zip(columns, row))) conn = sqlite3.connect('users.db') conn.row_factory = user_factory cursor = conn.cursor() cursor.execute("SELECT * FROM users") users = cursor.fetchall() for user in users: print(f"User: {user.username}") print(f"Email: {user.email}") if user.is_active(): print("Active user") else: print("Inactive user") days = user.days_since_last_login() if days is not None: print(f"Last login: {days} days ago") else: print("Never logged in") print()
3. Financial Transaction Tracker:
import sqlite3 from decimal import Decimal class Transaction: def __init__(self, id, date, amount, category, description): self.id = id self.date = date self.amount = Decimal(amount) self.category = category self.description = description def is_expense(self): return self.amount < 0 def get_absolute_amount(self): return abs(self.amount) def transaction_factory(cursor, row): columns = [column[0] for column in cursor.description] return Transaction(**dict(zip(columns, row))) conn = sqlite3.connect('finances.db') conn.row_factory = transaction_factory cursor = conn.cursor() cursor.execute("SELECT * FROM transactions ORDER BY date DESC LIMIT 10") recent_transactions = cursor.fetchall() total_income = Decimal(0) total_expenses = Decimal(0) for transaction in recent_transactions: print(f"Date: {transaction.date}") print(f"Category: {transaction.category}") print(f"Description: {transaction.description}") print(f"Amount: ${transaction.get_absolute_amount():.2f}") if transaction.is_expense(): print("Type: Expense") total_expenses += transaction.get_absolute_amount() else: print("Type: Income") total_income += transaction.amount print() print(f"Total Income: ${total_income:.2f}") print(f"Total Expenses: ${total_expenses:.2f}") print(f"Net: ${(total_income - total_expenses):.2f}")
These examples demonstrate how custom row objects can be used in various scenarios to create more intuitive and powerful database-driven applications. They showcase the benefits of encapsulation, type safety, and the ability to add domain-specific methods to your data objects.
Source: https://www.pythonlore.com/using-sqlite3-row-factory-for-custom-row-objects/