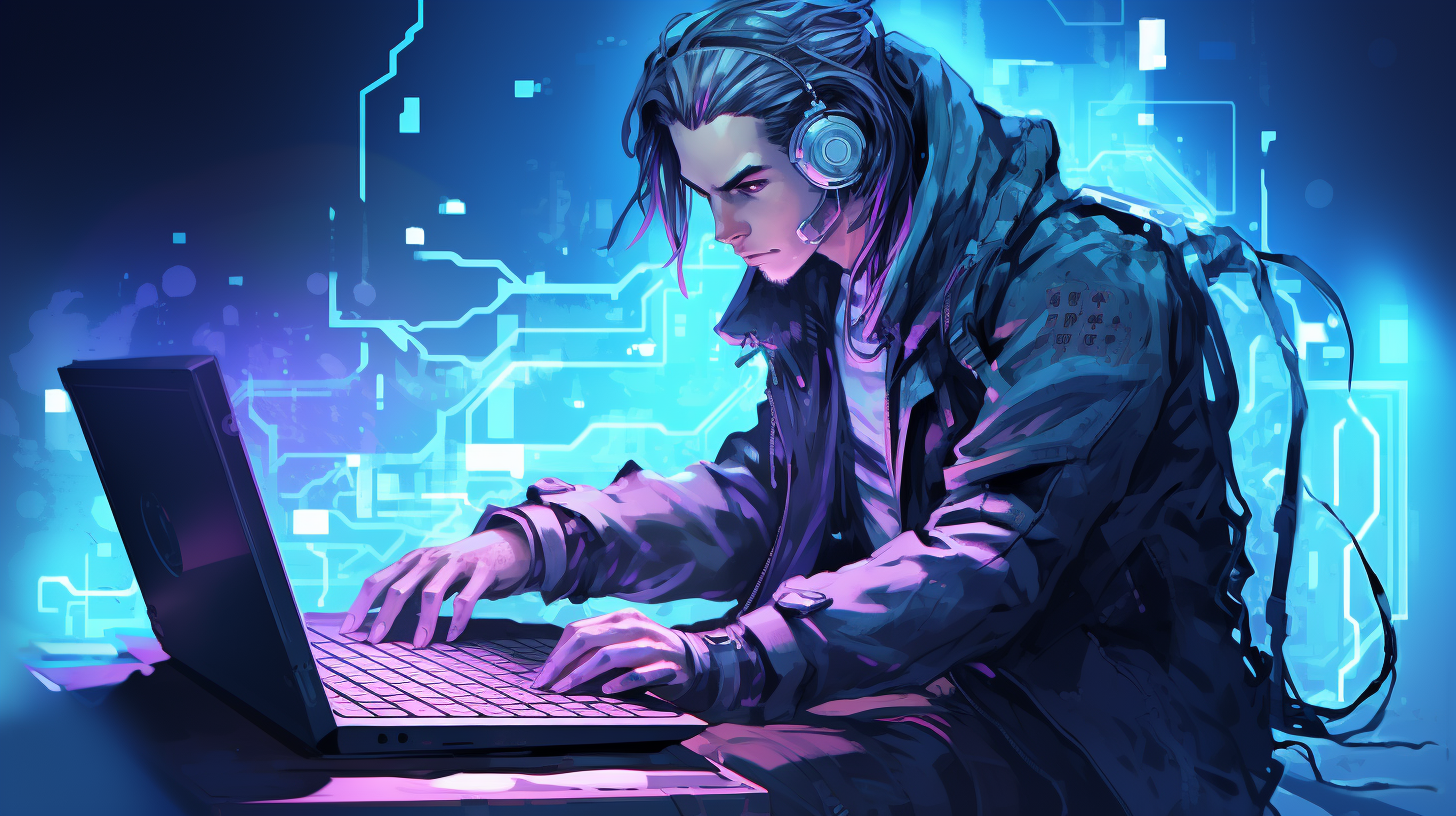
The Java Collections Framework (JCF) provides a set of interfaces and classes that allow developers to manage a group of objects more effectively. At the core of the JCF are a number of interfaces that define the basic structure and behavior of collections.
Key interfaces in the Java Collections Framework include Collection, List, Set, Map, and Queue. Each of these interfaces serves a specific purpose, and they extend one another in a hierarchical manner, allowing for a versatile and powerful way to handle collections of objects.
The Collection interface is the root of the collection hierarchy. It defines basic operations such as add
, remove
, clear
, and size
. Implementations of this interface can be categorized into two main types: Sets and Lists.
The List interface extends Collection and allows for an ordered collection that can contain duplicate elements. The most commonly used implementations of the List interface are ArrayList and LinkedList. Here’s a simple example demonstrating the use of an ArrayList:
import java.util.ArrayList; public class ListExample { public static void main(String[] args) { ArrayList<String> fruits = new ArrayList<>(); fruits.add("Apple"); fruits.add("Banana"); fruits.add("Cherry"); System.out.println("Fruits: " + fruits); } }
On the other hand, the Set interface represents a collection that cannot contain duplicate elements. The most prominent implementations of Set are HashSet, LinkedHashSet, and TreeSet. Below is an example of using a HashSet:
import java.util.HashSet; public class SetExample { public static void main(String[] args) { HashSet<String> uniqueNumbers = new HashSet<>(); uniqueNumbers.add("One"); uniqueNumbers.add("Two"); uniqueNumbers.add("One"); // Duplicate will not be added System.out.println("Unique Numbers: " + uniqueNumbers); } }
Next, the Map interface is designed to store key-value pairs, where each key is unique, and each key maps to exactly one value. The most widely used implementations include HashMap, LinkedHashMap, and TreeMap. Here’s an example of how to use a HashMap:
import java.util.HashMap; public class MapExample { public static void main(String[] args) { HashMap<String, Integer> map = new HashMap<>(); map.put("Apple", 1); map.put("Banana", 2); map.put("Cherry", 3); System.out.println("Map: " + map); } }
Lastly, the Queue interface represents a collection designed for holding elements prior to processing. It is typically used in scenarios where elements are processed in a first-in-first-out (FIFO) manner. Implementations like LinkedList and PriorityQueue are commonly used, depending on the need for ordering. Consider the following example using a PriorityQueue:
import java.util.PriorityQueue; public class QueueExample { public static void main(String[] args) { PriorityQueue<Integer> priorityQueue = new PriorityQueue<>(); priorityQueue.add(5); priorityQueue.add(1); priorityQueue.add(3); System.out.println("Priority Queue: " + priorityQueue); } }
These foundational interfaces provide the blueprint for creating and manipulating various collections in Java, making it easier to handle data in an organized and efficient manner.
Commonly Used Collection Implementations
Understanding the commonly used collection implementations within the Java Collections Framework very important for using its full potential. Each implementation is tailored to specific use cases, optimizing for performance in terms of speed and memory usage.
The ArrayList implementation of the List interface is particularly popular due to its ability to dynamically resize and allow fast random access. ArrayLists use an array to store elements, making it ideal for scenarios where frequent access is needed but modifications, such as adding or removing elements, occur less frequently. Here’s an example showcasing the properties of an ArrayList:
import java.util.ArrayList; public class ArrayListExample { public static void main(String[] args) { ArrayList<String> colors = new ArrayList<>(); colors.add("Red"); colors.add("Green"); colors.add("Blue"); // Accessing elements System.out.println("First color: " + colors.get(0)); // Modifying elements colors.set(1, "Yellow"); System.out.println("Colors after modification: " + colors); } }
In contrast, the LinkedList is another List implementation that’s optimized for insertions and deletions. It maintains a doubly-linked list structure which allows for efficient modification of elements but does not provide the same performance for random access. The following example illustrates the use of a LinkedList:
import java.util.LinkedList; public class LinkedListExample { public static void main(String[] args) { LinkedList<String> animals = new LinkedList<>(); animals.add("Dog"); animals.add("Cat"); animals.addFirst("Rabbit"); // Adding at the start System.out.println("Animals: " + animals); } }
The HashSet, a widely used Set implementation, stores elements in a hash table, which allows for constant-time performance for basic operations such as add, remove, and contains, provided the hash function disperses elements properly. However, unlike a List, there are no guarantees regarding the iteration order. Here is how you can use a HashSet:
import java.util.HashSet; public class HashSetExample { public static void main(String[] args) { HashSet<String> set = new HashSet<>(); set.add("Apple"); set.add("Banana"); set.add("Apple"); // Duplicate will not be added System.out.println("HashSet: " + set); } }
For ordered collections, LinkedHashSet combines the benefits of a hash table with a linked list, maintaining the order of insertion. This makes it useful when you require both uniqueness and the original order of elements:
import java.util.LinkedHashSet; public class LinkedHashSetExample { public static void main(String[] args) { LinkedHashSet<String> linkedHashSet = new LinkedHashSet<>(); linkedHashSet.add("One"); linkedHashSet.add("Two"); linkedHashSet.add("Three"); System.out.println("LinkedHashSet: " + linkedHashSet); } }
The TreeSet is another Set implementation that stores elements in a sorted order, using a red-black tree structure. This allows for operations like range views and ordered operations to be performed efficiently. Here’s an example demonstrating TreeSet:
import java.util.TreeSet; public class TreeSetExample { public static void main(String[] args) { TreeSet<Integer> treeSet = new TreeSet<>(); treeSet.add(5); treeSet.add(1); treeSet.add(3); System.out.println("TreeSet (sorted): " + treeSet); } }
Moving on to the Map interface, the HashMap provides a fast and efficient way to store key-value pairs with average constant time complexity for basic operations. Here’s a practical example to illustrate its functionality:
import java.util.HashMap; public class HashMapExample { public static void main(String[] args) { HashMap<String, Integer> map = new HashMap<>(); map.put("One", 1); map.put("Two", 2); map.put("Three", 3); System.out.println("HashMap: " + map); } }
If the order of entries is significant, the LinkedHashMap is the go-to choice, as it maintains the insertion order. The following snippet shows its usage:
import java.util.LinkedHashMap; public class LinkedHashMapExample { public static void main(String[] args) { LinkedHashMap<String, Integer> linkedMap = new LinkedHashMap<>(); linkedMap.put("A", 1); linkedMap.put("B", 2); linkedMap.put("C", 3); System.out.println("LinkedHashMap: " + linkedMap); } }
The TreeMap provides a sorted map functionality, allowing for ordered key-value pairs based on the natural ordering of the keys or a specified comparator:
import java.util.TreeMap; public class TreeMapExample { public static void main(String[] args) { TreeMap<String, Integer> treeMap = new TreeMap<>(); treeMap.put("C", 3); treeMap.put("A", 1); treeMap.put("B", 2); System.out.println("TreeMap (sorted): " + treeMap); } }
Lastly, the Queue interface is realized in various ways, with the PriorityQueue being a notable implementation that orders elements based on their natural ordering or a comparator. That’s particularly useful in scenarios like scheduling tasks:
import java.util.PriorityQueue; public class PriorityQueueExample { public static void main(String[] args) { PriorityQueue<Integer> priorityQueue = new PriorityQueue<>(); priorityQueue.add(4); priorityQueue.add(1); priorityQueue.add(3); System.out.println("Priority Queue (ordered): " + priorityQueue); } }
This exploration of commonly used collection implementations reveals the versatility and power of the Java Collections Framework, facilitating developers in choosing the right tool for the specific requirements of their applications.
Working with Collections: Algorithms and Utilities
Working with collections in Java is not just about storing data but also about manipulating and processing that data efficiently. The Java Collections Framework offers a rich set of algorithms and utilities that make it easy to perform operations on collections. These tools can simplify complex tasks, enhance performance, and improve code readability.
Java provides a class named Collections that contains static methods for operating on or returning collections. This includes methods for sorting, searching, reversing, shuffling, and more. These utility methods allow developers to apply complex operations with a simple method call, which can significantly reduce the amount of boilerplate code required.
For instance, think the following example where we use the Collections.sort() method to sort a list of strings:
import java.util.ArrayList; import java.util.Collections; public class SortExample { public static void main(String[] args) { ArrayList<String> names = new ArrayList<>(); names.add("Charlie"); names.add("Alice"); names.add("Bob"); System.out.println("Before sorting: " + names); // Sorting the list Collections.sort(names); System.out.println("After sorting: " + names); } }
In this example, the Collections.sort() method sorts the elements of the ArrayList in natural order. By using this utility, developers can avoid writing their own sorting logic, thus adhering to the DRY (Don’t Repeat Yourself) principle.
Another useful method is Collections.shuffle(), which randomly shuffles the elements of a list. This can be particularly handy in scenarios like card games or when needing randomized data for testing.
import java.util.ArrayList; import java.util.Collections; public class ShuffleExample { public static void main(String[] args) { ArrayList<String> deck = new ArrayList<>(); deck.add("Ace"); deck.add("King"); deck.add("Queen"); System.out.println("Before shuffling: " + deck); // Shuffling the deck Collections.shuffle(deck); System.out.println("After shuffling: " + deck); } }
Besides these, searching through collections is also simplified with the Collections.binarySearch() method, which requires the list to be sorted. This method uses a binary search algorithm to find the index of a specified element, offering significant performance advantages compared to a linear search.
import java.util.ArrayList; import java.util.Collections; public class BinarySearchExample { public static void main(String[] args) { ArrayList<Integer> numbers = new ArrayList<>(); numbers.add(3); numbers.add(1); numbers.add(2); // Sorting the list before binary search Collections.sort(numbers); System.out.println("Sorted Numbers: " + numbers); int index = Collections.binarySearch(numbers, 2); System.out.println("Index of 2: " + index); } }
In addition to these operations, the Collections class provides methods like reverse() and fill(), which allow for easy manipulation of a collection’s contents. Such built-in functionality demonstrates the framework’s ability to handle common tasks with minimal fuss, enabling developers to focus on the unique aspects of their applications.
Moreover, Java 8 introduced the Stream API, which complements the collections framework by providing a way to process sequences of elements in a functional style. Streams enable operations such as filtering, mapping, and reducing, allowing for complex operations on collections to be expressed clearly and concisely.
Here’s an example of using streams to filter a list of integers:
import java.util.Arrays; import java.util.List; import java.util.stream.Collectors; public class StreamExample { public static void main(String[] args) { List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6); // Filtering even numbers using Stream API List<Integer> evenNumbers = numbers.stream() .filter(n -> n % 2 == 0) .collect(Collectors.toList()); System.out.println("Even Numbers: " + evenNumbers); } }
As you can see, the Stream API not only simplifies the syntax but also enhances the readability of code, allowing for more expressive operations on collections.
The Java Collections Framework equips developers with powerful algorithms and utilities that streamline data manipulation within collections. By using the built-in capabilities of the framework, you can write more efficient, maintainable code while focusing on achieving your application’s goals.
Best Practices for Using Java Collections
When using the Java Collections Framework, adhering to best practices is vital to ensure that your code is efficient, maintainable, and easy to understand. Here are several guidelines to follow when working with collections in Java.
1. Choose the Right Collection Type
Selecting the correct collection type for your specific use case is important. Understand the properties and performance characteristics of each collection implementation. For instance, if you need fast random access and retrieval, opt for an ArrayList
. If your application requires frequent insertions and deletions, consider a LinkedList
. For unique elements, a HashSet
is a solid choice. Below is an example illustrating the need for the appropriate collection type:
import java.util.ArrayList; import java.util.HashSet; public class CollectionTypeExample { public static void main(String[] args) { // Fast access ArrayList<String> list = new ArrayList<>(); list.add("Apple"); list.add("Banana"); // Only unique elements HashSet<String> set = new HashSet<>(); set.add("Apple"); set.add("Apple"); // Duplicate does not get added System.out.println("List: " + list); System.out.println("Set: " + set); } }
2. Use Generics
Generics provide type safety and eliminate the need for explicit type casting. They ensure that only the specified object types can be added to a collection. By using generics, you can catch type-related errors at compile-time rather than run-time. Here’s how to properly implement generics in a List
:
import java.util.List; import java.util.ArrayList; public class GenericsExample { public static void main(String[] args) { List<String> names = new ArrayList<>(); names.add("Alice"); names.add("Bob"); // The following line would cause a compile-time error // names.add(1); // Incorrect type System.out.println("Names: " + names); } }
3. Avoid Using Raw Types
Using raw types can lead to potential runtime issues, as it bypasses compile-time type checking. Always prefer using parameterized types to retain type safety:
import java.util.HashMap; public class RawTypeExample { public static void main(String[] args) { // Avoid this - raw type HashMap map = new HashMap(); map.put("One", 1); // Instead, use HashMap<String, Integer> typedMap = new HashMap<>(); typedMap.put("One", 1); System.out.println("Typed Map: " + typedMap); } }
4. Prefer Immutable Collections When Possible
Using immutable collections can lead to safer and more predictable code, as it prevents inadvertent modifications of the collection. Java 9 introduced factory methods for creating immutable collections:
import java.util.List; public class ImmutableCollectionExample { public static void main(String[] args) { List<String> immutableList = List.of("Apple", "Banana", "Cherry"); // This line will throw an UnsupportedOperationException // immutableList.add("Date"); System.out.println("Immutable List: " + immutableList); } }
5. Use the Enhanced For-Loop
When iterating over collections, the enhanced for-loop (or for-each loop) provides a cleaner and more readable syntax. It avoids the complexity of using iterators or index-based loops, thereby reducing the risk of errors:
import java.util.ArrayList; public class EnhancedForLoopExample { public static void main(String[] args) { ArrayList<String> fruits = new ArrayList<>(); fruits.add("Apple"); fruits.add("Banana"); fruits.add("Cherry"); // Using enhanced for-loop for (String fruit : fruits) { System.out.println("Fruit: " + fruit); } } }
6. Be Mindful of Concurrent Modifications
When modifying a collection while iterating over it, you may encounter ConcurrentModificationException
. To avoid this, think using the Iterator
directly or using concurrent collections like CopyOnWriteArrayList
:
import java.util.ArrayList; import java.util.Iterator; public class ConcurrentModificationExample { public static void main(String[] args) { ArrayList<String> items = new ArrayList<>(); items.add("One"); items.add("Two"); // Correct way using Iterator Iterator<String> iterator = items.iterator(); while (iterator.hasNext()) { String item = iterator.next(); // You can safely remove items if ("One".equals(item)) { iterator.remove(); } } System.out.println("Items after removal: " + items); } }
By adhering to these best practices, you not only enhance the performance and readability of your code but also make it easier for others to maintain. The Java Collections Framework offers a powerful suite of tools, and using them wisely can lead to robust and efficient applications.
Source: https://www.plcourses.com/java-collections-framework-overview/