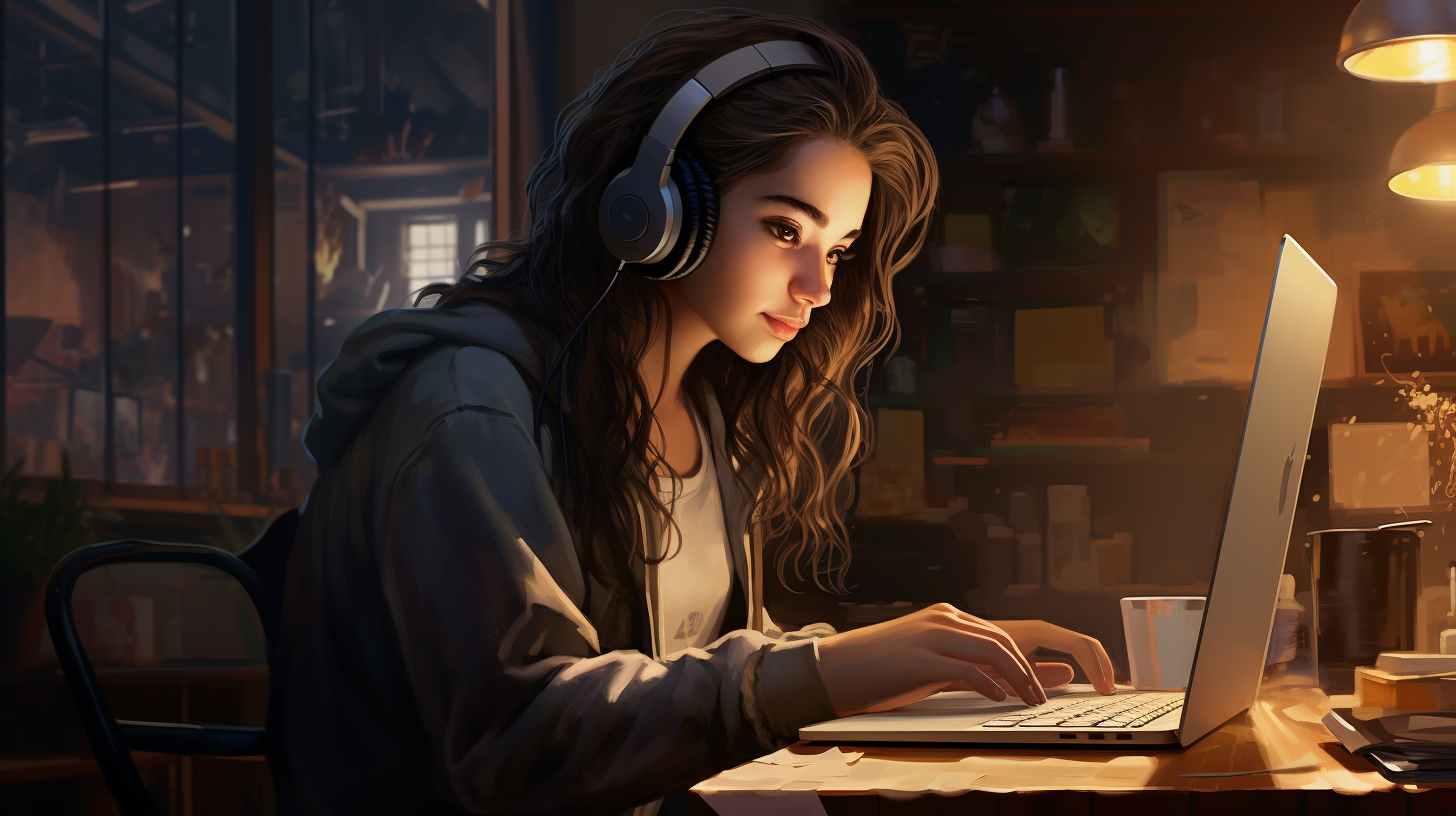
Object-Relational Mapping (ORM) is a programming technique that facilitates the interaction between object-oriented programming languages and relational databases. It acts as a bridge that allows developers to manipulate database records using object-oriented syntax, rather than writing complex SQL queries. This abstraction is particularly useful for developers who are more familiar with programming paradigms than with SQL syntax.
ORM concepts help to visualize the database structure as objects, where each object represents a row in a database table, and attributes of that object correspond to columns in that table. Here are some fundamental concepts of ORM:
- Entities: In ORM, entities are the objects that map to tables in the database. Each entity usually corresponds to a single table, and instances of that entity represent rows within that table.
- Attributes: Attributes of an entity are the properties that correspond to the columns of the table. In Python, these attributes can be defined using class variables.
- Relationships: ORM also allows establishing relationships between different entities. Common relationships include one-to-many (a single entity linked to multiple entities) and many-to-many (many entities linked to many others).
- Sessions: A session is a primary interface for working with the ORM. It allows developers to interact with objects, manage persistence, and facilitate transactions.
Considering these concepts, let’s take a look at a simple Python code example that illustrates how to define an entity using SQLAlchemy ORM:
from sqlalchemy import create_engine, Column, Integer, String from sqlalchemy.ext.declarative import declarative_base Base = declarative_base() class User(Base): __tablename__ = 'users' id = Column(Integer, primary_key=True) name = Column(String) age = Column(Integer) def __repr__(self): return f"" # Here we create the database engine engine = create_engine('sqlite:///:memory:') Base.metadata.create_all(engine)
In this code snippet:
- The
Base
class is created usingdeclarative_base()
, which serves as the foundation for defining database models. - The
User
class corresponds to theusers
table in the database. The attributesid
,name
, andage
represent the columns of this table. - The
__repr__
method is overridden for easier string representation of objects. - A database engine is created, in this case, using an in-memory SQLite database, and the metadata is initialized with
Base.metadata.create_all(engine)
to generate the associated tables.
Understanding these ORM concepts is vital for effectively using SQLAlchemy in your projects.
Installing SQLAlchemy
To start using SQLAlchemy ORM in your Python projects, the first step is to install the SQLAlchemy package. Installing SQLAlchemy is simpler and can be accomplished through the Python package management system, pip. Below are the detailed instructions for different environments.
-
Using pip: The easiest way to install SQLAlchemy is via pip. You can install it for your current Python environment by running the following command in your terminal or command prompt:
pip install SQLAlchemy
Note: If you are using a virtual environment, make sure to activate it before running the command to ensure that SQLAlchemy is installed in the correct environment.
-
Using a Requirements File: If you’re working on a project where you manage dependencies with a `requirements.txt` file, you can add SQLAlchemy to that file. Simply include the following line:
SQLAlchemy
Then, install all the dependencies by running:
pip install -r requirements.txt
-
Using Conda: If you’re using Anaconda as your Python distribution, you can install SQLAlchemy from the Anaconda repository using the following command:
conda install -c anaconda sqlalchemy
-
Verifying the Installation: After installation, you can verify that SQLAlchemy was installed successfully by checking its version. Open a Python shell or script and run:
import sqlalchemy print(sqlalchemy.__version__)
If it displays the version number without any errors, your installation was successful.
Once SQLAlchemy is successfully installed, you’re ready to start using its features for your Object-Relational Mapping needs. Be sure to install the appropriate database driver for your chosen database (e.g., psycopg2 for PostgreSQL, mysqlclient for MySQL) as SQLAlchemy relies on these drivers to connect to your database.
Setting Up a Database Connection
To connect to a database using SQLAlchemy, you need to establish a database connection. The connection is specified using a URL that indicates the type of database and the credentials required to access it. SQLAlchemy supports multiple database types, including SQLite, PostgreSQL, MySQL, and more. Below are the steps to set up a database connection.
The first step is to create an engine, which serves as the starting point for any SQLAlchemy application. The engine is responsible for managing connections to the database and executing SQL commands. Here’s how to set up a connection to different databases:
- For a SQLite database, you can use the following connection URL format:
engine = create_engine('sqlite:///path_to_your_database.db')
In this example, replace path_to_your_database.db
with the location of your SQLite database file. If you want to create a new database in memory, you can use:
engine = create_engine('sqlite:///:memory:')
- To connect to a PostgreSQL database, you would use the following URL format:
engine = create_engine('postgresql://username:password@localhost:5432/database_name')
Make sure to replace username
, password
, and database_name
with your actual database credentials and name.
- For a MySQL database, the connection string looks similar:
engine = create_engine('mysql+pymysql://username:password@localhost:3306/database_name')
In this case, ensure that you have pymysql
installed as it’s a driver used for MySQL connections.
Once the engine is created, it’s essential to confirm that the connection to the database is successful. This can be done using the connect()
method of the engine, which creates a connection and closes it immediately after to check if there are any issues.
connection = engine.connect() connection.close()
If no errors are thrown during this process, your database connection is established successfully. Do remember to handle exceptions appropriately to catch connection issues.
Setting up a database connection with SQLAlchemy involves creating an engine with the correct database URL, and optionally testing that connection. This allows you to begin interacting with your database using the powerful SQLAlchemy ORM capabilities.
Defining Database Models
To define database models in SQLAlchemy, you utilize Python classes to represent database tables, where each class corresponds to a table in the database schema. SQLAlchemy makes it easy to create and manage these models through its declarative system, which allows you to set up classes that automatically link to database tables.
When defining a model, several important aspects should be considered:
- Each class must specify a name for the corresponding table using the
__tablename__
attribute. - You define the columns of the table as class attributes, using instances of the
Column
class, where you specify the data types and constraints such as primary keys and unique indexes. - You can define relationships between models to establish how they interact with each other.
- The models are linked to a metadata object that maintains information about the tables and their relationships.
Here is an example of defining a database model using SQLAlchemy for a simple application that involves users and their articles:
from sqlalchemy import create_engine, Column, Integer, String, ForeignKey from sqlalchemy.ext.declarative import declarative_base from sqlalchemy.orm import relationship Base = declarative_base() class User(Base): __tablename__ = 'users' id = Column(Integer, primary_key=True) name = Column(String) age = Column(Integer) # Relationship to Article articles = relationship('Article', back_populates='author') class Article(Base): __tablename__ = 'articles' id = Column(Integer, primary_key=True) title = Column(String) content = Column(String) user_id = Column(Integer, ForeignKey('users.id')) # Relationship to User author = relationship('User', back_populates='articles') # Create the database engine engine = create_engine('sqlite:///:memory:') Base.metadata.create_all(engine)
In this example:
- The
User
class andArticle
class are defined to represent theusers
andarticles
tables, respectively. - Each class specifies its own table name through the
__tablename__
attribute. - Columns are defined using the
Column
class, where we setInteger
,String
, and relationships usingForeignKey
. - A one-to-many relationship is established between
User
andArticle
using therelationship
function. This means that one user can have multiple articles, while each article is associated with one user. - Finally, the
Base.metadata.create_all(engine)
function creates the tables in the database based on the defined models.
Defining models in SQLAlchemy allows for a clear representation of the database schema while providing the functionality to handle complex queries and relationships within the database. By adhering to the conventions of SQLAlchemy, developers can easily manage their database interactions through the ORM capabilities effectively.
Performing CRUD Operations
Performing CRUD (Create, Read, Update, Delete) operations is fundamental when working with databases and SQLAlchemy ORM is designed to simplify these tasks. Each of these operations corresponds to actions that can be easily executed with the ORM’s session management capabilities. Let’s dive into how you can perform these operations using SQLAlchemy.
Before performing CRUD operations, you need to establish a session, which serves as the workspace for all interactions with your database. Here’s how to create a session:
from sqlalchemy.orm import sessionmaker # Create a session factory Session = sessionmaker(bind=engine) # Create a new session session = Session()
With the session set up, we can proceed to the CRUD operations:
Create: To add new entries to your database, you can create an instance of your model and add it to the session. After that, calling session.commit()
will save the changes to the database.
# Creating a new user new_user = User(name="Alice", age=30) session.add(new_user) session.commit()
This snippet creates a new User object and adds it to the session. When session.commit()
is called, the new user is saved to the database.
Read: To read or fetch data from the database, you can use the session’s query capabilities. Here are a couple of ways to perform read operations:
# Fetching all users users = session.query(User).all() # Fetching a specific user by id specific_user = session.query(User).filter_by(id=1).first()
In the above examples, session.query(User).all()
retrieves all user records, while filter_by(id=1).first()
fetches a user with an id of 1.
Update: Updating existing entries involves first querying the object you wish to update, modifying its attributes, and then committing the session.
# Updating a user's age user_to_update = session.query(User).filter_by(name="Alice").first() if user_to_update: user_to_update.age = 31 session.commit()
Here, we find the user named “Alice” and update her age to 31. After modifying the record, session.commit()
saves the changes.
Delete: Deleting entities is also simpler. You first want to find the object, and then call session.delete()
.
# Deleting a user user_to_delete = session.query(User).filter_by(name="Alice").first() if user_to_delete: session.delete(user_to_delete) session.commit()
In this example, if a user named “Alice” is found, we delete her from the database and commit the change.
It is important to note that after performing these CRUD operations, you should always close the session when done:
session.close()
By using SQLAlchemy’s session capabilities for making CRUD operations easy and intuitive, developers can efficiently manage their database entries through the ORM without needing detailed knowledge of SQL syntax.
Querying the Database
from sqlalchemy.orm import sessionmaker # Assuming the engine is already created and models are defined Session = sessionmaker(bind=engine) session = Session() # Querying the Database # Querying all users all_users = session.query(User).all() print("All users:", all_users) # Filtering users by age filtered_users = session.query(User).filter(User.age > 25).all() print("Users older than 25:", filtered_users) # Fetching a specific user with a specific id specific_user = session.query(User).filter_by(id=1).first() print("User with ID 1:", specific_user) # Using the `in_` method to filter multiple criteria specific_names = session.query(User).filter(User.name.in_(["Alice", "Bob"])).all() print("Users named Alice or Bob:", specific_names) # Ordering results ordered_users = session.query(User).order_by(User.age.desc()).all() print("Users ordered by age (descending):", ordered_users) # Limiting results limited_users = session.query(User).limit(2).all() print("First two users:", limited_users) # Joining tables to fetch related data articles_with_authors = session.query(Article).join(User).all() for article in articles_with_authors: print(f"Article Title: {article.title}, Author: {article.author.name}") # Closing the session session.close()
Querying the database with SQLAlchemy is powerful and flexible, which will allow you to retrieve data in various ways depending on your requirements. Below are some of the key techniques for querying the database using SQLAlchemy ORM:
- You can fetch all records from a table by using the
all()
method, as shown in the examples where we retrieve all users. - SQLAlchemy allows filtering results using conditions. This can be done using methods like
filter()
orfilter_by()
. For instance, you can filter users based on age or name, as demonstrated above. - SQLAlchemy supports various operators that can be utilized in filtering, such as
==
,!=
,>
,<
, and more for comparisons. - Instead of fetching entire objects, you can select specific columns by passing those columns to the
with_entities()
method. - You can arrange the order of the returned results using the
order_by()
method, which allows sorting by any column (ascending or descending). - To restrict the number of results returned, the
limit()
method can be employed placing a cap on the result set. That’s useful for pagination applications. - SQLAlchemy supports joining tables seamlessly. This functionality allows you to fetch related entities across multiple tables, as seen with the articles and their authors.
Managing Relationships Between Models
“`html
When working with databases, managing relationships between models very important for representing complex data interactions effectively. SQLAlchemy ORM simplifies this process by allowing developers to define relationships between their models in an intuitive manner. Understanding how to manage these relationships enables developers to create more sophisticated and interconnected data models.
There are several types of relationships you can define in SQLAlchemy, including:
- A single record in one table relates to multiple records in another table. This is commonly seen in scenarios like users with multiple orders.
- Multiple records in one table relate to a single record in another table. That is the inverse of the one-to-many relationship.
- Records in one table relate to multiple records in another table, and vice versa. That’s often implemented using an association table to facilitate the relationship.
Let’s take a closer look at how to implement each of these relationship types within SQLAlchemy models.
### One-to-Many Relationship Example
In this example, we will expand on the previous models of users and articles. Each user can have multiple articles, which is represented as a one-to-many relationship.
from sqlalchemy import create_engine, Column, Integer, String, ForeignKey from sqlalchemy.ext.declarative import declarative_base from sqlalchemy.orm import relationship, sessionmaker Base = declarative_base() class User(Base): __tablename__ = 'users' id = Column(Integer, primary_key=True) name = Column(String) age = Column(Integer) # One-to-many relationship with Articles articles = relationship('Article', back_populates='author') class Article(Base): __tablename__ = 'articles' id = Column(Integer, primary_key=True) title = Column(String) content = Column(String) user_id = Column(Integer, ForeignKey('users.id')) # Many-to-one relationship with User author = relationship('User', back_populates='articles') engine = create_engine('sqlite:///:memory:') Base.metadata.create_all(engine)
In this code snippet, the User
class has a relationship with the Article
class where one user can have many articles. The relationship
function is used to specify that the author
attribute in the Article
class points back to the User
.
### Many-to-One Relationship Example
In the previous example, the many-to-one relationship is already set up with the user_id
in the Article
model, which references the primary key of the User
model.
### Many-to-Many Relationship Example
Many-to-many relationships can be established using an association table. This table will include foreign keys pointing to the primary keys of both related tables.
class User(Base): __tablename__ = 'users' id = Column(Integer, primary_key=True) name = Column(String) articles = relationship('Association', back_populates='user') # Association Table association_table = Base.metadata.tables['association'] = Table('association', Base.metadata, Column('user_id', ForeignKey('users.id')), Column('article_id', ForeignKey('articles.id')) ) class Article(Base): __tablename__ = 'articles' id = Column(Integer, primary_key=True) title = Column(String) content = Column(String) users = relationship('Association', back_populates='article') class Association(Base): __tablename__ = 'association' user_id = Column(Integer, ForeignKey('users.id'), primary_key=True) article_id = Column(Integer, ForeignKey('articles.id'), primary_key=True) Base.metadata.create_all(engine)
In the above example, we establish a many-to-many relationship between users and articles through an association table called association
. Each record in this table links a user to an article, enabling flexibility in how these relationships are queried.
### Using Relationships in Queries
Once relationships are defined, you can easily query them. For example:
# Create a new user and articles session = sessionmaker(bind=engine)() new_user = User(name="Bob", age=25) new_article_1 = Article(title="First Article", content="Content of the first article.") new_article_2 = Article(title="Second Article", content="Content of the second article.") # Associate articles with the user new_user.articles.append(new_article_1) new_user.articles.append(new_article_2) session.add(new_user) session.commit() # Query the user and their articles user_with_articles = session.query(User).filter(User.name == "Bob").first() print(f"User: {user_with_articles.name}") for article in user_with_articles.articles: print(f"Article: {article.title}") # Close the session session.close()
In this code snippet, we create a new user and associate multiple articles with that user. When querying the user, we can easily access all articles linked to him through the established relationship.
Managing relationships between models in SQLAlchemy ORM is a powerful feature that simplifies data handling. It allows developers to create complex data structures while keeping the code understandable and manageable. With clear relationships defined, your application can efficiently model real-world scenarios and perform extensive data querying with ease.
“`
Source: https://www.pythonlore.com/getting-started-with-sqlalchemy-orm/