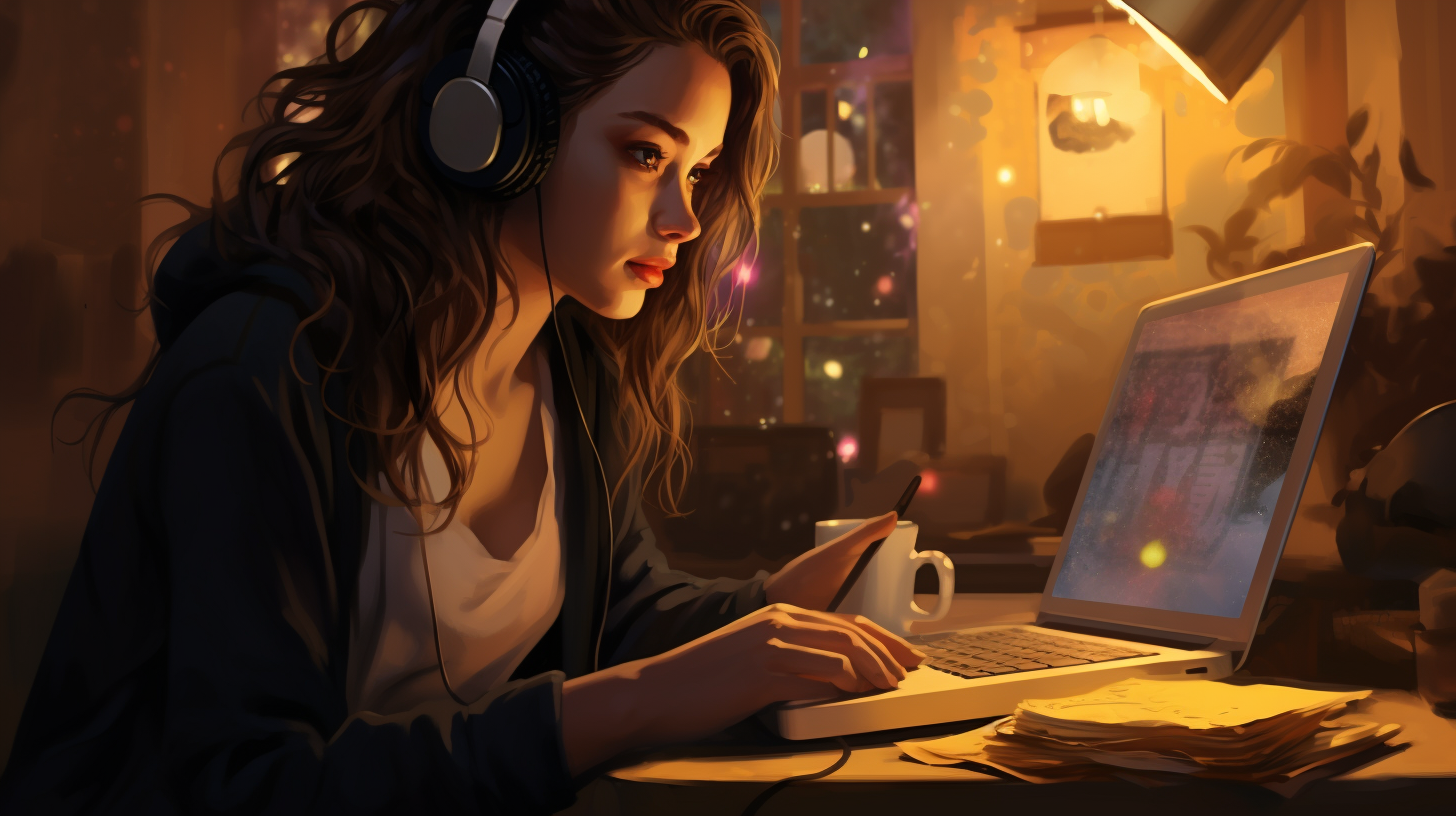
When working with SQL, understanding data types is fundamental to ensuring that your database operates efficiently and accurately. Data types define the nature of the data that can be stored in a column, influencing storage requirements, performance, and how data can be queried and manipulated.
SQL data types can be broadly categorized into several groups, including:
- These include integers, floating-point numbers, and decimal numbers. They are used for any data that involves calculations.
- This category includes fixed-length and variable-length strings. They’re essential for representing textual data.
- Used for storing dates and times, these types support various formats and allow for time-based queries.
- These represent true/false values, often utilized in conditional logic.
- Binary Large Objects and Character Large Objects are used for storing large binary and text data, respectively.
Understanding these categories assists in not only choosing the appropriate type for your data but also in optimizing performance and storage. For instance, selecting an INT
type for a value that will never exceed 255, instead of a BIGINT
, can save significant space. Here’s a practical example:
CREATE TABLE example ( id INT, -- Good choice for small integers name VARCHAR(100), -- Variable-length string with max length created_at DATETIME -- Storing date and time information );
Moreover, each SQL database management system (DBMS) may offer specific implementations for these data types. For instance, while VARCHAR
is standard across SQL, some DBMSs may have unique variations or additional types, such as TEXT
in MySQL, which has no maximum length, or NVARCHAR
for Unicode data in SQL Server.
Understanding SQL data types helps developers make informed decisions that lead to better-performing databases and more efficient queries. The right data type can enhance data integrity and optimize storage, which are essential for maintaining a robust relational database.
Choosing the Right Data Type for Your Needs
Choosing the right data type for your needs is a critical decision that can significantly impact the performance and scalability of your database. A well-chosen data type not only optimizes storage but also enhances data integrity by enforcing the rules and constraints of the data being stored. Here are several key factors to think when selecting data types:
1. Nature of the Data: The first and foremost consideration should be the nature of the data you intend to store. For instance, if you’re dealing with whole numbers, an integer type is appropriate. If your data involves fractional values, then you should opt for floating-point or decimal types. Here’s how you might define a table for a simple inventory system:
CREATE TABLE inventory ( product_id INT PRIMARY KEY, -- Unique identifier for this product product_name VARCHAR(255) NOT NULL, -- Name of this product quantity INT NOT NULL CHECK (quantity >= 0), -- Non-negative quantity price DECIMAL(10, 2) NOT NULL -- Price with two decimal places );
2. Expected Range of Values: Understanding the expected range of values is also crucial. Using a SMALLINT for a value that will never exceed 32,767 instead of a standard INT can save space, particularly in large datasets. Consider this example:
CREATE TABLE employee ( employee_id SMALLINT PRIMARY KEY, -- Small range of employee IDs name VARCHAR(100) NOT NULL, department VARCHAR(50) NOT NULL );
3. Performance Implications: Different data types can also have varying performance implications. In general, smaller data types can lead to faster operations because they consume less memory and storage. This affects not just storage space but also the speed of retrieval and indexing. For example, using CHAR for a fixed-length string can be faster than VARCHAR in certain situations, especially when the length is consistent:
CREATE TABLE fixed_length_names ( name CHAR(20) NOT NULL -- Fixed-length names for quicker access );
4. Future Growth: Anticipate future growth and changes in your data requirements. It’s often beneficial to select a data type that accommodates potential increases in data volume. For example, if you anticipate that customer ID numbers may need to expand in the future, opting for a BIGINT from the start could save a migration headache later:
CREATE TABLE customers ( customer_id BIGINT PRIMARY KEY, -- Accommodating for future expansion first_name VARCHAR(50) NOT NULL, last_name VARCHAR(50) NOT NULL );
5. Compatibility and Standards: Ensure that the data types you choose align with the standards and compatibility requirements of the SQL dialect you’re using. For example, while many systems support VARCHAR, not all may support TEXT or BLOB types in the same way, which could lead to unexpected issues during data operations.
The careful selection of data types is pivotal in SQL database design. By considering the nature of the data, expected value ranges, performance implications, future growth, and compatibility, you can make informed decisions that enhance both the efficiency and robustness of your database schema.
Common Data Types in SQL
Common data types in SQL serve as the backbone of any relational database, providing a structure that dictates how data is stored, manipulated, and retrieved. Understanding the nuances of these types enhances not only the performance of queries but also the overall integrity of the data. Let’s delve into the most prevalent data types found in SQL.
Numeric Types: These are fundamental for any database that requires arithmetic calculations. Numeric types can be further divided into integers and floating-point numbers. For example:
CREATE TABLE sales ( sale_id INT PRIMARY KEY, amount DECIMAL(10, 2) NOT NULL, -- Two decimal places for currency quantity INT NOT NULL );
In this example, DECIMAL(10, 2)
is selected for the monetary amount, allowing for precise representation of values without rounding errors typical of floating-point representations.
Character Types: This category is important for storing textual information. SQL provides both fixed-length (CHAR
) and variable-length (VARCHAR
) strings. For instance:
CREATE TABLE users ( user_id INT PRIMARY KEY, username VARCHAR(50) NOT NULL, -- Variable length for flexibility password CHAR(64) NOT NULL -- Fixed length for hashing );
In this case, VARCHAR(50)
is appropriate for usernames where length may vary, while CHAR(64)
is suitable for passwords hashed into a fixed-length format.
Date and Time Types: SQL provides various data types for handling dates and times, allowing developers to perform time-based operations efficiently. Think this table for events:
CREATE TABLE events ( event_id INT PRIMARY KEY, event_name VARCHAR(100) NOT NULL, event_date DATETIME NOT NULL -- Date and time of the event );
This structure allows for comprehensive date and time management, essential for applications that rely on scheduling and time tracking.
Boolean Types: These types store true/false values, often used in control flow and logical operations. An example can be seen in a user permissions table:
CREATE TABLE permissions ( permission_id INT PRIMARY KEY, user_id INT NOT NULL, can_edit BOOLEAN NOT NULL DEFAULT FALSE -- Default to false );
Here, BOOLEAN
is used to determine if a user has edit permissions, simplifying conditional checks in application logic.
BLOB and CLOB Types: Binary Large Objects (BLOBs) and Character Large Objects (CLOBs) are designed to store large amounts of data, like images or extensive text. They can be defined as follows:
CREATE TABLE documents ( doc_id INT PRIMARY KEY, doc_content CLOB NOT NULL, -- Large text content doc_image BLOB -- Large binary image data );
This allows for flexible storage of non-standard data types, crucial for applications handling multimedia or extensive documentation.
By grasping these common data types, developers can design more efficient and robust databases. Each type serves specific purposes and choosing the right one can lead to significant performance improvements and enhanced data integrity. Remember that the correct application of these data types will not only streamline data management but also facilitate smoother interactions within your database schemas.
Performance Considerations for Data Types
When considering performance in SQL databases, the choice of data types plays a pivotal role. Each data type has its own storage requirements and processing capabilities, which can significantly impact the speed of data retrieval and manipulation. Here we explore several performance-related aspects of data types.
Storage Size and Memory Usage: One of the most simpler ways to enhance performance is by minimizing the amount of memory used by your database. Smaller data types consume less disk space and memory, leading to faster I/O operations. For instance, using a SMALLINT
instead of a regular INT
can save space in tables that only require a limited range of values.
CREATE TABLE short_range ( id SMALLINT PRIMARY KEY, -- Uses only 2 bytes instead of 4 for INT description VARCHAR(100) );
Indexing Efficiency: Indexes are crucial for speeding up data retrieval, but the size of the indexed columns affects their efficiency. Smaller, properly chosen data types lead to smaller indexes, which can be processed faster. For example, consider indexing a column defined as CHAR(10)
versus VARCHAR(100)
. The fixed length of CHAR
can yield faster comparisons in certain scenarios.
CREATE INDEX idx_short_name ON short_names (name CHAR(10));
CPU Usage: The type of data can also affect CPU usage. Operations involving floating-point numbers can be more resource-intensive than those using integer types. Depending on the operations performed, the performance of arithmetic calculations may vary significantly. For instance, if precision isn’t an issue, using INT
or SMALLINT
where applicable can yield performance benefits.
CREATE TABLE calculations ( value1 INT, value2 INT, total INT AS (value1 + value2) -- Simple integer calculations );
Data Type Compatibility: When performing joins or comparisons, ensuring that the data types of the columns involved match can prevent implicit conversions that slow down query performance. For example, joining a table with an INT
column to a SMALLINT
column may introduce overhead due to type conversion. Consistently using the same data type across related tables is a best practice.
CREATE TABLE orders ( order_id INT PRIMARY KEY, customer_id INT -- Ensure same type for joins );
Batch Processing: The way data is processed can also be influenced by data types. Operations such as bulk inserts or updates can be optimized by selecting appropriate data types. For example, performing batch inserts where all data types are as compact as possible can reduce the overhead of transaction logging and improve overall performance.
INSERT INTO short_range (id, description) VALUES (1, 'First entry'), (2, 'Second entry');
The performance considerations surrounding SQL data types are multifaceted, involving not just the size of the data type itself but also its impact on indexing, CPU usage, compatibility, and batch processing. By carefully selecting and optimizing the data types used in your SQL database, you can create a system this is both efficient and scalable, ultimately leading to a more responsive application.
Best Practices for Data Type Usage
When using SQL, adhering to best practices for data type usage is essential to achieve optimal performance and maintainability within your database. Properly chosen data types not only enhance performance but also safeguard data integrity. Here are some key guidelines to consider when working with SQL data types:
1. Be Specific with Data Types: Always choose the most appropriate type for your data. Avoid generic types when more specific options are available. For instance, if you know a particular column will only contain small integer values, opt for SMALLINT
instead of a broader INT
. This saves space and improves performance.
CREATE TABLE products ( product_id SMALLINT PRIMARY KEY, -- Small range, efficient storage price DECIMAL(8, 2) NOT NULL -- Precision for monetary values );
2. Ponder Data Length: When using character types, specify a length that reflects your data’s maximum expected size. For example, if you’re storing postal codes, using a CHAR(5)
for U.S. ZIP codes is more efficient than a VARCHAR(10)
.
CREATE TABLE locations ( location_id INT PRIMARY KEY, postal_code CHAR(5) NOT NULL -- Fixed length for ZIP codes );
3. Use Defaults Wisely: Setting default values for columns can prevent issues with NULL values and ensure data consistency. However, be cautious with defaults, as setting a poorly chosen default may lead to undesirable data states.
CREATE TABLE users ( user_id INT PRIMARY KEY, status VARCHAR(20) DEFAULT 'active' -- Default status );
4. Indexing Considerations: Be mindful of the data types you choose for indexed columns. Smaller data types can significantly reduce the size of indexes, leading to faster query performance. Careful indexing can enhance search operations without compromising data retrieval speed.
CREATE INDEX idx_customer_name ON customers (last_name);
5. Optimize for Joins: When designing tables that will be frequently joined, ensure that the data types across these tables match. Mismatched types can lead to implicit conversions, which degrade performance. Consistency is key.
CREATE TABLE orders ( order_id INT PRIMARY KEY, customer_id INT NOT NULL -- Consistent data type for joins );
6. Use Nullable Types Judiciously: While allowing NULL values can provide flexibility, overusing them can lead to complex queries and potential data integrity issues. Assess whether a column truly needs to allow NULLs, and consider using NOT NULL
wherever appropriate.
CREATE TABLE attendance ( event_id INT PRIMARY KEY, attendee_id INT NOT NULL -- Ensuring attendance is always recorded );
7. Regularly Review Your Data Types: As your application evolves, the data types you’ve initially chosen may no longer be the best fit. Regular reviews and audits of data types can help you adjust to changing requirements and improve performance.
Best practices for data type usage in SQL revolve around specificity, efficiency, and consistency. By applying these principles, you can create a robust database structure that optimally supports your application’s needs and enhances overall performance.
Handling Data Type Conversions and Compatibility
Handling data type conversions and compatibility in SQL is a critical aspect that many developers encounter as they design and maintain their databases. The need for conversion arises when the data types of two or more columns in a query do not match, which can lead to implicit or explicit conversions. Understanding how these conversions work can help in optimizing queries and preserving data integrity.
Implicit Conversions: SQL engines often perform implicit conversions automatically when two differing data types are used in a comparison or an operation. For instance, when an integer is compared with a float, the integer might be implicitly converted to a float. While this can simplify certain operations, it may introduce performance overheads and unexpected behaviors, especially if the underlying data types are not handled properly.
SELECT * FROM orders WHERE quantity = 10.0; -- Implicit conversion from INT to FLOAT
In the example above, if the quantity
column is defined as an integer and is compared to a float literal, the database engine must convert the integer to a float for the comparison, which may lead to performance degradation.
Explicit Conversions: Explicit conversions, on the other hand, are performed using SQL functions such as CAST
or CONVERT
. This gives developers control over how conversions are done and can be used to avoid unintended consequences of implicit conversions.
SELECT * FROM orders WHERE quantity = CAST(10 AS INT); -- Explicit conversion to INT
In this case, the explicit conversion ensures that the types being compared are the same, leading to clearer intent and potentially better performance.
Handling NULL Values: When dealing with data type conversions, special attention should be given to NULL values. Depending on the data type, implicit conversions involving NULL can lead to unexpected results, particularly when comparing NULL with non-NULL values. For example, any operation with NULL results in NULL, which might not be the intended behavior.
SELECT * FROM users WHERE last_login = NULL; -- Will not return any results
To properly handle NULL values in comparisons, use the IS NULL
clause instead:
SELECT * FROM users WHERE last_login IS NULL;
Data Type Compatibility: Compatibility between data types is essential when performing joins and unions. Mismatched types can lead to implicit conversions that degrade performance. Always strive for type consistency across your schema. For example, if you’re joining two tables based on a common ID, both should have the same data type:
CREATE TABLE orders ( order_id INT PRIMARY KEY, customer_id INT NOT NULL ); CREATE TABLE customers ( customer_id INT PRIMARY KEY, name VARCHAR(100) );
In this scenario, both customer_id
fields have the same type, ensuring efficient joins without unnecessary type conversions.
Best Practices for Data Type Conversions: To minimize the pitfalls of data type conversions, consider the following best practices:
- Always be explicit about the desired data type when performing operations or comparisons.
- Regularly review your schema for consistent data types, particularly in join conditions.
- Be cautious with NULLs and how they interact during conversions.
- Monitor performance and query plans to identify any implicit conversions that may be causing slowdowns.
By understanding and managing data type conversions effectively, you can create a more robust and efficient SQL environment that minimizes data integrity issues and enhances overall performance.
Source: https://www.plcourses.com/sql-and-data-types-best-practices/