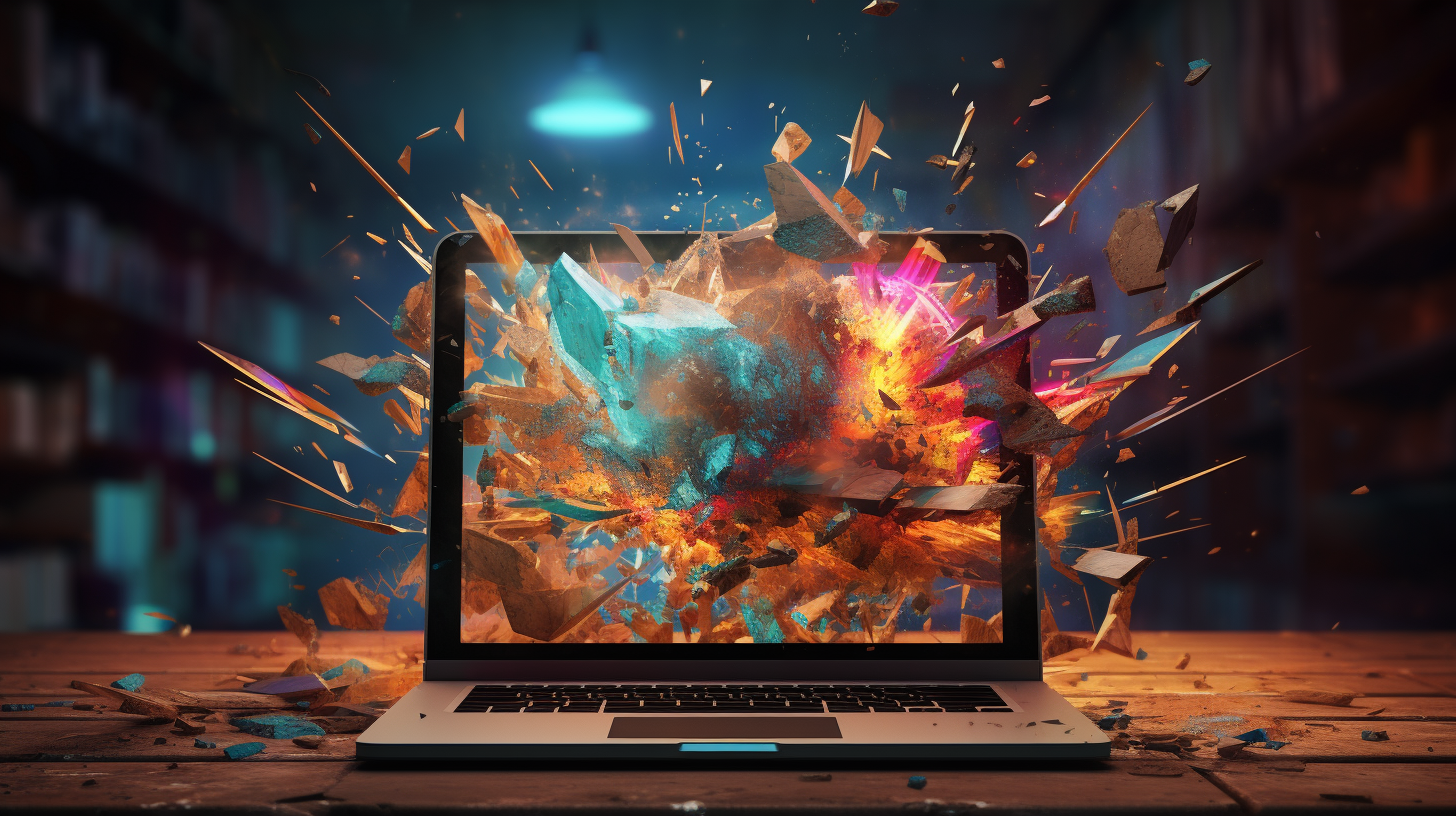
In the context of Java, exceptions are events that disrupt the normal flow of program execution. They signal that something unexpected has occurred, which the program must handle to avoid crashing. Java’s robust exception handling framework allows developers to manage these anomalies gracefully, ensuring that applications can respond to errors in a controlled manner.
At the core of Java’s exception handling mechanism is the concept of “throwing” and “catching” exceptions. An exception is an object that encapsulates an error or unexpected event, while the process of handling it involves writing code that can “catch” these exceptions and respond appropriately.
Java exceptions are divided into two main categories: checked and unchecked exceptions. Checked exceptions are those that are checked at compile-time, meaning the compiler requires the programmer to handle them using a try-catch block or declare them in the method signature with a throws
clause. Unchecked exceptions, on the other hand, are not checked at compile-time but can occur during runtime, typically due to programming errors such as null pointer dereferencing or array index out of bounds.
Here’s a simple example demonstrating how to throw and catch an exception:
public class ExceptionExample { public static void main(String[] args) { try { int result = divide(10, 0); System.out.println("Result: " + result); } catch (ArithmeticException e) { System.out.println("Error: " + e.getMessage()); } } public static int divide(int a, int b) { return a / b; // This will throw ArithmeticException if b is 0 } }
In this example, the divide
method attempts to divide by zero, which throws an ArithmeticException
. The exception is caught in the main
method’s try-catch block, allowing the program to handle the error gracefully instead of terminating unexpectedly.
To summarize, mastering Java exception handling very important for building robust applications. By understanding how to throw and catch exceptions effectively, developers can create programs that are resilient to unexpected issues, providing a better experience for users and reducing the likelihood of abrupt failures.
Types of Exceptions in Java
Java provides a comprehensive framework for categorizing exceptions, which enables developers to implement precise error handling strategies. As mentioned, exceptions are classified primarily into two categories: checked and unchecked exceptions. This distinction plays a significant role in how developers write and maintain their code.
Checked Exceptions
Checked exceptions are subclasses of the Exception class, excluding RuntimeException and its subclasses. These exceptions are checked at compile time, meaning the Java compiler enforces a requirement for the code to either handle these exceptions with a try-catch block or declare them in the method signature using the throws
keyword.
For instance, if you are working with file operations, the IOException
is a typical checked exception that you must manage. Here’s how it can be handled:
import java.io.BufferedReader; import java.io.FileReader; import java.io.IOException; public class FileReadExample { public static void main(String[] args) { BufferedReader reader = null; try { reader = new BufferedReader(new FileReader("example.txt")); String line = null; while ((line = reader.readLine()) != null) { System.out.println(line); } } catch (IOException e) { System.out.println("Error reading file: " + e.getMessage()); } finally { try { if (reader != null) { reader.close(); } } catch (IOException e) { System.out.println("Error closing the file: " + e.getMessage()); } } } }
In this example, the FileReader
can throw an IOException
if the file does not exist or cannot be opened. The catch
block captures that exception, and the finally
block ensures that resources are properly closed, demonstrating responsible resource management.
Unchecked Exceptions
Unchecked exceptions are derived from RuntimeException
and include errors that are often the result of programming mistakes, such as accessing an array with an invalid index or dereferencing a null object. These exceptions are not checked at compile time, which means programmers are not required to handle them explicitly.
For example, consider the following code that can result in a NullPointerException
:
public class NullPointerExample { public static void main(String[] args) { String str = null; System.out.println(str.length()); // This will throw NullPointerException } }
In this case, attempting to access the length
property of a null reference leads to a NullPointerException
. While developers can certainly handle unchecked exceptions, they are often indicative of bugs in the code that should be fixed rather than handled.
Error Types
In addition to these, there are errors that extend from the Error
class, which are not typically subject to application-level handling. Errors represent serious issues that a reasonable application should not try to catch, such as StackOverflowError
or OutOfMemoryError
. These errors indicate a fundamental problem with the Java Virtual Machine (JVM) or the environment in which the application is running.
By understanding these different types of exceptions and their characteristics, developers can create more robust error handling mechanisms, ensuring that their applications can manage both expected and unexpected issues. This knowledge not only helps in writing cleaner code but also in maintaining applications over time as they evolve and interact with various external systems.
Using Try-Catch Blocks Effectively
Using try-catch blocks effectively is a cornerstone of Java’s exception handling paradigm. A well-structured try-catch block allows a programmer to anticipate potential errors and respond in a meaningful way, preserving the integrity of the application and enhancing user experience.
A try-catch block works by enclosing potentially error-prone code within a try
block. If an exception occurs, control is transferred to the corresponding catch
block, where the exception can be handled. This mechanism prevents the application from crashing and allows for tailored responses to different types of exceptions.
To illustrate, consider the following example, which demonstrates the use of multiple catch blocks to handle different exceptions:
public class MultiCatchExample { public static void main(String[] args) { String[] numbers = {"10", "2", "0", "invalid"}; for (String num : numbers) { try { int result = 100 / Integer.parseInt(num); System.out.println("Result: " + result); } catch (ArithmeticException e) { System.out.println("Cannot divide by zero."); } catch (NumberFormatException e) { System.out.println("Invalid number format: " + num); } } } }
In this example, we are iterating over an array of strings representing numbers. The try
block attempts to parse each string to an integer and perform a division operation. If a division by zero occurs, an ArithmeticException
is caught, while a NumberFormatException
is handled if the string does not represent a valid integer. This approach allows for graceful degradation of functionality without crashing the application.
Another important aspect of using try-catch blocks effectively is the use of nested try-catch blocks. While it can lead to more complex code, it allows for specific handling of exceptions that might occur at different levels of execution. Below is an example demonstrating this technique:
public class NestedTryCatchExample { public static void main(String[] args) { try { String[] data = {"1", "2", "three"}; for (String item : data) { try { int number = Integer.parseInt(item); System.out.println("Number: " + number); } catch (NumberFormatException e) { System.out.println("Error: Unable to parse '" + item + "' to an integer."); } } } catch (Exception e) { System.out.println("An unexpected error occurred: " + e.getMessage()); } } }
In this case, the outer try
block serves as a catch-all for any unforeseen exceptions that may propagate from the inner block, while the inner block specifically addresses the possibility of parsing errors.
While using try-catch blocks, it’s also essential to understand the importance of clean and informative error messages. Instead of vague messages, providing specific context about what went wrong can greatly aid in debugging. Ponder the following example:
public class InformativeErrorExample { public static void main(String[] args) { try { String input = null; System.out.println(input.length()); // This will throw NullPointerException } catch (NullPointerException e) { System.out.println("Error: Attempted to call length on a null reference."); } } }
Lastly, it’s worth mentioning that overusing try-catch blocks can lead to code this is difficult to read and maintain. It’s crucial to use them judiciously, wrapping only the code that is likely to throw exceptions, while keeping the rest of the logic clear and simpler.
Using try-catch blocks effectively requires a thoughtful approach to handling exceptions that balances specificity with clarity. Properly structured error handling not only prevents application crashes but also enriches the overall software experience by providing meaningful feedback and maintaining control over program execution.
Finally and Try-With-Resources
When dealing with exceptions in Java, the use of the finally block is pivotal for ensuring that critical cleanup code is executed, regardless of whether an exception was thrown or caught. The finally block is an integral part of the try-catch structure that guarantees the execution of its code segment, thus enabling developers to release resources, close connections, or perform other necessary cleanup tasks. That is particularly crucial in applications that manage limited resources, such as file streams or network connections, where failing to release resources can lead to memory leaks or other performance issues.
Here’s a simpler example illustrating the use of a finally block:
public class FinallyExample { public static void main(String[] args) { try { int result = 10 / 0; // This throws ArithmeticException } catch (ArithmeticException e) { System.out.println("Caught an exception: " + e.getMessage()); } finally { System.out.println("This block always executes."); } } }
In this example, even though an ArithmeticException is thrown when attempting to divide by zero, the message from the finally block is still printed. This guarantees that any necessary final operations are carried out, irrespective of whether an exception occurs.
While the finally block is a powerful tool, Java also introduces the try-with-resources statement, which simplifies resource management by automatically closing resources when they’re no longer needed. This feature was introduced in Java 7 and is especially useful when working with AutoCloseable resources, such as streams, files, and database connections. The try-with-resources statement manages the closing of these resources automatically, removing the potential for resource leaks.
Here’s how a try-with-resources statement looks in practice:
import java.io.BufferedReader; import java.io.FileReader; import java.io.IOException; public class TryWithResourcesExample { public static void main(String[] args) { try (BufferedReader reader = new BufferedReader(new FileReader("example.txt"))) { String line; while ((line = reader.readLine()) != null) { System.out.println(line); } } catch (IOException e) { System.out.println("Error reading file: " + e.getMessage()); } } }
In this example, the BufferedReader is declared inside the parentheses of the try statement. When the try block is exited—whether through normal execution or due to an exception—the BufferedReader is automatically closed. This feature greatly reduces boilerplate code and enhances the readability of programs by ensuring that resource management is handled cleanly and safely.
It’s worth noting that the try-with-resources statement can handle multiple resources, providing a clean and concise way to manage several resources without extensive try-finally blocks:
import java.io.BufferedReader; import java.io.FileReader; import java.io.IOException; public class MultiResourceExample { public static void main(String[] args) { try (BufferedReader reader = new BufferedReader(new FileReader("example.txt")); FileWriter writer = new FileWriter("output.txt")) { String line; while ((line = reader.readLine()) != null) { writer.write(line); writer.write(System.lineSeparator()); } } catch (IOException e) { System.out.println("Error: " + e.getMessage()); } } }
In this code, both the BufferedReader and FileWriter are declared within the same try-with-resources statement. Both resources are automatically closed when the try block completes, either normally or due to an exception, ensuring that all resources are properly managed without requiring additional code.
Using the finally block and the try-with-resources statement effectively leads to cleaner, more maintainable code, while providing robust mechanisms for resource management and cleanup in Java applications.
Best Practices for Exception Handling in Java
When it comes to exception handling in Java, adhering to best practices is essential for developing resilient and maintainable applications. These practices not only aid in managing exceptions effectively but also enhance the overall quality of the codebase, making it more understandable for both current and future developers.
1. Catch Specific Exceptions
Always aim to catch the most specific exception types first before catching more general ones. This practice allows for tailored handling of different error conditions, resulting in clearer and more effective error management. For example:
public class SpecificExceptionExample { public static void main(String[] args) { try { int result = 100 / 0; } catch (ArithmeticException e) { System.out.println("Arithmetic error: " + e.getMessage()); } catch (Exception e) { System.out.println("An error occurred: " + e.getMessage()); } } }
In this example, the ArithmeticException is caught specifically, allowing for a distinct handling mechanism tailored to arithmetic errors.
2. Avoid Empty Catch Blocks
Never leave catch blocks empty. Doing so can lead to silent failures, making debugging incredibly difficult. Always provide at least logging or some form of notification to indicate that an exception has occurred. Here’s an example of a poor practice:
public class EmptyCatchExample { public static void main(String[] args) { try { String s = null; System.out.println(s.length()); } catch (NullPointerException e) { // Do nothing } } }
Instead, handle the exception appropriately:
public class NotEmptyCatchExample { public static void main(String[] args) { try { String s = null; System.out.println(s.length()); } catch (NullPointerException e) { System.out.println("Caught a NullPointerException: " + e.getMessage()); } } }
3. Use Finally or Try-With-Resources for Cleanup
Whenever you open resources such as files or database connections, ensure that you release them promptly. Use finally blocks or the try-with-resources statement to guarantee resources are closed. This prevents memory leaks and ensures your application runs smoothly:
import java.io.BufferedReader; import java.io.FileReader; import java.io.IOException; public class CleanUpExample { public static void main(String[] args) { try (BufferedReader reader = new BufferedReader(new FileReader("file.txt"))) { String line; while ((line = reader.readLine()) != null) { System.out.println(line); } } catch (IOException e) { System.out.println("Error reading file: " + e.getMessage()); } } }
4. Document Exception Handling
Documenting exception handling logic is as crucial as implementing it. This includes explaining why certain exceptions are caught in specific ways and what actions are taken in response. Use comments and documentation to clarify your intent, which aids in maintenance and onboarding new developers:
public class DocumentedExample { public static void main(String[] args) { try { Integer.parseInt("invalid"); } catch (NumberFormatException e) { // Catching NumberFormatException because parsing an invalid string is expected System.out.println("Invalid number format: " + e.getMessage()); } } }
5. Ponder Custom Exceptions
In cases where standard exceptions do not suffice, consider creating custom exception classes. This allows for more granular control over exception handling and can also encapsulate application-specific error conditions. Here’s a simple custom exception class:
public class CustomException extends Exception { public CustomException(String message) { super(message); } } public class CustomExceptionExample { public static void main(String[] args) { try { throw new CustomException("Something went wrong!"); } catch (CustomException e) { System.out.println("Caught custom exception: " + e.getMessage()); } } }
By implementing these best practices, developers can create more reliable, maintainable, and uncomplicated to manage Java applications that gracefully handle exceptions and provide meaningful feedback, improving the overall robustness of the software.
Source: https://www.plcourses.com/java-exception-handling-try-catch/