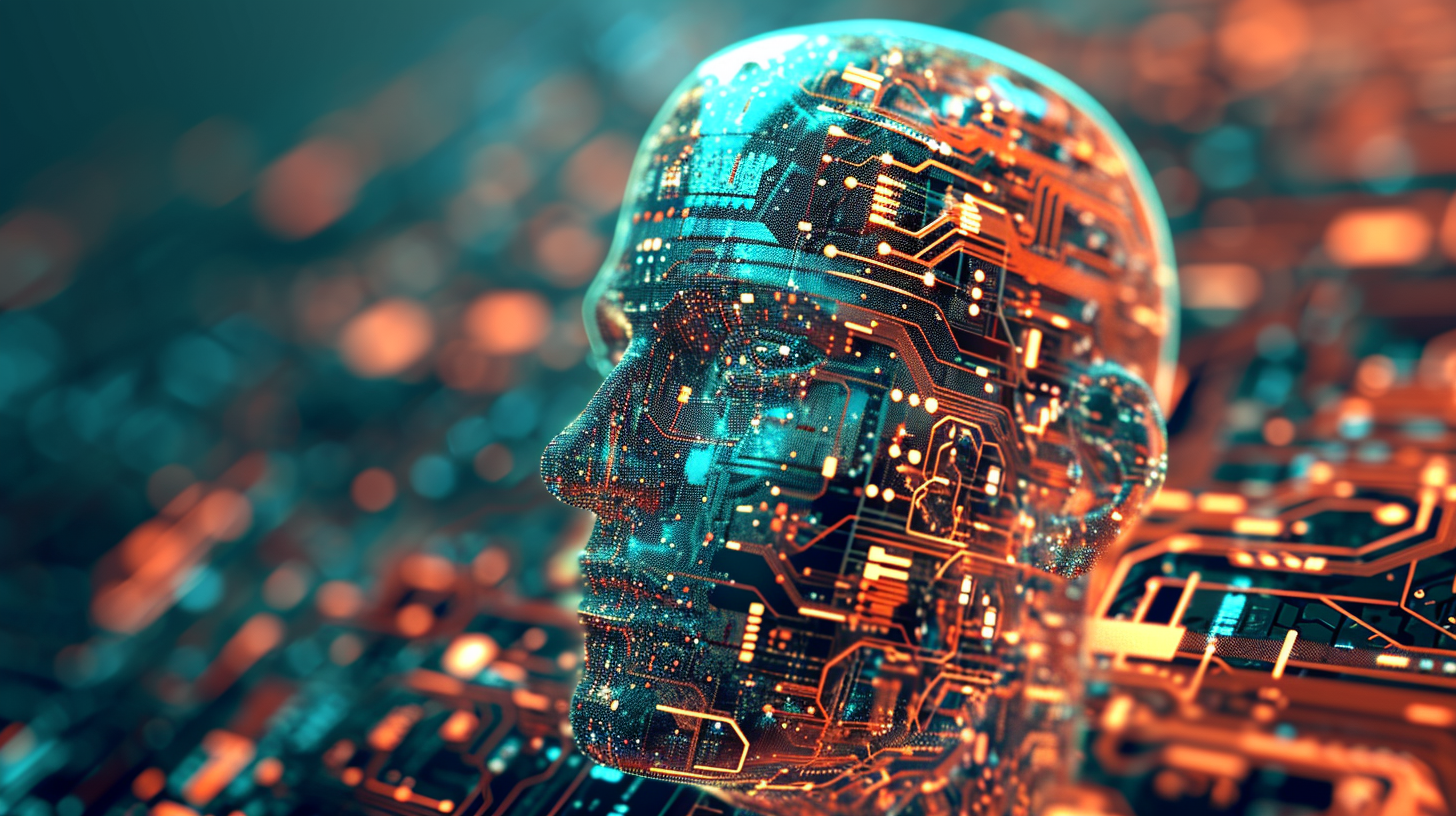
In the world of data analysis, pandas has become an essential library in Python due to its powerful tools for handling structured data. One of the advanced features of pandas is the MultiIndex
functionality, which allows for more complex data representations using multiple levels of indexing.
A MultiIndex
is essentially an array of tuples that gives a hierarchical representation of your data. This allows you to have a DataFrame or Series that can be indexed with more than one label, making it easier to work with higher-dimensional data in a two-dimensional structure. Below is a simple overview of a MultiIndex.
- A MultiIndex can have multiple levels of index. Each level serves as a categorization of the data. For instance, you may have a level for ‘Country’ and another for ‘State’.
- Each item in a MultiIndex is represented as a tuple, where each element corresponds to an index level. This arrangement allows for more granular and intuitive data selection.
- MultiIndex enhances data handling capabilities, especially for datasets that involve categories or hierarchical information, such as time series data across multiple dimensions.
The following example demonstrates how to create a simple MultiIndex
from existing arrays:
import pandas as pd # Creating arrays for MultiIndex arrays = [ ['A', 'A', 'B', 'B'], ['one', 'two', 'one', 'two'] ] # Creating MultiIndex object index = pd.MultiIndex.from_arrays(arrays, names=('letter', 'number')) # Creating DataFrame using MultiIndex df = pd.DataFrame({'value': [1, 2, 3, 4]}, index=index) print(df)
This will yield the following output:
value letter number A one 1 two 2 B one 3 two 4
In this example, we see that the DataFrame is indexed by two levels: ‘letter’ and ‘number’. Each tuple in the MultiIndex corresponds to a unique combination of these levels, providing a detailed view of the data.
Understanding how to utilize MultiIndex
is important for efficiently managing complex datasets. It allows analysts to perform operations on different levels, streamline data manipulation, and simplify grouping and aggregating processes. This level of abstraction creates a more manageable and efficient way to handle data with multiple dimensions.
Creating MultiIndex Objects from Scratch
Creating MultiIndex objects from scratch can be done in several ways, depending on how you want to structure your data. The most common method is to use a combination of arrays or lists to define the levels of the index. In this section, we will explore some of the methods available for constructing MultiIndex objects, along with practical examples to illustrate their usage.
One simpler way to create a MultiIndex is by using the pd.MultiIndex.from_arrays
method, as demonstrated previously. However, pandas also offers other methods such as pd.MultiIndex.from_tuples
and pd.MultiIndex.from_product
. These methods provide flexibility based on your specific data requirements.
Here’s a breakdown of these methods:
- Takes a list of arrays and creates a MultiIndex. Each array corresponds to a level in the MultiIndex.
- Takes a list of tuples where each tuple represents a combination of index levels. This is useful when you already know the combinations you need.
- Creates a MultiIndex from the cartesian product of provided iterables. This is particularly useful for creating indexes for all combinations of given categories.
Let’s explore these methods with example code:
Using pd.MultiIndex.from_tuples
:
import pandas as pd # Creating tuples for MultiIndex tuples = [ ('A', 'one'), ('A', 'two'), ('B', 'one'), ('B', 'two') ] # Creating MultiIndex object from tuples index_from_tuples = pd.MultiIndex.from_tuples(tuples, names=('letter', 'number')) # Creating DataFrame using MultiIndex df_tuples = pd.DataFrame({'value': [1, 2, 3, 4]}, index=index_from_tuples) print(df_tuples)
The output will be:
value letter number A one 1 two 2 B one 3 two 4
Now, let’s see how to create a MultiIndex using pd.MultiIndex.from_product
:
import pandas as pd # Creating lists for each level letters = ['A', 'B'] numbers = ['one', 'two'] # Creating MultiIndex object using product index_from_product = pd.MultiIndex.from_product([letters, numbers], names=('letter', 'number')) # Creating DataFrame using MultiIndex df_product = pd.DataFrame({'value': [1, 2, 3, 4]}, index=index_from_product) print(df_product)
The output will once again be:
value letter number A one 1 two 2 B one 3 two 4
These examples demonstrate the flexibility of creating MultiIndex objects according to your data structures. By using the appropriate method, you can represent your data more effectively, enhancing data manipulation and analysis capabilities in your projects. As you develop your skills in using pandas and MultiIndex, understanding these methods will significantly improve your ability to tackle sophisticated data analysis tasks.
Hierarchical Indexing: Structure and Benefits
Hierarchical indexing, enabled by pandas.MultiIndex, provides a structured way to manage and analyze high-dimensional data within a DataFrame or Series. Each level in a MultiIndex serves a unique purpose and allows for the representation of complex datasets in a way that remains intuitive and manageable. Below are some key features and benefits of hierarchical indexing:
- A MultiIndex can accommodate multiple levels of indices, enabling the organization of data hierarchically. For instance, using levels for ‘Year’, ‘Country’, and ‘Region’ can facilitate easier data analysis across different timeframes and geographic granularity.
- Each entry in a MultiIndex is represented as a tuple of labels corresponding to the levels of indexing. This representation allows for accessing subsets of data through clear and simple syntax. For example, you can easily extract all data regarding a specific year and country combination.
- With hierarchical indexing, it becomes simpler to filter data based on one or more levels. This capability simplifies operations like selecting slices of data, meaning you can retrieve only the relevant information without excessive overhead.
- Aggregating data becomes more efficient with MultiIndex, as you can group by levels independently. For instance, you might want to calculate the mean for all regions within a specific country and year.
- Datasets, such as those with measurements taken in different conditions or over various categories, can be effectively represented. Hierarchical indexing clarifies the relationships between data points, making the dataset easier to understand and analyze.
To illustrate the effectiveness of hierarchical indexing, consider the following example. Suppose we have sales data recorded in different years across various regions. We will structure this data to leverage the benefits of a MultiIndex:
import pandas as pd # Creating arrays for MultiIndex arrays = [ ['2021', '2021', '2022', '2022'], ['USA', 'Canada', 'USA', 'Canada'], ['East', 'West', 'East', 'West'] ] # Creating MultiIndex object index = pd.MultiIndex.from_arrays(arrays, names=('Year', 'Country', 'Region')) # Creating DataFrame using MultiIndex sales_data = pd.DataFrame({ 'Sales': [100, 150, 200, 250] }, index=index) print(sales_data)
The DataFrame produced will look like this:
Sales Year Country Region 2021 USA East 100 West 150 2022 USA East 200 West 250
With this MultiIndex, analysts can easily access data for specific years, countries, and regions in a nested manner. For example, to slice and view all sales data for Canada, you could use:
canada_sales = sales_data.loc[pd.IndexSlice[:, 'Canada', :], :] print(canada_sales)
This will yield:
Sales Year Country Region 2021 Canada East 150 West 250
Hierarchical indexing with pandas.MultiIndex not only improves data organization but also enhances the ease of data manipulation, filtering, and aggregation. This structured approach allows for more simpler data analysis processes, especially when dealing with complex, multi-dimensional datasets.
Advanced Slicing and Dicing with MultiIndex
Advanced slicing and dicing capabilities are one of the standout features of using a MultiIndex in pandas. By using hierarchical indexing, users can perform intricate data manipulations that are not only efficient but also intuitive. In this section, we will explore a variety of advanced slicing techniques in pandas using MultiIndex, demonstrating how to extract specific subsets or entire sections of data based on multiple criteria.
To showcase these capabilities, let’s start with a DataFrame that uses MultiIndex. Suppose we have a dataset consisting of sales data recorded by year, country, and region:
import pandas as pd # Creating arrays for MultiIndex arrays = [ ['2021', '2021', '2022', '2022'], ['USA', 'Canada', 'USA', 'Canada'], ['East', 'West', 'East', 'West'] ] # Creating MultiIndex object index = pd.MultiIndex.from_arrays(arrays, names=('Year', 'Country', 'Region')) # Creating DataFrame using MultiIndex sales_data = pd.DataFrame({ 'Sales': [100, 150, 200, 250] }, index=index) print(sales_data)
The produced DataFrame looks like this:
Sales Year Country Region 2021 USA East 100 West 150 2022 USA East 200 West 250
Let’s examine different ways to slice and dice this DataFrame:
- You can use the
.loc
accessor to retrieve data at specific index levels. For instance, to select all data for the year 2021:
year_2021_sales = sales_data.loc['2021'] print(year_2021_sales)
Sales Country Region USA East 100 West 150
canada_sales = sales_data.loc[pd.IndexSlice[:, 'Canada', :], :] print(canada_sales)
Sales Year Country Region 2021 Canada East 150 West 250
year_slice = sales_data.loc['2021':'2022'] print(year_slice)
Sales Year Country Region 2021 USA East 100 West 150 2022 USA East 200 West 250
high_sales = sales_data[sales_data['Sales'] > 150] print(high_sales)
Sales Year Country Region 2022 Canada West 250
These advanced slicing techniques using pandas.MultiIndex allow for greater flexibility in data analysis. You can navigate through complex datasets easily and extract meaningful insights efficiently. This enables analysts to focus on specific data points and derive valuable information without complicated filtering processes.
Reshaping Data: Stacking and Unstacking
Reshaping data with pandas.MultiIndex involves two key operations: stacking and unstacking. These operations allow users to manipulate a MultiIndex DataFrame’s structure, enabling greater flexibility in how data is presented and analyzed. Stacking transforms the DataFrame by tightening the index and consolidating columns into a lower index level, while unstacking does the reverse by widening the DataFrame and turning the lower index levels into columns.
Let’s begin with the stacking operation. When you have a MultiIndex DataFrame, stacking will pivot the innermost column index level to the row index levels. This is particularly useful when you want to simplify your DataFrame for more simpler analysis or visualization.
import pandas as pd # Creating arrays for MultiIndex arrays = [ ['2021', '2021', '2022', '2022'], ['USA', 'Canada', 'USA', 'Canada'] ] # Creating MultiIndex object index = pd.MultiIndex.from_arrays(arrays, names=('Year', 'Country')) # Creating DataFrame using MultiIndex with columns as regions df = pd.DataFrame({ 'East': [100, 150, 200, 250], 'West': [110, 160, 210, 260] }, index=index) print("Original DataFrame:") print(df) # Stacking the DataFrame stacked_df = df.stack() print("nStacked DataFrame:") print(stacked_df)
The output will be:
Original DataFrame: East West Year Country 2021 USA 100 110 Canada 150 160 2022 USA 200 210 Canada 250 260 Stacked DataFrame: Year Country 2021 USA East 100 West 110 Canada East 150 West 160 2022 USA East 200 West 210 Canada East 250 West 260 dtype: int64
In this example, by stacking the original DataFrame, we moved the innermost columns (‘East’ and ‘West’) into a lower level of the index, resulting in a more compact representation of our data. This transformation can be highly beneficial when working with time-series data where subsequent analysis might focus on specific dimensions.
On the other hand, unstacking is used to convert the stacked DataFrame back to a wider format. This is useful for visualizations, comparisons, and when you want to revert to the original DataFrame layout.
# Unstacking the stacked DataFrame unstacked_df = stacked_df.unstack() print("nUnstacked DataFrame:") print(unstacked_df)
The output of the unstacked DataFrame will resemble the original structure:
Unstacked DataFrame: Year Country East West 2021 USA 100 110 Canada 150 160 2022 USA 200 210 Canada 250 260
In this unstacked DataFrame, we can see that the operations have successfully reverted back to the original DataFrame format. When reshaping data, it is important to ponder the implications of stacking and unstacking, as these operations alter the DataFrame’s layout but not its underlying data.
Stacking and unstacking offer clear pathways to reshape your DataFrame based on your analytical needs, enabling more intuitive data presentation and facilitating efficient data analysis workflows. These operations are not only powerful but also essential for efficiently managing and visualizing complex datasets using pandas.MultiIndex.
MultiIndex and GroupBy: Aggregating Data Efficiently
When working with MultiIndex DataFrames in pandas, one of the most powerful features at your disposal is the GroupBy functionality. Grouping and aggregating data with MultiIndex allows for efficient summarization and analysis based on complex, multi-level categories. This section will explore how to utilize MultiIndex in conjunction with GroupBy to achieve sophisticated data aggregation.
GroupBy operations involve splitting the data into groups based on some criteria, applying a function to each group independently, and then combining the results back into a DataFrame or Series. When using a MultiIndex, you can take advantage of the hierarchical structure to perform more granular aggregations across different levels of the index.
Let’s think a practical example with some sales data represented in a MultiIndex DataFrame. We will aggregate the sales data by applying different functions (like sum and mean) to showcase how easy it is to summarize data based on hierarchical indices.
import pandas as pd # Creating a MultiIndex DataFrame arrays = [ ['2021', '2021', '2022', '2022'], ['USA', 'Canada', 'USA', 'Canada'], ] index = pd.MultiIndex.from_arrays(arrays, names=('Year', 'Country')) # Creating the DataFrame with sales amount sales_data = pd.DataFrame({ 'Sales': [100, 150, 200, 250], 'Returns': [10, 5, 20, 30] }, index=index) print("Original Sales Data:") print(sales_data) # Grouping by Year to sum Sales and Returns grouped_sales = sales_data.groupby(level='Year').sum() print("nGrouped by Year - Sum of Sales and Returns:") print(grouped_sales) # Grouping by Country to find mean sales mean_sales = sales_data.groupby(level='Country').mean() print("nGrouped by Country - Mean of Sales and Returns:") print(mean_sales)
The output for the original sales DataFrame would look like this:
Original Sales Data: Sales Returns Year Country 2021 USA 100 10 Canada 150 5 2022 USA 200 20 Canada 250 30
After grouping by Year, the aggregation will be shown as:
Grouped by Year - Sum of Sales and Returns: Sales Returns Year 2021 250 15 2022 450 50
Each year’s total sales and return values are now clearly summarized. Similarly, when we group by Country, we can see the mean values:
Grouped by Country - Mean of Sales and Returns: Sales Returns Country USA 150.0 15.0 Canada 200.0 17.5
In this example, aggregating by different levels of the MultiIndex illustrates the flexibility of the GroupBy functionality. You can change the level of aggregation easily by specifying different levels, which allows for customized insights depending on your analysis requirements.
Moreover, you can use multiple aggregating functions in a single operation by making use of the agg method. This enables presenting multiple metrics in one go, providing a more comprehensive view of your data. Here’s a small modification to the previous example:
# Grouping by Year and applying multiple aggregations aggregated_sales = sales_data.groupby(level='Year').agg({'Sales': ['sum', 'mean'], 'Returns': ['sum', 'mean']}) print("nGrouped by Year with Multiple Aggregations:") print(aggregated_sales)
The results will yield a DataFrame containing both sum and mean values for each metric, categorized by year:
Grouped by Year with Multiple Aggregations: Sales Returns sum mean sum mean Year 2021 250.0 125.0 15.0 7.5 2022 450.0 225.0 50.0 25.0
This showcases the power of the GroupBy functionality in conjunction with MultiIndex, allowing aggregated insights and facilitating effective data analysis. By using these techniques, analysts can derive rich insights from their datasets, ultimately leading to informed decision-making and strategic planning.
Best Practices for Managing MultiIndex DataFrames
Managing MultiIndex DataFrames requires an understanding of how to effectively manipulate and streamline your data for optimal performance and clarity. Below are some best practices for working with MultiIndex in pandas that can help improve your data handling efficiency and maintainability.
- It is essential to employ clear and descriptive names for each level of your MultiIndex. This not only improves the readability of your DataFrame but also makes it easier to reference and interact with the index levels. For example, using names like ‘Date’, ‘Country’, and ‘Product’ helps convey the meaning of the data better than generic labels.
- When performing operations on a MultiIndex DataFrame, use index slicing with the .loc method to access specific subsets of your data efficiently. This reduces overhead and speeds up processing times. For instance, if you’re interested in data for a specific country and year, you can retrieve the slice like this:
country_data = sales_data.loc[pd.IndexSlice['2022', 'Canada'], :]
pd.MultiIndex.from_frame
when you are ready to analyze the data hierarchically.sort_index
method. This ensures that your data is organized predictably and helps avoid errors when performing operations that rely on the index ordering.sorted_df = df.sort_index()
flattened_df = df.reset_index() # Turns MultiIndex into columns
df_copy = df.copy()
By employing these best practices, you can enhance your productivity when working with MultiIndex DataFrames in pandas. Efficient management of MultiIndex can significantly improve data analysis workflows, leading to more insightful analyses and clearer communication of results.
Source: https://www.pythonlore.com/advanced-indexing-with-pandas-multiindex/