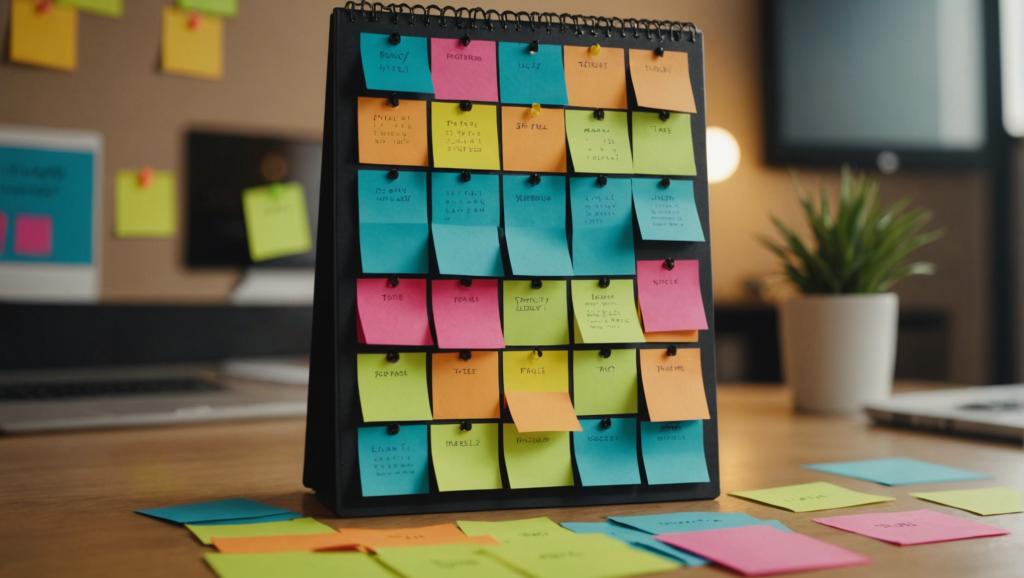
EventKit is a powerful framework provided by Apple that allows developers to work with users’ calendar and reminder data. By using this framework, applications can create, access, and modify calendar events and reminders seamlessly, enhancing the user experience through personalized scheduling features.
At its core, EventKit provides a structured way to interact with the calendar and reminders on iOS and macOS devices. It exposes a set of classes and methods that developers can use to retrieve, create, and modify events and reminders, all while respecting user privacy and data integrity.
One of the key benefits of using EventKit is its ability to sync with iCloud calendars and reminders, making it easier to manage events across multiple devices. When an application requests access to these resources, it especially important to ensure that the user understands why the access is needed, as this builds trust and encourages users to grant permissions.
In terms of architecture, the EventKit framework primarily revolves around two main classes: EKEventStore and EKEvent. The EKEventStore class is responsible for managing access to the calendar and reminders, while the EKEvent class represents a specific event in a calendar.
Understanding the relationships between these classes is essential for effective use of the framework. For instance, developers can utilize EKEventStore to fetch all existing events and reminders, and then manipulate EKEvent objects to create new events or modify existing ones.
Here’s a simple example of how to initialize an EKEventStore instance and request access to the calendar:
import EventKit let eventStore = EKEventStore() eventStore.requestAccess(to: .event) { (granted, error) in if granted { print("Access granted to the calendar.") } else { print("Access denied: (String(describing: error?.localizedDescription))") } }
This code snippet demonstrates how to create an instance of EKEventStore and asynchronously request access to the user’s calendar. Proper handling of permission requests is important, as failing to obtain access can result in a significant limitation on functionality.
In addition to access management, EventKit allows for rich querying capabilities. Developers can fetch events based on various criteria such as time frames, event types, and more. This flexibility enables applications to provide tailored experiences, displaying only relevant events to the user.
The EventKit framework serves as a comprehensive toolkit for managing calendar events and reminders within iOS and macOS applications. Its robust set of features empowers developers to create applications that seamlessly interact with users’ scheduling needs while maintaining a focus on privacy and usability.
Setting Up EventKit in Your Swift Project
To effectively set up EventKit in your Swift project, you’ll need to follow a few simpler steps to ensure that your application can interact with the calendar and reminders on the user’s device. The first step is to include the EventKit framework in your project, which allows you to access the necessary classes and methods for managing events and reminders.
Start by opening your Xcode project and navigating to the project settings. Under the “General” tab, find the “Frameworks, Libraries, and Embedded Content” section. Click the “+” button to add a new framework and search for “EventKit.” Select it and add it to your project. This inclusion is essential for the subsequent steps, as it grants your application the ability to utilize EventKit’s functionalities.
Next, it’s important to ensure that your app has the appropriate permissions to access the user’s calendar. That is managed through the app’s Info.plist file. You need to add a new entry for the usage description key that corresponds to calendar access: NSCalendarsUsageDescription
. This string should describe to users why your app requires access to their calendar data. Not only does this serve as a guide for users, but it also aligns with best practices for user privacy.
NSCalendarsUsageDescription This app needs access to your calendar to manage your events and reminders.
Once you’ve configured your project settings and Info.plist, you can begin integrating EventKit into your app’s logic. As illustrated in the previous section, the first step in using EventKit is to create an instance of the EKEventStore
class and request permission to access the calendar. The following example demonstrates how to encapsulate this request in a function, ensuring that the permission check is reusable throughout your application:
import EventKit func requestCalendarAccess(completion: @escaping (Bool) -> Void) { let eventStore = EKEventStore() eventStore.requestAccess(to: .event) { (granted, error) in if granted { print("Access granted to the calendar.") completion(true) } else { print("Access denied: (String(describing: error?.localizedDescription))") completion(false) } } }
In this function, you create an EKEventStore
instance and request access to the calendar events. The closure passed to requestAccess(to:)
provides a callback with the result of the request, allowing you to handle both scenarios: when access is granted and when it is denied.
Once you’ve set up the framework and handled permissions, you can start using EventKit to query and interact with calendar data. This setup is foundational for building applications that require calendar functionality, and proper configuration ensures a smooth user experience when accessing and managing calendar events.
Accessing Calendar Events and Reminders
To access calendar events and reminders using EventKit, you’ll primarily work with the EKEventStore class, which provides methods to fetch events and reminders from the user’s calendar. This process involves specifying search criteria such as date ranges, event types, and more, allowing you to tailor the data you retrieve based on the needs of your application.
When fetching calendar events, one of the most common tasks is to define a time range. By setting a start and end date, you can limit the events returned to a specific window. Here’s an example of how to fetch events occurring within a specified time frame:
import EventKit func fetchEvents(startDate: Date, endDate: Date) { let eventStore = EKEventStore() eventStore.requestAccess(to: .event) { (granted, error) in guard granted else { print("Access denied: (String(describing: error?.localizedDescription))") return } let predicate = eventStore.predicateForEvents(withStart: startDate, end: endDate, calendars: nil) let events = eventStore.events(matching: predicate) for event in events { print("Event Title: (event.title), Start Date: (event.startDate), End Date: (event.endDate)") } } }
In this example, the function fetchEvents
accepts a startDate
and endDate
to define the range for the event search. After confirming access, it constructs a predicate using predicateForEvents(withStart:end:calendars:)
to filter events. The resulting events are then iterated through, where each event’s title and start/end dates are printed.
Similarly, accessing reminders follows a comparable pattern, but you’ll utilize the EKReminder
class. Reminders can also be fetched using a predicate, allowing for efficient retrieval based on due dates or other attributes. Here’s how you might fetch reminders:
func fetchReminders() { let eventStore = EKEventStore() eventStore.requestAccess(to: .reminder) { (granted, error) in guard granted else { print("Access denied: (String(describing: error?.localizedDescription))") return } let predicate = eventStore.predicateForReminders(in: nil) eventStore.fetchReminders(matching: predicate) { reminders in for reminder in reminders ?? [] { print("Reminder Title: (reminder.title), Due Date: (String(describing: reminder.dueDateComponents))") } } } }
In this function, fetchReminders
, access to reminders is requested, and if granted, a predicate is created using predicateForReminders(in:)
. The reminders are then fetched with fetchReminders(matching:)
, so that you can handle them in a closure. This approach ensures that you only work with reminders the user has given consent to access, thereby respecting privacy while enabling functionality.
Overall, accessing calendar events and reminders using EventKit is streamlined and efficient, allowing developers to create personalized scheduling experiences in their apps. Using the power of predicates and the structured nature of EKEventStore, you can retrieve and display relevant data in a user-friendly manner.
Creating and Modifying Events Programmatically
Creating and modifying events programmatically with EventKit is a powerful feature that allows developers to imropve their applications with personalized scheduling capabilities. The EKEvent class provides a way to represent events, while EKEventStore manages the storage and retrieval of these event objects.
To create a new event, you’ll first need to instantiate an EKEvent object and populate it with the necessary details such as the title, start and end dates, and any additional properties like location and notes. Here’s a simpler example of how to create an event:
import EventKit func createEvent(title: String, startDate: Date, endDate: Date, location: String?, notes: String?) { let eventStore = EKEventStore() eventStore.requestAccess(to: .event) { (granted, error) in guard granted else { print("Access denied: (String(describing: error?.localizedDescription))") return } let event = EKEvent(eventStore: eventStore) event.title = title event.startDate = startDate event.endDate = endDate event.calendar = eventStore.defaultCalendarForNewEvents event.location = location event.notes = notes do { try eventStore.save(event, span: .thisEvent) print("Event created: (event.title)") } catch { print("Error saving event: (error.localizedDescription)") } } }
This function, createEvent, takes parameters for the event’s title, start and end dates, location, and notes. After requesting access to the calendar, it creates an EKEvent, populates it with the provided information, and saves it to the event store. It’s essential to handle potential errors when saving the event, as this can safeguard against issues such as write permissions or invalid data.
In addition to creating events, you may also need to modify existing events. This involves fetching the event you want to change, updating its properties, and then saving the changes back to the event store. Here’s an example of how to modify an event:
func modifyEvent(eventIdentifier: String, newTitle: String?, newStartDate: Date?, newEndDate: Date?, newLocation: String?, newNotes: String?) { let eventStore = EKEventStore() eventStore.requestAccess(to: .event) { (granted, error) in guard granted else { print("Access denied: (String(describing: error?.localizedDescription))") return } if let event = eventStore.event(withIdentifier: eventIdentifier) { if let newTitle = newTitle { event.title = newTitle } if let newStartDate = newStartDate { event.startDate = newStartDate } if let newEndDate = newEndDate { event.endDate = newEndDate } if let newLocation = newLocation { event.location = newLocation } if let newNotes = newNotes { event.notes = newNotes } do { try eventStore.save(event, span: .thisEvent) print("Event modified: (event.title)") } catch { print("Error modifying event: (error.localizedDescription)") } } else { print("Event not found.") } } }
The modifyEvent function takes an event identifier and new details for the event. It first requests access to the calendar, then fetches the event with the specified identifier. After updating the necessary properties, it saves the modified event back to the event store. Proper error handling is also critical here, particularly in cases where the event may not exist or access is denied.
By using these methods, developers can provide users with the ability to create and modify events directly from within their applications, making scheduling and organization more accessible and effortless to handle. The integration of EventKit into your app not only enhances functionality but also empowers users to manage their time effectively.
Handling Permissions for Calendar Access
Handling permissions for calendar access in an iOS application is a fundamental aspect that ensures user privacy while allowing your app to offer powerful scheduling capabilities. The EventKit framework requires explicit permission from the user before accessing their calendar and reminder data, which is a critical step in maintaining trust and transparency.
When your application first attempts to access calendar data, the system prompts the user with a dialog, providing them with the choice to grant or deny access. The wording of this prompt is crucial; it should clearly communicate why access is necessary and how it will enhance the user experience. That’s typically set in the Info.plist
file using the key NSCalendarsUsageDescription
. Here’s an example of how to define this key:
NSCalendarsUsageDescription This app requires access to your calendar to manage your events and reminders.
After configuring the Info.plist, your app can request access programmatically. It’s essential to check for permission status before attempting to access or manipulate calendar data. Here’s a function that elegantly handles this permission check:
import EventKit func checkCalendarAuthorization(completion: @escaping (Bool) -> Void) { let eventStore = EKEventStore() let status = EKEventStore.authorizationStatus(for: .event) switch status { case .authorized: completion(true) case .denied: print("Access denied to calendar.") completion(false) case .notDetermined: eventStore.requestAccess(to: .event) { (granted, error) in if granted { print("Access granted to calendar.") completion(true) } else { print("Access denied: (String(describing: error?.localizedDescription))") completion(false) } } case .restricted: print("Calendar access is restricted.") completion(false) default: completion(false) } }
This function, checkCalendarAuthorization
, first checks the current authorization status. If access has already been granted, it simply returns true. If the status is .notDetermined
, it initiates a request for access, handling both granted and denied outcomes appropriately. The cases for .denied
and .restricted
ensure that your application can react accordingly if permission issues arise.
Once permissions are granted, it’s crucial to remind users why the application needs this access. This can be achieved through UI prompts or educational pop-ups, particularly when users attempt to perform calendar-related actions for the first time. Building this context helps users feel more comfortable sharing their data.
Moreover, it’s essential to handle cases where permissions are denied gracefully. Ensure your application can still function without calendar access, perhaps by providing alternative features or notifying users about limited functionality. This approach not only respects user choices but also enhances the overall user experience.
Managing permissions effectively is a key part of working with the EventKit framework in Swift. By clearly communicating the purpose for accessing calendar data, implementing robust permission checks, and handling various outcomes appropriately, developers can create applications that respect user privacy while still offering powerful features for managing events and reminders.
Best Practices for Using EventKit in Swift
When working with EventKit in Swift, adhering to best practices is essential for creating an application this is both easy to use and respectful of user privacy. This framework, which handles the intricacies of interacting with calendar and reminder data, can be leveraged effectively by following a few key guidelines.
1. Respect User Privacy: Always ensure that your application requests permission to access the user’s calendar data before attempting to retrieve or modify any events or reminders. Be transparent about why you need this access. Craft clear and concise descriptions in your Info.plist, using the NSCalendarsUsageDescription key to inform users about the purpose of the access.
NSCalendarsUsageDescription This app needs access to your calendar to manage your events and reminders.
2. Handle Permissions Gracefully: Implement robust checks for calendar access permissions before performing any operations with EventKit. Create reusable functions to streamline the process of requesting access and handling different authorization statuses. This not only promotes code reusability but also ensures that your app behaves predictably regardless of the user’s permission settings.
func checkCalendarAuthorization(completion: @escaping (Bool) -> Void) { let eventStore = EKEventStore() let status = EKEventStore.authorizationStatus(for: .event) switch status { case .authorized: completion(true) case .denied: print("Access denied to calendar.") completion(false) case .notDetermined: eventStore.requestAccess(to: .event) { (granted, error) in if granted { print("Access granted to calendar.") completion(true) } else { print("Access denied: (String(describing: error?.localizedDescription))") completion(false) } } case .restricted: print("Calendar access is restricted.") completion(false) default: completion(false) } }
3. Provide Feedback to Users: Whenever a user performs an action that requires calendar access, provide immediate feedback. If access is denied, inform users why certain features are unavailable and offer alternative paths, if possible. Maintaining user engagement and satisfaction very important in creating a positive experience.
4. Optimize Event Queries: When fetching events or reminders, use predicates to narrow down the search results efficiently. This approach not only reduces the load on the device but also speeds up data retrieval, allowing for a seamless user experience. For example, when searching for events, specify a date range to limit the results to relevant entries.
func fetchEvents(startDate: Date, endDate: Date) { let eventStore = EKEventStore() eventStore.requestAccess(to: .event) { (granted, error) in guard granted else { return } let predicate = eventStore.predicateForEvents(withStart: startDate, end: endDate, calendars: nil) let events = eventStore.events(matching: predicate) // Process events... } }
5. Handle Errors Gracefully: EventKit operations can fail due to various reasons, such as lack of permissions or invalid data. Always wrap your save operations in do-catch blocks and provide meaningful error messages to users when something goes wrong. This not only enhances robustness but also builds trust with your users.
do { try eventStore.save(event, span: .thisEvent) print("Event created: (event.title)") } catch { print("Error saving event: (error.localizedDescription)") }
6. Test on Real Devices: While the simulator is a fantastic tool for development, always test your EventKit functionalities on actual devices. Permissions and event handling can behave differently on physical devices, and ensuring that your app performs well across various scenarios will help you deliver a polished final product.
By following these best practices, developers can harness the full potential of the EventKit framework while providing users with a respectful and efficient experience in managing their calendar and reminder data. In doing so, you enhance usability and foster a trusting relationship with your users, paving the way for a successful application that meets their scheduling needs.
Source: https://www.plcourses.com/swift-and-eventkit/