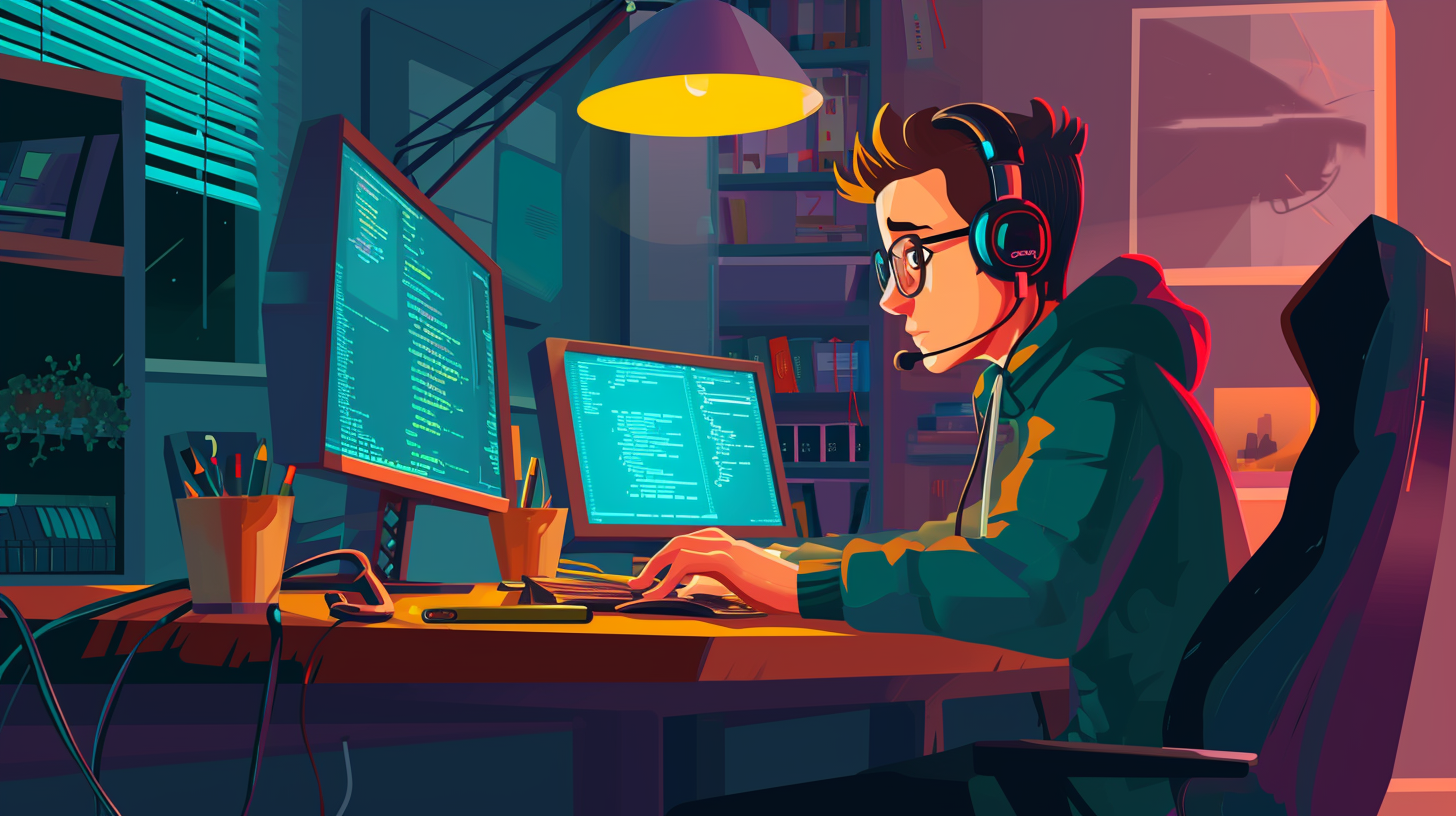
Regular expressions (regex) are powerful tools for matching patterns in strings, allowing for advanced searching and manipulation of text in Python. The re module provides full support for regular expressions, enabling complex queries to be executed with ease. Understanding how to construct and utilize these expressions is key to using the capabilities of the re module effectively.
A regular expression is essentially a sequence of characters that defines a search pattern. These patterns can range from simple to complex, depending on the task at hand. In Python, regex patterns are used primarily for searching, replacing, and splitting strings.
Here are some fundamental concepts and components of regular expressions:
- These are the simplest elements of regex and represent the exact text they match. For example, the regex
hello
will match the string “hello”. - Characters that have special meanings in regex. For example,
.
matches any single character, while*
matches zero or more occurrences of the preceding element. - Defined by square brackets
[ ]
, they allow matching any one of a set of characters. For instance,[abc]
will match ‘a’, ‘b’, or ‘c’. - Used to denote positions in a string, such as
^
for the start and$
for the end. For example,^hello
matches “hello” only if it occurs at the beginning of the string. - Specify how many instances of a character or group must be present for a match. Common quantifiers include
+
(one or more),?
(zero or one), and{n}
(exactly n times). - Parentheses
( )
are used to group portions of patterns and can also capture the matched text for further use.
Regular expressions are typically enclosed in raw string literals to avoid issues with escape characters. In Python, that’s accomplished by prefixing the string with an r:
pattern = r'd{3}-d{2}-d{4}' # Matches a pattern like 123-45-6789
When constructing regex patterns, it’s important to consider the specific requirements of your use case, as well as the nuances of regex syntax. This flexibility and expressiveness make regular expressions a valuable asset in any Python developer’s toolkit. Understanding these foundational concepts is important as we move on to using the re.search function to find matches within strings.
The Basics of re.search Function
The re.search
function is one of the most commonly used methods in the Python re module for searching through strings. It scans the input string, looking for a location that matches a specified pattern. If a match is found, it returns a match object; otherwise, it returns None. Understanding how to effectively use this function is essential for anyone looking to perform regex-based searches.
Here’s a basic syntax for the re.search
function:
re.search(pattern, string, flags=0)
- This is the regex pattern you want to search for, which can be defined as a raw string.
- The input string in which you want to search for the pattern.
- Optional parameter which allows you to modify certain aspects of the pattern matching (e.g.,
re.IGNORECASE
for case-insensitive matching).
Here’s a simple example demonstrating the use of re.search
:
import re # Define the pattern and the string pattern = r'd+' # Matches one or more digits string = 'The year 2023 is almost over.' # Use re.search to find the match match = re.search(pattern, string) if match: print("Match found:", match.group()) else: print("No match found.") # Output: Match found: 2023
In this example, the regex pattern d+
is used to search for one or more digits in the input string. The match.group()
method retrieves the matched substring.
In cases where there may be multiple possible matches, re.search
will return the first match it finds. If you need to find all occurrences, you would typically use re.findall
or re.finditer
instead. Below is an example to illustrate this.
# Using re.findall to get all matches all_matches = re.findall(r'd+', string) print("All matches found:", all_matches) # Output: All matches found: ['2023']
It’s important to note that the match object returned by re.search
includes various methods to extract additional information:
- Returns the substring matched by the pattern.
- Returns the starting index of the match in the string.
- Returns the ending index of the match in the string.
- Returns a tuple containing the start and end indices of the match.
Here’s an example demonstrating the use of these methods:
# Continuing from the previous example if match: print("Matched string:", match.group()) # Output: Matched string: 2023 print("Start index:", match.start()) # Output: Start index: 8 print("End index:", match.end()) # Output: End index: 12 print("Span:", match.span()) # Output: Span: (8, 12)
The re.search
function is a flexible and invaluable tool for pattern matching in strings. As we explore more advanced features and variations in regex, mastering this function will enhance your ability to manipulate and analyze text efficiently.
Pattern Matching Techniques
Pattern matching in Python using regular expressions can be very powerful and flexible. The way you structure your regex patterns determines the success of your searches, and there are several techniques you can employ to enhance your pattern matching capabilities. Below are some of the key techniques to think when working with regex.
- As previously mentioned, using anchors like ^ (beginning of a string) and $ (end of a string) helps you to specify the exact position in the string for a match. For example:
pattern = r'^Hello' # Matches "Hello" only if it's at the start of the string
pattern = r'he..o' # Matches any five-character string that starts with "he" and ends with "o"
pattern = r'(abc)+' # Matches one or more occurrences of "abc"
pattern = r'(?:abc|def)ghi' # Matches "abcghi" or "defghi"
pattern = r'd(?= dollars)' # Matches a digit followed by " dollars", but does not include " dollars" in the returned match
pattern = r'(w+) 1' # Matches any word that appears twice in succession
Combining these techniques can help create more advanced and tailored patterns for specific text processing tasks. Here is a practical example that demonstrates the use of some of these techniques:
import re # Example string text = "The quick brown fox jumps over the lazy dog." # We want to find all words that start with a vowel and end with a consonant pattern = r'b[aeiouAEIOU]w*[^aeious]b' matches = re.findall(pattern, text) print("Words that start with a vowel and end with a consonant:", matches)
In the example above, the regex pattern uses word boundaries (b) to anchor the match at the start and end of each word, ensuring it captures only whole words. It also uses character classes to specify conditions for the starting and ending characters. Mastering these techniques will greatly enhance your ability to perform complex searches and manipulations in Python, making you a more effective developer.
Common Use Cases for re.search
When it comes to practical applications of the re.search function, there are numerous common use cases that illustrate its versatility and effectiveness in string manipulation and pattern recognition. Below are some scenarios where re.search proves to be particularly useful:
- One of the most frequent use cases for re.search is validating email addresses. By defining a regex pattern that checks for a general email structure, you can verify whether a given string conforms to expected email formatting.
import re email_pattern = r'^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+.[a-zA-Z]{2,}$' email = "[email protected]" if re.search(email_pattern, email): print("Valid email address.") else: print("Invalid email address.") # Output: Valid email address.
date_pattern = r'bd{1,2}/d{1,2}/d{4}b' # Matches dates like 12/31/2023 text = "The event is scheduled for 12/31/2023." match = re.search(date_pattern, text) if match: print("Date found:", match.group()) # Output: Date found: 12/31/2023
phone_pattern = r'b(?d{3})?[-.s]?d{3}[-.s]?d{4}b' # Matches formats like (123) 456-7890 or 123-456-7890 phone_text = "You can reach me at (123) 456-7890 or 987-654-3210." phones = re.findall(phone_pattern, phone_text) print("Phone numbers found:", phones) # Output: Phone numbers found: ['(123) 456-7890', '987-654-3210']
keyword_pattern = r'bPythonb' text = "I am learning Python programming." if re.search(keyword_pattern, text): print("Keyword found!") # Output: Keyword found!
url_pattern = r'https?://[^s]+' web_content = "Visit our site at https://www.example.com for more info." url_match = re.search(url_pattern, web_content) if url_match: print("URL found:", url_match.group()) # Output: URL found: https://www.example.com
cleaning_pattern = r'[^ws]' # Matches any non-word character except whitespace dirty_text = "Hello, World! Let's clean this text!!" cleaned_text = re.sub(cleaning_pattern, '', dirty_text) print("Cleaned text:", cleaned_text) # Output: Cleaned text: Hello World Lets clean this text
These examples demonstrate the practical applications of the re.search function in a variety of contexts, highlighting its importance in tasks that involve pattern matching, validation, and data extraction. As you endeavor to apply regular expressions in your projects, ponder these use cases to imropve your string processing capabilities.
Troubleshooting and Best Practices
Troubleshooting and effectively using regular expressions with the re.search function can occasionally pose challenges, especially when dealing with complex patterns. However, applying best practices can streamline your development process and help you quickly resolve common issues. Here are some tips and tricks to assist you in this regard:
- Before implementing a regex pattern in your code, utilize online regex testers or Python interactive environments (like Jupyter Notebook) to experiment with your patterns. This can help you visualize matches and refine your expressions.
- When a regex pattern becomes too complex, using the re.VERBOSE flag can greatly enhance readability. This allows you to write multi-line regex patterns and include comments. For instance:
import re pattern = r""" ^ # Start of the string [a-zA-Z0-9._%+-]+ # Username part @ # Symbol '@' [a-zA-Z0-9.-]+ # Domain name .[a-zA-Z]{2,} # Domain suffix $ # End of the string """ email_pattern = re.compile(pattern, re.VERBOSE) email = "[email protected]" if email_pattern.search(email): print("Valid email address.") else: print("Invalid email address.")
date_pattern = r'bd{1,2}/d{1,2}/d{4}b' # Matches dates like 12/31/2023
This practice helps maintain clarity, especially when you revisit your code.
greedy_pattern = r'' # Greedy match for HTML tags non_greedy_pattern = r'' # Non-greedy match for HTML tags
import re pattern = r'd+' # Matches one or more digits string = 'The year 2023 is almost over.' match = re.search(pattern, string) if match: print("Match found at index:", match.start()) # Output: Match found at index: 8 else: print("No match found.")
try: result = re.search(pattern, string) # Further processing... except re.error as e: print("Regex error:", e)
By adhering to these best practices and troubleshooting tips, you can significantly improve your experience working with regular expressions in Python, ensuring that your pattern matching is both efficient and accurate.
Source: https://www.pythonlore.com/using-re-search-for-searching-strings/