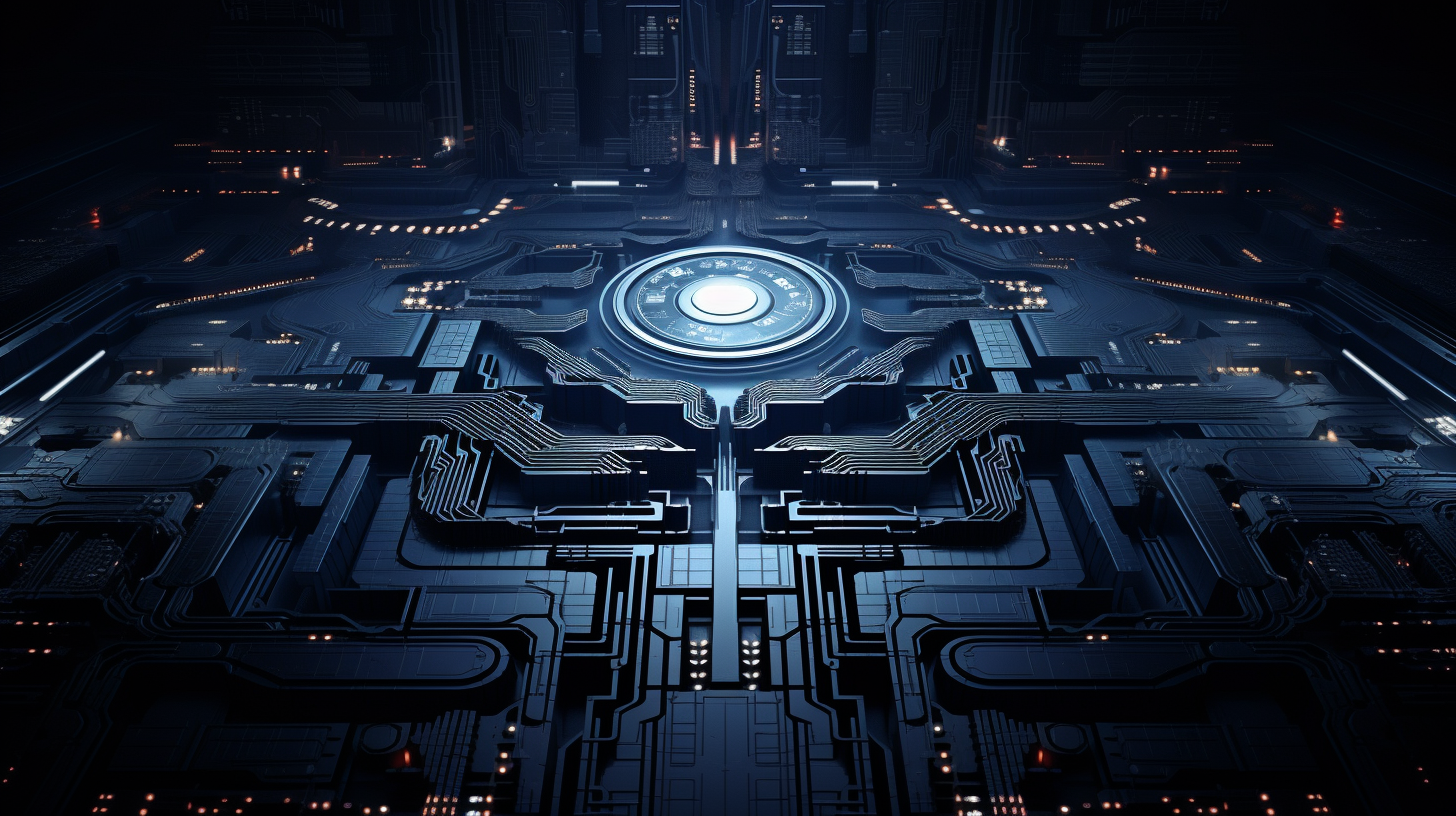
Data normalization is a fundamental concept in database design that seeks to organize data to reduce redundancy and improve data integrity. At its core, normalization involves structuring a relational database in such a way that dependencies are properly enforced, resulting in a system that’s robust, efficient, and easy to maintain.
To grasp the essence of data normalization, it is essential to understand the nature of relationships among data entities. In a well-normalized database, each piece of data is stored in one place, eliminating any possibility of duplication. This approach not only streamlines data management but also enhances the clarity of relationships among data items.
Normalization is typically achieved through a series of steps known as normal forms, each with its own set of rules and goals. These normal forms guide the database designer in achieving an optimal schema. The process begins with organizing data into tables, where each table represents a specific entity. That is where the first principles of normalization come into play.
To illustrate, think the following SQL table structure before normalization:
CREATE TABLE Orders ( OrderID INT PRIMARY KEY, CustomerName VARCHAR(100), ProductName VARCHAR(100), ProductPrice DECIMAL(10, 2), OrderDate DATE );
In this table, the product details will be duplicated for each order, leading to data redundancy. To normalize this structure, we would create separate tables for Customers and Products, establishing relationships between them. The normalized version might look like this:
CREATE TABLE Customers ( CustomerID INT PRIMARY KEY, CustomerName VARCHAR(100) ); CREATE TABLE Products ( ProductID INT PRIMARY KEY, ProductName VARCHAR(100), ProductPrice DECIMAL(10, 2) ); CREATE TABLE Orders ( OrderID INT PRIMARY KEY, CustomerID INT, ProductID INT, OrderDate DATE, FOREIGN KEY (CustomerID) REFERENCES Customers(CustomerID), FOREIGN KEY (ProductID) REFERENCES Products(ProductID) );
In this normalized schema, each order references the corresponding customer and product through their IDs. This arrangement not only saves space but also simplifies updates. If a product’s price changes, for instance, it only needs to be updated in one place—the Products table—instead of in every order record.
The journey towards normalization might seem tedious, but it leads to a significant payoff. Understanding how to effectively normalize data is important for any SQL developer aiming to create efficient, scalable, and maintainable database systems.
The Benefits of Data Normalization
The benefits of data normalization in database design stretch far beyond mere aesthetics. They encompass efficiency, integrity, and ease of management, all of which play an important role in the performance of applications relying on that data.
One of the most immediate advantages of normalization is the reduction of data redundancy. By ensuring that each data item is stored only once, databases can operate with greater efficiency. For example, if a customer moves to a new address, the system only needs to update that information in one location rather than multiple records, significantly reducing the chances of inconsistent data. This principle is vividly illustrated in the normalized schema where customer details are decoupled from order details.
Alongside redundancy reduction, normalization enhances data integrity. By enforcing relationships through foreign keys, the database maintains referential integrity, ensuring that relationships between data entities remain consistent. This means that if a product is deleted or changed, the database prevents orphaned records or inconsistencies. For instance, trying to delete a customer record that still has active orders would be disallowed without first handling those references, thus upholding the integrity of the dataset.
Performance is another area where normalization yields benefits, particularly in read-heavy environments. When data is organized in a way that minimizes duplication, it can lead to faster query performance. Well-structured queries can leverage indexes effectively, resulting in quicker data retrieval. A normalized database schema allows SQL queries to be more efficient, as they operate on smaller, more focused tables. For example, querying for all orders placed by a specific customer can be done succinctly:
SELECT o.OrderID, p.ProductName FROM Orders o JOIN Products p ON o.ProductID = p.ProductID WHERE o.CustomerID = 1;
Moreover, normalization supports clearer database design. When each table represents a distinct entity, the relationships between those entities become more apparent. This clarity aids in understanding the schema, paving the way for easier onboarding of new developers and smoother collaboration among teams. A developer can quickly grasp how entities relate to one another without navigating complex, intertwined structures.
Lastly, normalization facilitates easier maintenance and scalability. As businesses evolve, their data requirements often change. A normalized database schema is inherently more adaptable to changes. Adding new data elements or modifying existing relationships becomes a simpler task, minimizing the risk of errors and downtime. This adaptability is essential in today’s rapidly changing data landscape, where businesses must react to new opportunities and challenges effectively.
While the process of data normalization may seem complex and time-consuming, the long-term benefits it provides—reduction of redundancy, enhanced integrity, improved performance, clearer structure, and easier maintenance—make it an invaluable practice for any database designer. By embracing normalization, SQL developers can create systems that are not only more efficient but also more resilient to the challenges of future data management.
Key Normal Forms Explained
Understanding the various normal forms very important for any SQL developer aiming to design an optimized database schema. Normal forms are a series of criteria that a database schema must meet to achieve a certain level of normalization. Each normal form addresses specific types of redundancy and anomalies, guiding developers through the normalization process.
The first normal form (1NF) requires that all columns in a table contain atomic, indivisible values, and each entry in a column must be of the same data type. Furthermore, each row in a table must be unique, typically enforced through a primary key. Consider a scenario where we have a table capturing customer orders, with an issue of non-atomic values:
CREATE TABLE Orders ( OrderID INT PRIMARY KEY, CustomerName VARCHAR(100), Products VARCHAR(255) -- This allows multiple products in a single entry );
In this case, the Products column violates 1NF because it can contain multiple product names within a single entry. To adhere to 1NF, we need to separate these values into distinct rows:
CREATE TABLE Orders ( OrderID INT PRIMARY KEY, CustomerName VARCHAR(100), ProductName VARCHAR(100) );
Next, the second normal form (2NF) builds on the first by addressing partial dependencies. A table is in 2NF if it is in 1NF and all non-key attributes are fully functionally dependent on the primary key. This typically comes into play when a composite key is involved. For example, think the following table:
CREATE TABLE OrderDetails ( OrderID INT, ProductID INT, ProductName VARCHAR(100), -- That's partially dependent on ProductID PRIMARY KEY (OrderID, ProductID) );
In this case, ProductName is dependent only on ProductID, not on the combination of OrderID and ProductID. To achieve 2NF, we should separate the product information into its own table:
CREATE TABLE Products ( ProductID INT PRIMARY KEY, ProductName VARCHAR(100) ); CREATE TABLE OrderDetails ( OrderID INT, ProductID INT, PRIMARY KEY (OrderID, ProductID), FOREIGN KEY (ProductID) REFERENCES Products(ProductID) );
Moving on to the third normal form (3NF), a table is in 3NF if it is in 2NF and all the attributes are not only fully dependent on the primary key but also independent of each other. This means no transitive dependencies should exist. Think this example:
CREATE TABLE CustomerOrders ( OrderID INT PRIMARY KEY, CustomerID INT, CustomerName VARCHAR(100), -- Transitive dependency on CustomerID CustomerAddress VARCHAR(255) );
Here, CustomerName and CustomerAddress depend on CustomerID, not directly on OrderID. To comply with 3NF, we need to create a separate Customers table:
CREATE TABLE Customers ( CustomerID INT PRIMARY KEY, CustomerName VARCHAR(100), CustomerAddress VARCHAR(255) ); CREATE TABLE CustomerOrders ( OrderID INT PRIMARY KEY, CustomerID INT, FOREIGN KEY (CustomerID) REFERENCES Customers(CustomerID) );
Finally, the Boyce-Codd Normal Form (BCNF) is a stricter version of 3NF. A table is in BCNF if it is in 3NF and for every functional dependency, X → Y, X is a superkey. This addresses cases where there are overlapping candidate keys. For example:
CREATE TABLE CourseEnrollments ( StudentID INT, CourseID INT, InstructorName VARCHAR(100), PRIMARY KEY (StudentID, CourseID) );
In this case, InstructorName is dependent on CourseID, which is not a superkey. To satisfy BCNF, we should split the table into two:
CREATE TABLE Courses ( CourseID INT PRIMARY KEY, InstructorName VARCHAR(100) ); CREATE TABLE CourseEnrollments ( StudentID INT, CourseID INT, PRIMARY KEY (StudentID, CourseID), FOREIGN KEY (CourseID) REFERENCES Courses(CourseID) );
Each normal form has its own set of rules, and moving through these forms systematically enhances the structure of a database. Mastery of these concepts allows SQL developers to create schemas that are not only optimized for performance but also robust against data anomalies, ensuring a reliable environment for application development and data management.
SQL Techniques for Data Normalization
SQL techniques play a pivotal role in the normalization process, providing the tools necessary for database designers to implement and maintain a well-structured schema. These techniques encompass a variety of methods, including the use of primary keys, foreign keys, constraints, and normalization functions. Each of these elements contributes to the overarching goal of achieving a higher normal form in database design.
At the core of database normalization lies the concept of keys. A primary key uniquely identifies each record in a table, ensuring that no two rows are identical. That’s fundamental for maintaining data integrity. For instance, when creating a table for Customers, one might define a primary key as follows:
CREATE TABLE Customers ( CustomerID INT PRIMARY KEY, CustomerName VARCHAR(100), CustomerAddress VARCHAR(255) );
In this example, the CustomerID serves as the primary key, allowing each customer to be uniquely identified. The absence of duplicate records very important for enforcing the first normal form (1NF), which requires that every record be distinct.
Next, the foreign key establishes relationships between tables, linking them through a common attribute. That’s particularly useful in normalized databases where data is distributed across multiple tables. For example, in the Orders table, the CustomerID can be defined as a foreign key referencing the Customers table:
CREATE TABLE Orders ( OrderID INT PRIMARY KEY, CustomerID INT, OrderDate DATE, FOREIGN KEY (CustomerID) REFERENCES Customers(CustomerID) );
This foreign key constraint ensures referential integrity, meaning that any CustomerID in the Orders table must correspond to an existing CustomerID in the Customers table. This linkage directly supports the maintenance of normalized data, preventing orphaned records from existing.
Moreover, database constraints augment the normalization techniques by enforcing rules at the database level. Constraints such as UNIQUE, NOT NULL, and CHECK can be applied to ensure that data adheres to specific requirements. For example, if we want to prevent duplicate product names in the Products table, we could add a UNIQUE constraint:
CREATE TABLE Products ( ProductID INT PRIMARY KEY, ProductName VARCHAR(100) UNIQUE, ProductPrice DECIMAL(10, 2) );
This constraint prevents the insertion of multiple entries with the same ProductName, thereby enhancing data integrity and supporting the second normal form (2NF) by ensuring that non-key attributes are fully dependent on the primary key.
In addition to constraints, SQL provides a suite of aggregation functions and operators that can be utilized when querying normalized data. For instance, when analyzing sales data, one might want to retrieve total sales per product, which can be elegantly accomplished through SQL’s GROUP BY clause:
SELECT p.ProductName, SUM(o.Quantity) AS TotalSales FROM Orders o JOIN Products p ON o.ProductID = p.ProductID GROUP BY p.ProductName;
This query effectively aggregates data across related tables, demonstrating how normalized structures facilitate complex queries without redundancy.
Furthermore, the use of subqueries can also aid in normalization efforts, allowing for more dynamic data selection and manipulation. A common pattern might involve using a subquery to filter records based on aggregated results, like so:
SELECT * FROM Customers c WHERE c.CustomerID IN ( SELECT o.CustomerID FROM Orders o GROUP BY o.CustomerID HAVING COUNT(o.OrderID) > 5 );
This approach isolates customers who have made more than five orders, showcasing the power of normalized data in driving meaningful insights without compromising data integrity.
By strategically employing these SQL techniques, developers can achieve and maintain a normalized database structure that not only minimizes redundancy but also enhances the overall integrity, performance, and scalability of their applications. Mastery of these tools is essential for any SQL developer aspiring to optimize their database design and ensure efficient data management.
Common Pitfalls in Data Normalization and How to Avoid Them
While data normalization is a powerful tool for database design, it isn’t without its pitfalls. Understanding and avoiding these common missteps can enhance the effectiveness of normalization and lead to a more functional database schema.
One prevalent pitfall occurs during the initial design phase when developers rush to normalize without fully understanding the data requirements. This often results in over-normalization, where the database is broken down into excessive tables. While theoretically sound, this can lead to performance issues due to frequent joins in queries. For example, ponder an overly normalized structure where customer contact methods are separated into multiple tables:
CREATE TABLE Customers ( CustomerID INT PRIMARY KEY, CustomerName VARCHAR(100) ); CREATE TABLE CustomerEmails ( CustomerEmailID INT PRIMARY KEY, CustomerID INT, EmailAddress VARCHAR(100) ); CREATE TABLE CustomerPhones ( CustomerPhoneID INT PRIMARY KEY, CustomerID INT, PhoneNumber VARCHAR(15) );
This structure may complicate retrievals when querying customer contact methods, requiring multiple joins:
SELECT c.CustomerName, e.EmailAddress, p.PhoneNumber FROM Customers c LEFT JOIN CustomerEmails e ON c.CustomerID = e.CustomerID LEFT JOIN CustomerPhones p ON c.CustomerID = p.CustomerID;
Instead, a more balanced approach that combines email and phone into a single contact table would reduce complexity and improve query performance.
Another common pitfall is neglecting the implications of normalization on application performance. While normalization reduces redundancy, it can lead to increased query complexity and slower performance in write-heavy applications. In scenarios where high transaction rates are expected, denormalization—a controlled process of merging tables to reduce joins—may be more beneficial. For instance, in an e-commerce application where order processing speed is critical, a denormalized schema might look like this:
CREATE TABLE Orders ( OrderID INT PRIMARY KEY, CustomerName VARCHAR(100), EmailAddress VARCHAR(100), PhoneNumber VARCHAR(15), ProductName VARCHAR(100), ProductPrice DECIMAL(10, 2), OrderDate DATE );
This approach sacrifices some data integrity for speed, yet it is justifiable given the operational requirements of the application.
Additionally, developers sometimes fail to enforce referential integrity through foreign key constraints, leading to orphan records and inconsistent data. For instance, if products can be deleted without considering their presence in orders, the database is left in a state where orders reference non-existent products:
DELETE FROM Products WHERE ProductID = 1;
To avoid this, always implement foreign key constraints when establishing relationships. For example:
CREATE TABLE Orders ( OrderID INT PRIMARY KEY, ProductID INT, FOREIGN KEY (ProductID) REFERENCES Products(ProductID) ON DELETE CASCADE );
The ON DELETE CASCADE
option ensures that deleting a product will also remove all associated orders, thereby maintaining data integrity.
Lastly, one must be wary of the balance between normalization and usability. Over-normalizing can lead to a schema this is too complex for users to query effectively. Developers should strive for a schema that’s logical and intuitive, allowing end-users to perform operations without excessive complexity. This may involve providing views or stored procedures that abstract the underlying complexity of the normalized tables:
CREATE VIEW CustomerOrders AS SELECT c.CustomerName, o.OrderID, p.ProductName FROM Customers c JOIN Orders o ON c.CustomerID = o.CustomerID JOIN Products p ON o.ProductID = p.ProductID;
By carefully considering the implications of normalization and actively avoiding these pitfalls, database designers can build systems that are not only efficient and maintainable but also aligned with the practical needs of their applications.
Source: https://www.plcourses.com/using-sql-for-data-normalization/