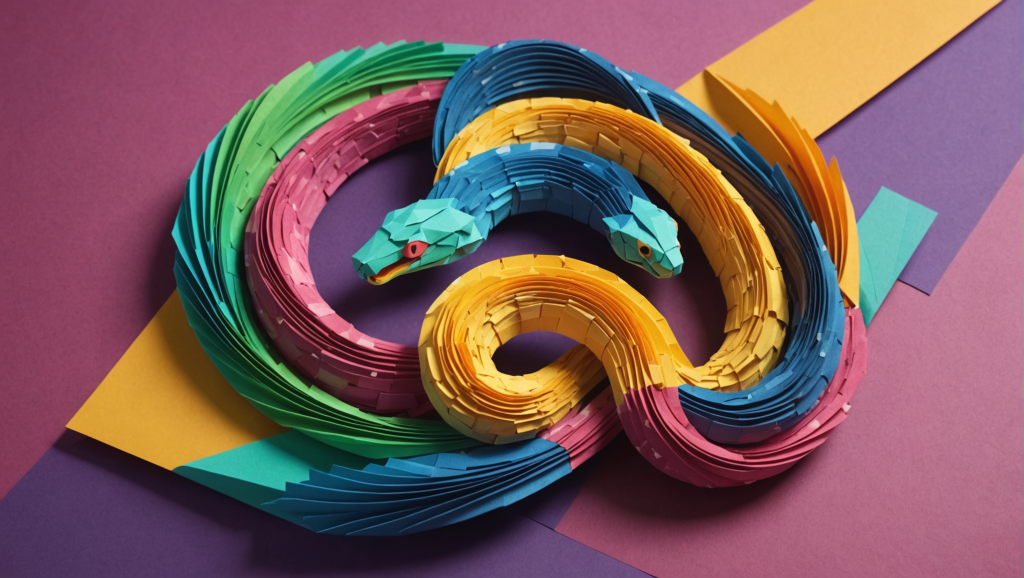
The re.Match
object is a cornerstone of the Python regular expression module, encapsulating the results of a search operation performed by the re
library. At its essence, the re.Match
object serves as a bridge between the intricacies of your regular expression and the text being analyzed. That is where computational finesse meets the elegance of text manipulation.
To comprehend the structure of a re.Match
object, consider its principal attributes. The most fundamental of these is the string. This attribute retains the original string that was subject to the search. Additionally, the start and end methods yield the indices of the match’s beginning and end positions, respectively. Notably, the result of the match itself can be accessed via the group method, which retrieves the specific substring that was matched.
Here is a concise illustration demonstrating the structure of a re.Match
object:
import re # Sample text and pattern text = "The quick brown fox jumps over the lazy dog" pattern = r"brown" # Searching the pattern in the text match = re.search(pattern, text) # Analyzing the re.Match object if match: print("Original String:", match.string) # The original string print("Matched String:", match.group()) # The matched string print("Match starts at index:", match.start()) # Start index print("Match ends at index:", match.end()) # End index else: print("No match found.")
Upon executing the above code, one is greeted with informative output that showcases the capabilities of the re.Match
object. In this case, the substring “brown” is extracted from the original string, while the indices provide context for its location within that string. Thus, the re.Match
object not only encapsulates information about what was matched, but also provides means to navigate and manipulate the surrounding text.
The re.Match
object is a veritable treasure trove for any programmer keen on using the power of regular expressions in Python. Armed with this understanding, one can deftly navigate through strings, extracting and using data with the precision of a master craftsman.
Creating Match Objects with Regular Expressions
In this section, we shall delve into the creation of match objects using Python’s regular expressions. Regular expressions provide a powerful means to identify patterns within text, and the re.Match object is created as a result of this pattern matching process. The method we typically employ to initiate a match operation is either re.search()
, re.match()
, or re.findall()
. Each has its nuances, yet they all lead to the generation of a re.Match object if a corresponding match is found.
Let’s consider the re.search()
method in greater detail. This method scans through the entire string looking for the first location where the regular expression pattern produces a match. If a match is found, it returns a re.Match object; if not, it returns None
. The beauty of re.search()
lies in its flexibility, as it does not require the pattern to match from the start of the string.
import re # Sample text and pattern text = "The rain in Spain stays mainly in the plain." pattern = r"ain" # Searching the pattern in the text match = re.search(pattern, text) # Check if a match was found if match: print("Matched String:", match.group()) # The matched string print("Match starts at index:", match.start()) # Start index print("Match ends at index:", match.end()) # End index else: print("No match found.")
In the above example, the search for the substring “ain” successfully yields a re.Match object, effectively demonstrating its capabilities. The start and end indices allow a programmer to locate the match precisely within the original string.
Next, consider the re.match()
method, which is somewhat more stringent than re.search()
. It only looks for a match at the beginning of the string. If the pattern does not occur at the start, re.match()
will yield None
without further ado.
import re # Sample text starting with the pattern text = "Hello, world!" pattern = r"Hello" # Matching the pattern at the start of the text match = re.match(pattern, text) if match: print("Matched String:", match.group()) # The matched string print("Match starts at index:", match.start()) # Start index print("Match ends at index:", match.end()) # End index else: print("No match found.")
Here, the match method is aptly suited for our requirement, capturing the greeting “Hello” as it resides comfortably at the start of the string. The resulting re.Match object once again provides insights into where the match occurs.
Finally, we turn our attention to the re.findall()
method. Unlike its counterparts, re.findall()
does not return a re.Match object. Instead, it returns a list of all non-overlapping matches of the pattern in the string. This can be exceedingly useful when multiple instances of a substring are present, allowing the programmer to retrieve them all simultaneously.
import re # Sample text with multiple occurrences of the pattern text = "The rain in Spain stays mainly in the plain." pattern = r"ain" # Finding all occurrences of the pattern in the text matches = re.findall(pattern, text) # Displaying the results print("All matches found:", matches)
The execution of this code snippet reveals a list containing all instances of “ain” found within the given text, highlighting the utility of re.findall() for comprehensive pattern recognition.
Thus far, we have examined the various methodologies for creating match objects in Python’s re module. Each method serves distinct purposes, enabling the programmer to select the most fitting approach based on the specific needs of their text processing task. Through these primal operations, the re.Match object emerges, ready to unlock the secrets embedded within strings.
Common Methods and Properties of Match Objects
The re.Match object encapsulates several methods and properties that enrich the experience of pattern matching in Python. Understanding these tools is akin to mastering the fundamental operations of a sophisticated machine; each method and property serves a specific purpose, enhancing our ability to manipulate and analyze strings efficiently.
Among the most prominent attributes of the re.Match object is the group() method. This method is instrumental when it comes to obtaining the matched substring. If the regular expression contains capturing groups, group() can also retrieve specific matched groups by passing an index. For instance, match.group(0) refers to the entire match, while match.group(1) refers to the first capturing group, and so forth.
import re # Sample text with capturing groups text = "Hello, my name is Alice." pattern = r"my name is (w+)" # Searching for the pattern match = re.search(pattern, text) # Displaying the full match and the captured group if match: print("Full Match:", match.group(0)) # Entire match print("Captured Name:", match.group(1)) # The name "Alice" else: print("No match found.")
In this scenario, the entire match is the phrase “my name is Alice,” while the group(1) call successfully extracts “Alice” from the context. This capability highlights the power of the re.Match object in fetching both specific and general match details.
In addition to the group() method, we also have access to start() and end() methods, which provide the indices marking the beginning and end of the matched substring within the original string. These methods are particularly useful when the precise location of the match is a requirement, allowing us to carry out subsequent manipulations or analyses based on the match’s position.
import re # Example text and pattern text = "Python is a powerful programming language." pattern = r"powerful" # Searching for the pattern match = re.search(pattern, text) # Displaying the start and end indices if match: print("Start Index:", match.start()) # Start index of "powerful" print("End Index:", match.end()) # End index of "powerful" else: print("No match found.")
The above code provides the indices of where “powerful” resides in the original context, showcasing the utility of the re.Match object in terms of text navigation.
Moreover, the span() method can be employed to retrieve a tuple containing both the start and end positions of the match in a single call. This method offers a concise way to access the match indices, streamlining our operations.
import re # Sample text and pattern text = "Regular expressions are helpful for pattern matching." pattern = r"helpful" # Searching for the pattern match = re.search(pattern, text) # Displaying the start and end indices using span() if match: print("Match Span:", match.span()) # Tuple with (start, end) else: print("No match found.")
Lastly, the re.Match object also possesses the lastindex property, which can be used to ascertain the index of the last captured group in a match. That is particularly advantageous when working with complex patterns containing multiple capturing groups, providing insight into which group was the last one matched.
import re # Text with multiple capturing groups text = "The car is red and the bike is blue." pattern = r"(?Pcar|bike) is (?Pred|blue)" # Searching for the pattern match = re.search(pattern, text) # Displaying the last index captured if match: print("The last group captured is:", match.lastindex) # Should show 2 for color else: print("No match found.")
The re.Match object offers a robust collection of methods and properties that allow programmers to extract, navigate, and manipulate matched substrings with precision. By becoming intimately acquainted with these tools, one can wield regular expressions like a seasoned artisan, crafting elegant solutions to complex text-processing challenges.
Practical Examples of Using Match Objects
The practical applications of the re.Match object in Python are manifold, enabling programmers to engage with text in a nuanced and sophisticated manner. To illustrate these possibilities, consider the following practical examples that illustrate how to harness the power of match objects effectively.
First, let us explore how to validate user input, a common task in many Python applications. Suppose we wish to ensure that a user-provided email address conforms to standard formatting. By employing a regular expression, we can extract the relevant components of the email address and confirm its validity.
import re # Sample email address email = "[email protected]" # Regular expression for validating an email address pattern = r"(^[a-zA-Z0-9_.+-]+@[a-zA-Z0-9-]+.[a-zA-Z0-9-.]+$)" # Searching for the pattern in the email address match = re.search(pattern, email) if match: print("Valid email address:", match.group()) else: print("Invalid email address.")
In this example, if the input email is valid, the re.Match object captures the entire email address, allowing us to confirm its correctness. The output will indicate whether the email address entered by the user conforms to the expected format.
Next, ponder a scenario where you need to extract all date occurrences from a text block. By crafting an appropriate regular expression and using the re.findall() method, we can leverage the power of the re.Match object to retrieve multiple occurrences efficiently:
import re # Sample text containing dates text = "The events were held on 2020-01-20, 2021-05-15, and 2022-12-31." # Regular expression to match date format YYYY-MM-DD pattern = r"(d{4}-d{2}-d{2})" # Finding all date occurrences in the text matches = re.findall(pattern, text) print("Extracted dates:", matches)
The above code employs re.findall() to extract all dates matching the YYYY-MM-DD format from a given string. These matches are stored in a list, demonstrating how the re.Match object can facilitate data extraction in bulk.
Moreover, if you need to iterate over multiple matches and perform different actions based on the match results, you can use the re.finditer() method. This method returns an iterator yielding match objects for each match found, allowing for detailed manipulation:
import re # Sample text containing multiple patterns text = "The quick brown fox jumps over the lazy dog. The quick brown dog jumps over the lazy fox." # Pattern to match the word "quick" and return its span pattern = r"quick" # Using finditer to iterate over matches for match in re.finditer(pattern, text): print(f"Found '{match.group()}' at indices: {match.span()}")
By using re.finditer(), each match object generated allows us to access both the matched string and its indices, providing valuable context as we interact with our text.
In yet another practical example, you might find yourself needing to implement text substitution based on pattern matches. The re.sub() method allows for identifying matches and replacing them effectively:
import re # Sample text text = "The rain in Spain stays mainly in the plain." # Regular expression to match "ain" and replace with "***" pattern = r"ain" # Substituting the matched pattern new_text = re.sub(pattern, "***", text) print("Modified text:", new_text)
This code snippet replaces all occurrences of “ain” with “***” in the original string, showcasing the versatility of regular expressions in transforming text as per requirements.
Throughout these examples, the power and flexibility of the re.Match object become evident, illustrating its capacity to assist in various text manipulation tasks. By mastering these practical applications, one cultivates the ability to wield regular expressions as an invaluable tool for sophisticated text processing within Python.
Troubleshooting Common Issues with Match Objects
When delving into the world of re.Match objects, one must inevitably confront the challenges that arise during pattern matching. Troubleshooting common issues with match objects is an essential skill for any Python programmer, as it not only clarifies the intricacies of regular expressions but also enhances one’s problem-solving acumen.
One prevalent issue stems from the misunderstanding of the behavior of the search methods. For example, using re.search() may yield None when one expects a match, often due to subtle discrepancies in the regular expression or the target string. It is prudent to check whether the pattern and the string are in the expected format. A frequent pitfall is the failure to escape special characters in the regex pattern, which can lead to unexpected results.
Think the following scenario where a programmer attempts to match a literal period in a text:
import re # Sample text with a period text = "I love programming in Python." # Pattern to match a period pattern = r"." # Searching for the pattern match = re.search(pattern, text) if match: print("Matched String:", match.group()) else: print("No match found.") # This will print "matched string" unexpectedly
Here, the intention may have been to find a literal period; however, the pattern r”.” matches any character due to the dot’s special role in regex. To accurately match the period, one should escape it:
pattern = r"." # Escaped period match = re.search(pattern, text)
Subsequently, one can find the desired match, thereby uncovering the importance of escaping special characters within regular expressions.
Another common issue involves mismatched expectations regarding the output of re.findall() versus re.search(). While re.search() returns a single match object (or None), re.findall() returns a list of all occurrences, leading to potential confusion:
text = "There are two cats and three dogs." pattern = r"cats" # Using re.findall matches = re.findall(pattern, text) if matches: print("Matches found:", matches) # This outputs a list, ["cats"] else: print("No match found.") # This will not print
Should one use re.search() with the same pattern, it would return a match object rather than a list. Therefore, understanding the outputs of these methods is important when debugging, as mismatches can lead to logical errors in one’s code.
Additionally, programmers may encounter issues related to the indexing of groups within the match object. It’s essential to remember that group indices begin at 0 for the entire match and increment for each capturing group. A misinterpretation of indices can result in None being returned when attempting to access non-existent groups.
text = "The number is 42." pattern = r"(d+)" # A capturing group for digits match = re.search(pattern, text) if match: print("Full match:", match.group(0)) # Match for the whole print("First group:", match.group(1)) # Correct group index print("Second group:", match.group(2)) # This will raise an IndexError!
To mitigate such issues, it is advisable to first assess the number of capturing groups in the pattern prior to accessing them, thus ensuring robust code that anticipates possible indexing errors.
Lastly, failure to recognize case sensitivity in regex patterns could lead a programmer to erroneously conclude that a valid match does not exist. In Python, pattern matching is case-sensitive by default. To overcome this obstacle, the re.IGNORECASE flag can be employed to ignore case distinctions:
pattern = r"python" match = re.search(pattern, text, re.IGNORECASE) # Case insensitive search
By effectively using this flag, programmers can expand their matching capabilities, ensuring that variations in casing do not prevent successful matches.
Through a systematic approach to troubleshooting, one can navigate the challenges associated with re.Match objects and regular expressions. By honing these skills, programmers will enhance their mastery over text processing in Python, transforming potential frustrations into fluidity and confidence in their coding endeavors.
Source: https://www.pythonlore.com/exploring-re-match-for-match-objects/