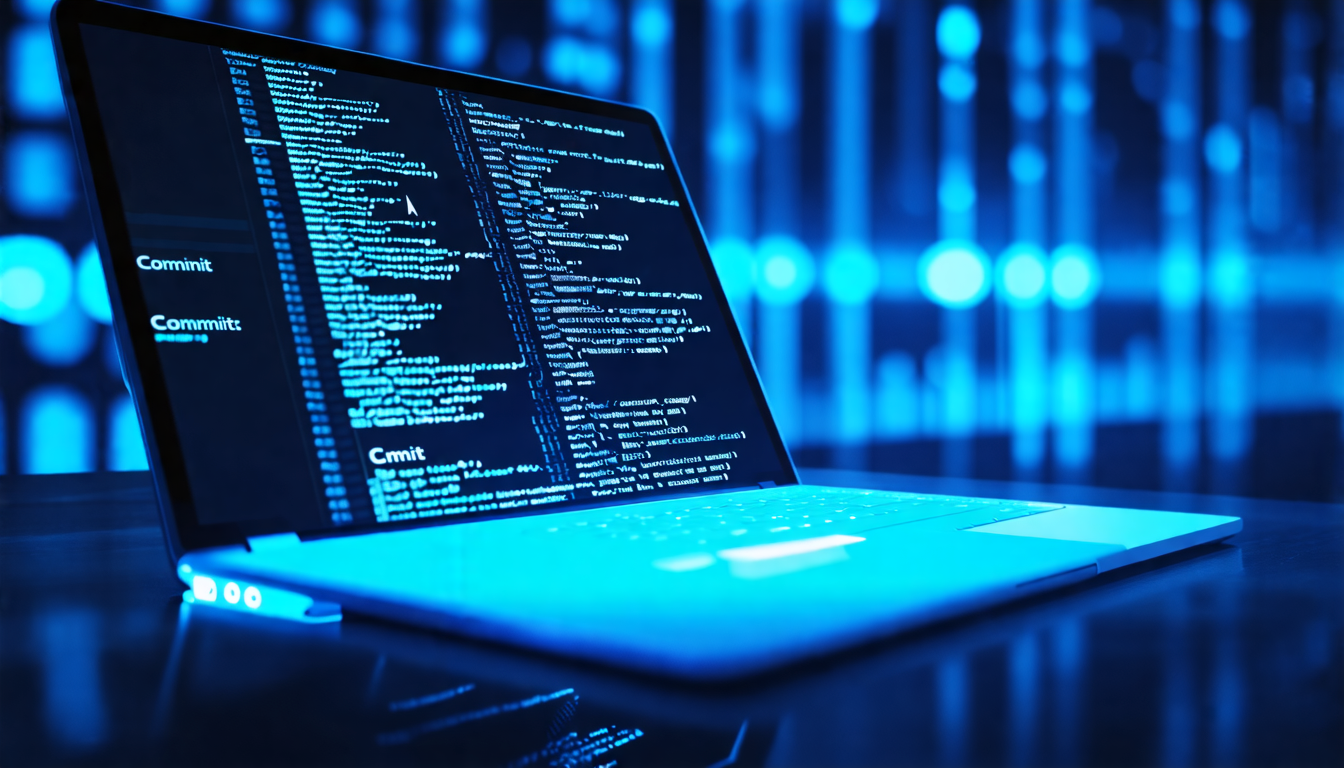
Transactions are a fundamental concept in database management systems, including SQLite3. They ensure that a series of operations within a database are executed in a reliable and consistent manner. A transaction is essentially a sequence of one or more database operations that are treated as a single unit of work. The primary aim of transactions is to maintain the integrity of the database, particularly in environments where multiple operations may be happening at the same time.
In SQLite3, transactions are governed by the properties defined by the acronym ACID, which stands for:
- This property ensures that all operations within a transaction are completed successfully; if any operation fails, the entire transaction is rolled back, leaving the database unchanged.
- Transactions must take the database from one valid state to another, preserving the integrity constraints defined for the database.
- Transactions should operate independently of one another. Even if multiple transactions are executing concurrently, the result should be the same as if they were executed serially.
- Once a transaction has been committed, it remains permanent, even in the event of a system crash or failure.
To demonstrate how transactions work, here’s how you can utilize the transaction commands in SQLite3 using Python. Open a connection to your SQLite3 database, begin a transaction, perform some database operations, and either commit or rollback based on success or failure.
import sqlite3 # Connect to the SQLite database connection = sqlite3.connect('example.db') try: # Start a transaction connection.execute('BEGIN TRANSACTION') # Perform some database operations connection.execute("INSERT INTO users (name, age) VALUES ('Alice', 30)") connection.execute("INSERT INTO users (name, age) VALUES ('Bob', 25)") # Commit the transaction if all operations succeed connection.commit() except Exception as e: # Rollback the transaction if an error occurs connection.rollback() print(f"Transaction failed: {e}") finally: # Close the database connection connection.close()
In this example, the transaction begins with the BEGIN TRANSACTION
command. Multiple insert operations are then executed. If all insertions are successful, the transaction is committed using connection.commit()
. However, if an error occurs, the connection.rollback()
method is called, ensuring that none of the changes are applied to the database.
Understanding and using transactions correctly is vital for robust database operations, allowing developers to handle errors gracefully and maintain data integrity in their applications.
The Role of Commit in Transaction Management
The commit operation in SQLite3 very important for ensuring that the changes made during a transaction become permanent in the database. When you call the commit()
method, you’re telling SQLite3 that you’re satisfied with all the operations that have been executed since the last BEGIN TRANSACTION
. At this point, SQLite3 will write all changes to the database and make them visible to other transactions.
Here are some key points about the role of commit in transaction management:
- The commit command finalizes all changes made during the transaction. Until a commit is executed, the changes are only temporarily held in memory.
- Once a commit is issued, all changes made in the transaction become immediately visible to other transactions. This means that other processes can now read the updated data.
- If an error occurs during the transaction, you have the option to rollback instead of committing. Rolling back reverts the database to its state before the transaction started, ensuring no partial changes are made.
- Frequent commits can lead to performance overhead, especially in high-volume transactions. It’s important to balance between committing often enough to ensure data safety and not too often to incur excess performance costs.
Here’s a further example of using the commit operation in a more complex scenario where multiple operations are performed:
import sqlite3 # Function to add users in bulk to the database def add_users(user_list): connection = sqlite3.connect('example.db') try: connection.execute('BEGIN TRANSACTION') for user in user_list: connection.execute("INSERT INTO users (name, age) VALUES (?, ?)", (user['name'], user['age'])) # Commit all the changes after successful insertion connection.commit() print("All users added successfully.") except Exception as e: # Rollback if any error occurs connection.rollback() print(f"Transaction failed: {e}") finally: connection.close() # Sample user data users_to_add = [{'name': 'Charlie', 'age': 28}, {'name': 'Diana', 'age': 34}] add_users(users_to_add)
In this example, a function add_users is defined to bulk insert users into the database. The commit()
call ensures that all the insert statements are executed successfully before making them permanent. If any error occurs during the execution of the insert statements, the entire transaction is rolled back, preventing any modifications from being applied.
Understanding the commit function is essential for effective transaction management in SQLite3. By ensuring that changes are only made permanent when intended, developers can maintain the integrity and consistency of their databases while handling potential errors gracefully.
Implementing Rollback for Error Handling
When working with databases, error handling is an important aspect of data integrity and reliability. In SQLite3, implementing rollback functionality allows developers to revert any changes made during the transaction if an error occurs. This ensures that the database remains in a consistent state, and no partial or erroneous data is stored. Below is a detailed explanation of how to implement rollback for error handling in SQLite3 transactions.
The rollback operation is triggered whenever an exception is raised during the transaction. This operation undoes all the changes made during the transaction since it started, restoring the database to its previous state. The rollback is particularly important in scenarios where multiple database operations are performed in a single transaction, as it prevents inconsistent data from being committed.
Here is a practical example of implementing rollback for error handling:
import sqlite3 # Function to update user age in the database def update_user_age(user_id, new_age): connection = sqlite3.connect('example.db') try: # Start a transaction connection.execute('BEGIN TRANSACTION') # Attempt to update user's age connection.execute("UPDATE users SET age = ? WHERE id = ?", (new_age, user_id)) # Simulate an error for demonstration purposes if new_age 0: raise ValueError("Age cannot be negative") # Commit the transaction if successful connection.commit() print("User age updated successfully.") except Exception as e: # Rollback the transaction if an error occurs connection.rollback() print(f"Transaction failed: {e}") finally: connection.close() # Update user with ID 1 to a new age update_user_age(1, -5) # This will trigger a rollback
In this example, the update_user_age
function attempts to update a user’s age in the database. A transaction starts with the command connection.execute('BEGIN TRANSACTION')
. If the provided age is negative, a ValueError
is raised, simulating a logical error in the business rules. Upon catching this exception, the connection.rollback()
method is invoked to revert any changes made during the transaction, ensuring that the database remains unchanged.
Implementing rollback effectively allows developers to manage errors gracefully. Here are a few tips to keep in mind when implementing rollback for error handling in SQLite3:
- Use specific exceptions to catch known errors and handle them accordingly, so that you can implement custom rollback logic when required.
- Limit the number of operations within a transaction. The longer a transaction holds locks, the more likely contention will occur, which can lead to errors.
- Thoroughly test your error handling logic to ensure that rollback behaves as expected under various conditions, especially when the application logic is complex.
- Implement logging within the exception handling block to aid in diagnosing errors during transaction processing.
By effectively using rollback, developers can ensure that their applications handle errors seamlessly, promoting data integrity and user confidence in the reliability of the system.
Best Practices for Using Commit and Rollback
When working with commit and rollback in SQLite3, adhering to best practices is essential for maintaining data integrity and achieving optimal performance. Here are some key strategies that can help you effectively use commit and rollback in your database operations:
- Always use transactions when multiple related changes are being made to ensure that either all or none of the changes are applied. That’s particularly important in operations that involve updates to multiple records or tables.
- Keep transactions as short as possible. This minimizes the time locks are held on the database, reducing potential contention and the likelihood of deadlocks. Performing quick operations within a transaction allows for better concurrency.
- Always implement exception handling to catch any errors that occur during transactions. The rollback operation should be called in the event of an error to revert the database to its previous state, ensuring data consistency.
- SQLite3 does not support true nested transactions, even though it allows for savepoints. Using them can lead to complexity and confusion. It’s best to handle transactions at a single level and manage multiple operations within that context.
- Thoroughly test your transaction logic, especially edge cases and failure scenarios. Make sure that your commit and rollback operations work as intended, and that the database accurately reflects the expected state after transactions.
- Regularly monitor your application’s performance and evaluate the impact of your transaction management. Look for opportunities to optimize and reduce the overhead associated with committing or rolling back transactions.
Here’s an example illustrating how to implement these best practices in a user registration scenario:
import sqlite3 def register_user(username, age): connection = sqlite3.connect('example.db') try: connection.execute('BEGIN TRANSACTION') # Check if username already exists existing_user = connection.execute("SELECT * FROM users WHERE name = ?", (username,)).fetchone() if existing_user: raise ValueError("Username already exists.") # Insert new user connection.execute("INSERT INTO users (name, age) VALUES (?, ?)", (username, age)) # If everything is fine, commit connection.commit() print(f"User {username} registered successfully.") except Exception as e: connection.rollback() print(f"Registration failed: {e}") finally: connection.close() # Example usage register_user('Eve', 29) # Should succeed register_user('Eve', 25) # Should fail and trigger rollback
In this example, the `register_user` function demonstrates several best practices:
- It begins with a transaction and checks for existing records to prevent duplicate usernames.
- Exceptions are handled gracefully to roll back any changes if an error occurs during registration.
- The connection is properly closed in the finally block to prevent resource leaks.
By following these best practices for commit and rollback operations in SQLite3, you can enhance your application’s reliability, maintain data integrity, and improve overall user experience.
Real-world Examples of Transaction Management in SQLite3
import sqlite3 # Function to transfer funds between accounts def transfer_funds(source_account, target_account, amount): connection = sqlite3.connect('bank.db') try: connection.execute('BEGIN TRANSACTION') # Deduct amount from source account connection.execute("UPDATE accounts SET balance = balance - ? WHERE account_id = ?", (amount, source_account)) # Simulate an error if there are insufficient funds current_balance = connection.execute("SELECT balance FROM accounts WHERE account_id = ?", (source_account,)).fetchone()[0] if current_balance 0: raise ValueError("Insufficient funds for transfer.") # Add amount to target account connection.execute("UPDATE accounts SET balance = balance + ? WHERE account_id = ?", (amount, target_account)) # Commit changes if both operations succeed connection.commit() print("Transfer completed successfully.") except Exception as e: # Rollback in case of error connection.rollback() print(f"Transfer failed: {e}") finally: connection.close() # Example transfer transfer_funds('acc123', 'acc456', 100) # This will either succeed or fail
In real-world applications, transaction management especially important. For example, think a banking system where funds need to be transferred between accounts. The transaction involves two main operations: deducting funds from the source account and adding them to the target account. Both operations must either succeed or fail together to ensure the consistency of the financial data.
In the provided function `transfer_funds`, a transaction is initiated and two updates are executed within it. If there’s an attempt to transfer more than the available balance in the source account, the code raises an exception to signify an error. The rollback function ensures that no part of the transaction is applied if an error occurs, maintaining the integrity of the database.
Another common scenario could be handling user orders in an e-commerce application:
import sqlite3 # Function to process an order def process_order(order_id): connection = sqlite3.connect('ecommerce.db') try: connection.execute('BEGIN TRANSACTION') # Get order details order_details = connection.execute("SELECT * FROM orders WHERE order_id = ?", (order_id,)).fetchone() # Check inventory for product availability product_id = order_details[1] # Assuming product_id is the second column quantity = order_details[2] # Assuming quantity is the third column available_stock = connection.execute("SELECT stock FROM products WHERE product_id = ?", (product_id,)).fetchone()[0] if available_stock quantity: raise ValueError("Insufficient stock to fulfill the order.") # Deduct stock from inventory connection.execute("UPDATE products SET stock = stock - ? WHERE product_id = ?", (quantity, product_id)) # Mark order as processed connection.execute("UPDATE orders SET status = 'processed' WHERE order_id = ?", (order_id,)) connection.commit() print("Order processed successfully.") except Exception as e: connection.rollback() print(f"Order processing failed: {e}") finally: connection.close() # Process an order process_order('ord789') # This may either succeed or fail based on stock availability
In this scenario, the `process_order` function initiates a transaction when processing an order. It checks the availability of stock for the product involved in the order. If there isn’t enough stock, an exception is raised, triggering a rollback that ensures no modifications are saved to the database. If both updates succeed, the transaction is committed, ensuring that both the stock is adjusted and the order status is updated simultaneously.
These examples highlight the importance of transaction management in scenarios where multiple database operations are interdependent. By employing transactions effectively, developers can maintain integrity and consistency within their applications, ensuring dependable user experiences.
Source: https://www.pythonlore.com/using-sqlite3-commit-and-rollback-for-transaction-management/