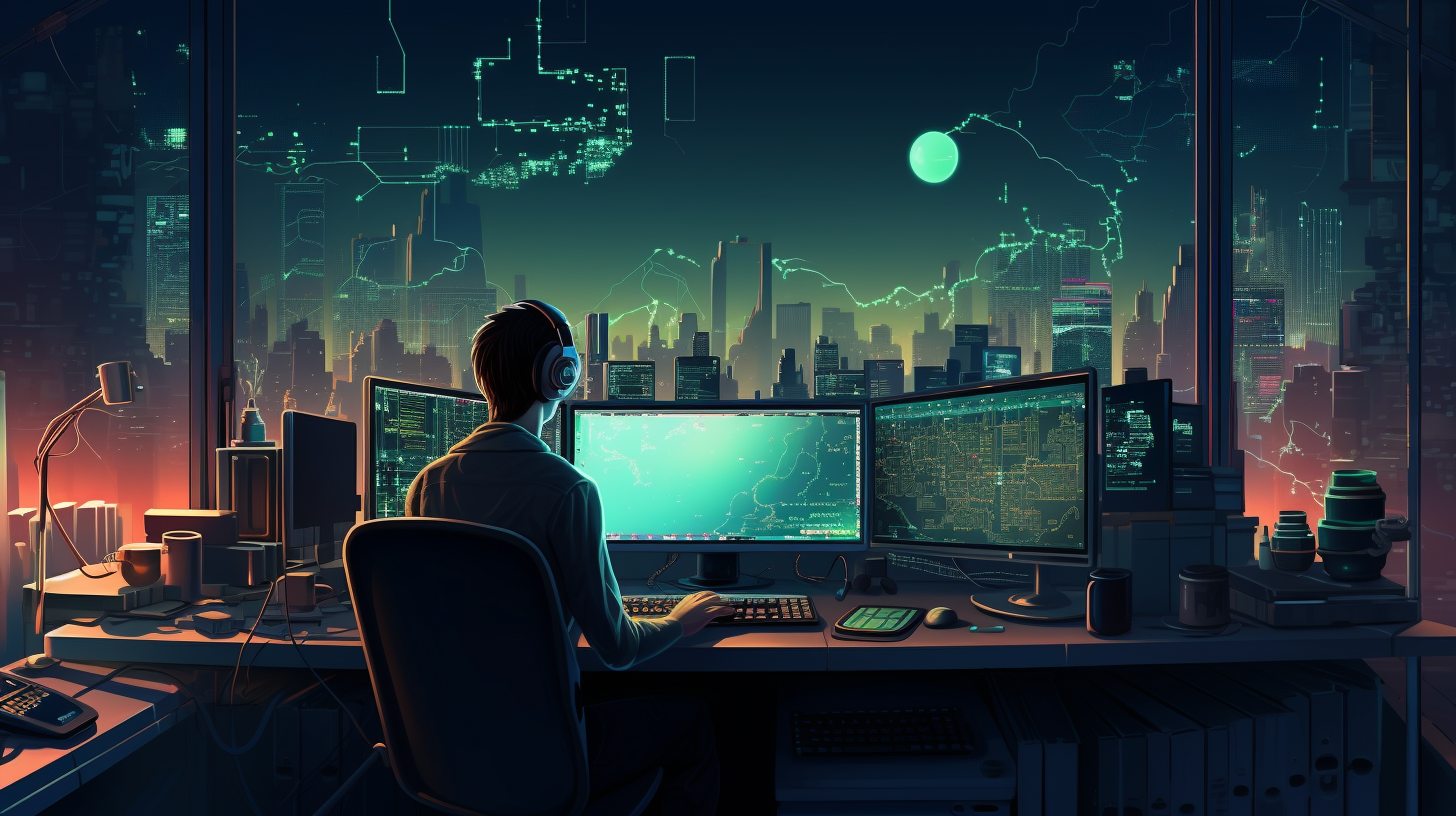
Code refactoring in Java is a fundamental practice that entails restructuring existing code without changing its external behavior. The goal is to improve nonfunctional attributes of the software, making it easier to understand, maintain, and extend. This process can illuminate hidden issues, enhance code readability, and ultimately lead to a more manageable codebase.
At its core, refactoring is about addressing code smells—those indicators that something might be wrong with the code. These smells can manifest as duplicate code, overly complex methods, or classes that have too many responsibilities. By refactoring, developers can simplify their code, making it cleaner and more efficient.
One common analogy is to consider of code as a garden. Over time, weeds (or code smells) can proliferate, overshadowing the healthy plants (well-structured code). Regularly tending to this garden through refactoring helps ensure that the garden remains vibrant and capable of producing the desired fruits (features, updates).
In Java, refactoring typically involves a series of small, incremental changes. This method is preferable because it minimizes the risk of introducing bugs. Each change should be tested immediately to confirm that the behavior of the program remains consistent. Tools like JUnit can aid in this process by automating unit tests.
For example, ponder a class that calculates the area of different shapes. As more shapes are added, the code can become unwieldy. A refactoring technique, such as extracting methods, can be applied:
public class AreaCalculator { public double calculateArea(String shape, double... dimensions) { switch (shape.toLowerCase()) { case "circle": return calculateCircleArea(dimensions[0]); case "rectangle": return calculateRectangleArea(dimensions[0], dimensions[1]); default: throw new IllegalArgumentException("Unknown shape: " + shape); } } private double calculateCircleArea(double radius) { return Math.PI * radius * radius; } private double calculateRectangleArea(double length, double width) { return length * width; } }
In this example, the area calculation for each shape is encapsulated in its own method, which enhances readability and allows for easier testing of individual components. By applying such techniques consistently, developers can build a robust codebase that adapts to changing requirements while maintaining its integrity.
Moreover, understanding when to refactor very important. It’s generally advisable to refactor during the development process—when new features are being added or bugs are being fixed. This proactive approach helps prevent technical debt from accumulating and keeps the codebase healthy.
Understanding code refactoring in Java is not merely a technical skill but a mindset that emphasizes quality and maintainability. It’s about fostering a culture that appreciates clean code and continuous improvement, which is vital for any successful software project.
Common Refactoring Techniques
Refactoring techniques in Java are a high number of, each serving distinct purposes that address specific code smells and improve overall code quality. Here, we delve into several common refactoring techniques that every Java developer should be familiar with, providing practical code examples for clarity.
Extract Method is a potent technique for simplifying complex methods. When a method does too much, it can be broken down into smaller, more focused methods. This enhances readability and reusability. For instance, ponder the following method that processes customer orders:
public void processOrder(Order order) { // Validate order if (!validateOrder(order)) { return; } // Process payment processPayment(order); // Prepare shipment prepareShipment(order); }
Here, the processOrder
method can benefit from extraction:
public void processOrder(Order order) { if (!validateOrder(order)) { return; } handlePaymentAndShipment(order); } private void handlePaymentAndShipment(Order order) { processPayment(order); prepareShipment(order); }
This restructuring clarifies the responsibilities of the original method, allowing developers to grasp its functionality at a glance.
Rename Method is another crucial technique, especially when a method name does not accurately convey its purpose. A well-named method enhances code clarity. If we have a method named doStuff
, it is ambiguous and requires renaming. For example:
public void doStuff(Customer customer) { // code to send a welcome email }
Renaming it to sendWelcomeEmail
conveys the action much more effectively:
public void sendWelcomeEmail(Customer customer) { // code to send a welcome email }
Inline Method is the reverse of extract method—it is useful when a method’s body is as clear as its name. For example, think this method:
private double getBasePrice() { return basePrice; }
Instead of keeping a separate method for this simpler retrieval, we can inline it directly where it is used:
public double calculateFinalPrice() { return basePrice * taxRate; // Inline the method }
Move Method and Move Field involve relocating methods or fields from one class to another to enhance cohesion. For instance, if a method primarily operates on another class’s data, it might make sense to move it there. Suppose we have a method in a Customer
class that calculates discounts based on order history:
public class Customer { public double calculateDiscount(Order order) { // logic for discount calculation } }
If this logic is more relevant to the Order
class, moving the method can enhance cohesion:
public class Order { public double calculateDiscount() { // logic for discount calculation } }
Extract Class is a beneficial technique for handling large classes with multiple responsibilities. By identifying grouped fields and methods, developers can create a new class to encapsulate these functionalities. For instance, in a class managing customers and their orders:
public class CustomerOrderManager { private Customer customer; private List orders; // Methods to manage customers and orders }
We can extract order management into its own class:
public class Customer { // Customer-related methods } public class OrderManager { private List orders; // Methods to manage orders }
Implementing these common refactoring techniques can significantly enhance the quality and maintainability of Java code. By adopting a mindset focused on continuous improvement, developers can create a robust and agile codebase that’s well-suited to evolving project requirements.
Best Practices for Effective Refactoring
When it comes to effective refactoring in Java, adhering to best practices can significantly enhance not only the quality of your code but also the efficiency of your refactoring process. Here are some key practices to consider:
1. Refactor in Small Steps
It’s essential to approach refactoring incrementally. Make small, manageable changes and test after each modification. This minimizes the risk of introducing bugs and allows for easier identification of issues should they arise. For instance, if you are tasked with refactoring a class, consider changing one method at a time and validating the application’s functionality after each change:
public class User { private String username; private String email; public User(String username, String email) { this.username = username; this.email = email; } // Refactor one method at a time public String getUserInfo() { return username + " "; } }
After testing the getUserInfo()
method, you would proceed to refactor the next method, ensuring that the application continues to function as expected.
2. Keep the Code Functionality Intact
During the refactoring process, the primary objective is to ensure that the external behavior of the code remains unchanged. This is where thorough testing comes into play. Having a solid suite of unit tests is invaluable. For instance, if you are refactoring a method to improve its readability, ensure that the output remains consistent:
public double calculateArea(double radius) { return Math.PI * Math.pow(radius, 2); } // Refactor to enhance readability public double calculateCircleArea(double radius) { return Math.PI * radius * radius; }
Both methods should return the same result for the same input. This ensures that your refactoring does not alter functionality.
3. Embrace Automated Testing
Automated testing plays an important role in effective refactoring. Using tools such as JUnit can streamline the testing process, enabling you to run tests rapidly after each refactor. Make it a routine to add tests for new features or scenarios as you refactor existing code. For example:
@Test public void testCalculateCircleArea() { double radius = 5; double expectedArea = Math.PI * radius * radius; assertEquals(expectedArea, calculateCircleArea(radius), 0.001); }
This test ensures that your refactored method produces the correct output, providing confidence that the refactor maintains existing functionality.
4. Prioritize Readability and Maintainability
Code should be easy to read and understand. As you refactor, strive for clarity. This could involve renaming variables, methods, or classes to more accurately reflect their purpose, or breaking down complex methods into simpler, smaller ones. A method should generally handle a singular task. For instance:
public void handleUserCreation(String username, String email) { User user = new User(username, email); sendWelcomeEmail(user); logUserCreation(user); } // Refactor for clarity private void createUser(String username, String email) { User user = new User(username, email); sendWelcomeEmail(user); } private void logUserCreation(User user) { // logging logic }
By separating concerns, you enhance the maintainability of the code, making it easier for others (or yourself) to understand and modify in the future.
5. Remove Dead Code
During refactoring, take the opportunity to eliminate unused code. Dead code clutters your codebase and can lead to confusion. If you find methods that are never called or variables that are never used, remove them. This practice keeps the code clean and minimizes potential sources of bugs:
public void unusedMethod() { // This method is never called; ponder removing it. }
By adhering to these best practices, you not only improve the quality of your code but also foster a healthy coding environment that values clarity, efficiency, and maintainability. This mindset is essential for any developer looking to create robust and adaptable Java applications.
Tools and IDEs for Java Refactoring
When it comes to refactoring in Java, having the right tools and Integrated Development Environments (IDEs) can make a significant difference in both efficiency and effectiveness. Refactoring tools can automate many processes that would otherwise be time-consuming and error-prone if done manually. They assist in ensuring that code modifications do not alter the expected behavior while allowing developers to focus on improving code quality. Below, we will explore some of the most popular tools and IDEs for Java refactoring, detailing their features and benefits.
Eclipse is a widely used IDE that offers a robust set of refactoring tools. Its built-in capabilities allow for refactoring operations such as renaming, moving, changing method signatures, and extracting methods with ease. Eclipse provides real-time feedback, highlighting potential issues as changes are made. The IDE also supports automated testing, which very important during the refactoring process. For instance, when renaming a method, Eclipse automatically updates all references to that method across the project, reducing the risk of introducing errors.
public class SampleClass { public void oldMethodName() { // method logic } } // Renaming is handled automatically by Eclipse public void newMethodName() { // method logic }
IntelliJ IDEA is another powerful IDE known for its intelligent code assistance features. It provides a wide range of refactoring options and offers suggestions for improvements. Features like “Safe Delete” ensure that developers can remove unused code without fear of breaking existing functionality. IntelliJ’s refactoring capabilities include inline method extraction, changing method parameters, and more. For example, if a developer wants to extract a method, IntelliJ will highlight the code and allow for easy extraction with a simple keyboard shortcut.
public class Calculator { public double add(double a, double b) { return a + b; } } // Extracting the addition logic will create a new method private double performAddition(double a, double b) { return a + b; }
NetBeans is also equipped with powerful refactoring tools that can handle various tasks, including renaming classes, methods, and variables. NetBeans supports the extraction of interfaces and classes as well, making it suitable for larger refactoring tasks. Its simpler interface allows for quick access to refactoring options, enabling developers to make changes swiftly without losing sight of context.
public class OrderProcessor { public void processOrder(Order order) { // processing logic } } // Refactoring can include extracting an interface for processing public interface OrderProcessing { void processOrder(Order order); }
JArchitect is a specialized tool that focuses on analyzing code quality and architecture, offering insights that can guide refactoring efforts. It provides a visual representation of code dependencies, allowing developers to identify problematic areas before proceeding with refactoring. This tool is especially useful for larger codebases where understanding interdependencies can be challenging.
Refactoring Browser is a lightweight option for those who prefer a more simpler approach to refactoring. While it may not have all the advanced features of larger IDEs, it offers essential functionality for renaming, moving, and extracting methods. This tool is beneficial for quick refactoring tasks or when working in environments where a full IDE may not be necessary.
In addition to these tools, there are various libraries and plugins available that enhance the refactoring capabilities of existing IDEs. For example, plugins for static code analysis can help identify code smells or areas needing refactoring, offering suggestions based on best practices.
Ultimately, the choice of tools and IDEs for Java refactoring can significantly impact a developer’s productivity and code quality. By using these resources, developers can streamline their refactoring processes, reduce errors, and maintain a clean, efficient codebase. The combination of automated tools and best practices is essential in fostering a culture of continuous improvement in software development.
Automated Refactoring: Pros and Cons
Automated refactoring in Java has emerged as a significant boon for developers striving to maintain high-quality codebases. It leverages sophisticated algorithms and tools to perform refactoring tasks, ensuring both efficiency and consistency in the code transformation process. However, like any tool, automated refactoring comes with its own set of advantages and disadvantages that developers must ponder.
Pros of Automated Refactoring:
One of the most compelling advantages of automated refactoring is the reduction of manual effort. Tools such as IntelliJ IDEA, Eclipse, and NetBeans offer built-in refactoring capabilities that allow developers to execute complex refactorings with just a few clicks. For instance, renaming a method across an entire codebase can be done seamlessly:
public class Example { public void oldMethod() { // some logic } } // Renaming using automated tools public void newMethod() { // some logic }
This automation mitigates the risk of human error, ensuring that all references to the method are updated consistently. That is particularly beneficial in large projects where the same method may be utilized in a high number of locations.
Another advantage is the speed of execution. Automated refactoring tools can process changes much faster than a developer manually combing through the code. This speed allows for quicker iterations, making it easier to implement frequent refactoring as part of the development cycle.
Automated tools also enhance the overall quality of code by helping enforce best practices. They can analyze code patterns and suggest improvements, such as identifying code smells or areas where specific refactoring techniques should be applied. For example, a tool may suggest extracting a method if it detects a method that’s too long or complex:
public void complexMethod() { // long and complex logic } // Tool recommends extracting this logic into a new method private void extractedMethod() { // extracted logic }
Additionally, automated refactoring aids in maintaining a robust suite of unit tests. When changes are made, developers can quickly run existing tests to verify that the refactoring has not altered the application’s behavior. This integration of refactoring with testing especially important in agile environments where rapid changes are commonplace.
Cons of Automated Refactoring:
Despite its benefits, automated refactoring is not without drawbacks. One significant concern is the potential for over-reliance on tools. Developers may become complacent, trusting automated processes without a thorough understanding of the underlying code and its implications. This can lead to scenarios where tools make changes that are syntactically correct but semantically inappropriate for the context.
Moreover, automated tools may not fully grasp the business logic embedded within the code. The nuances of certain refactorings may require a developer’s judgment—a perspective that tools lack. For instance, while a tool can easily rename methods, it might not ponder the broader implications of such changes on system functionality or user experience.
Another downside is that automated refactoring may introduce new bugs, particularly in complex systems where interactions between components are intricate. The risk of inadvertently breaking functionality exists, especially if the tool’s understanding of the code structure is incomplete or flawed. For example, renaming a method might not update all dependent classes correctly if they are not part of the same module:
public class DependentClass { public void callOldMethod() { new Example().oldMethod(); // Still calling old method } }
Additionally, not all refactoring tasks can or should be automated. Some require a deep understanding of the domain, and developers must prioritize certain refactorings based on their impact on the codebase. Consequently, automated tools should be viewed as aids rather than replacements for a developer’s expertise.
Automated refactoring offers a powerful way to enhance code quality and efficiency in Java development, yet it’s essential to balance its usage with human insight. By understanding both its strengths and limitations, developers can effectively employ automated refactoring as part of a broader strategy aimed at maintaining clean, maintainable code.
Refactoring Case Studies and Examples
public class Order { private double totalAmount; private List items; public Order() { items = new ArrayList(); } public void addItem(Item item) { items.add(item); totalAmount += item.getPrice(); } public double calculateTotal() { return totalAmount; } // Example of where refactoring could be applied public void printOrderDetails() { System.out.println("Order Details:"); for (Item item : items) { System.out.println(item.getName() + ": " + item.getPrice()); } System.out.println("Total Amount: " + calculateTotal()); } }
In a scenario where the `Order` class grows cumbersome due to added responsibilities, refactoring can simplify its design. Let’s say we need to add a new feature that calculates discounts. Instead of cluttering the `Order` class, we could extract discount logic into a new class:
public class DiscountCalculator { public double applyDiscount(Order order, double discountPercentage) { double discount = order.calculateTotal() * (discountPercentage / 100); return order.calculateTotal() - discount; } }
Now, the `Order` class remains focused on managing items and total calculations, while the `DiscountCalculator` class encapsulates the discount logic. This separation of concerns not only improves code readability but also enhances maintainability, as each class has a clear responsibility.
Another practical example can be found in the classic game of Tic-Tac-Toe. Suppose we begin by implementing the game in a single class:
public class TicTacToe { private char[][] board = new char[3][3]; private char currentPlayer; public void playGame() { // game logic } private void printBoard() { // print the board } private boolean checkWinner() { // check for a winner } }
As the game evolves, we might find ourselves needing to add more features—like a graphical user interface (GUI), scoring, or even an AI opponent. At this point, the class can become unwieldy. To alleviate this, we could refactor it into multiple classes, ensuring each class handles a specific aspect of the game:
public class Game { private Board board; private Player player1; private Player player2; public void start() { // game initiation logic } } public class Board { private char[][] grid; public void print() { // print board logic } public boolean isFull() { // check if board is full } } public class Player { private String name; private char symbol; public void makeMove(Board board) { // logic for making a move } }
This approach not only clarifies the functionality of each class but also allows for easier testing and future extensions. Each component can be developed and tested independently, leading to a more robust and flexible codebase.
Such examples illustrate the tangible benefits of refactoring in Java, allowing for improved organization and clarity. By studying real-world applications of these techniques, developers can appreciate the value of refactoring as a means to cultivate cleaner, more efficient code—one incremental change at a time.
Source: https://www.plcourses.com/java-and-code-refactoring-techniques-and-tools/