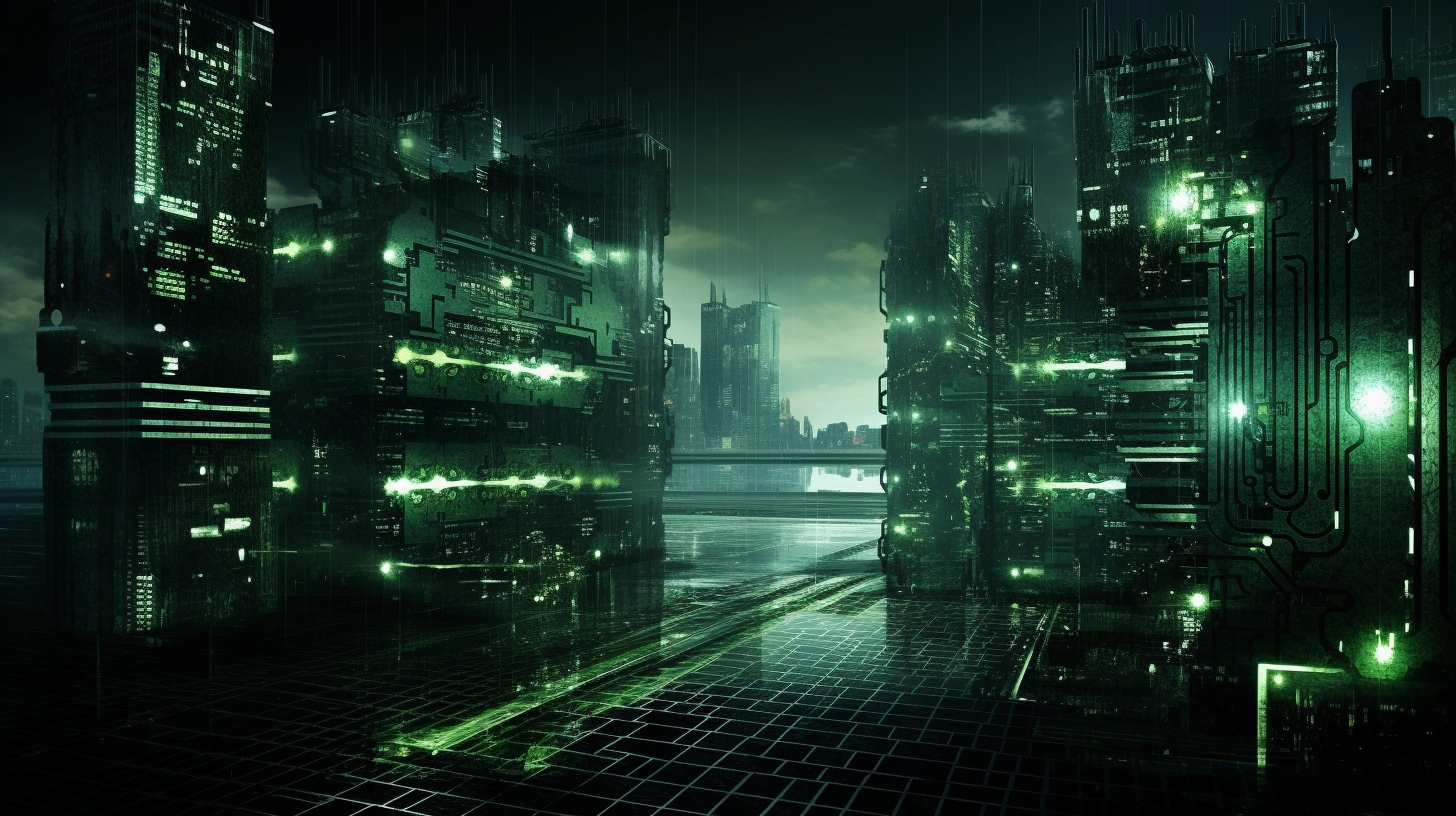
Monitoring database performance especially important for ensuring the smooth operation of any application. SQL queries can help you track various performance metrics that indicate the health of your database. Below are some essential SQL queries that can be utilized to monitor database performance effectively.
To start, let’s look at how to check for active sessions in the database. This can reveal whether your database is under heavy load or if there are any performance bottlenecks due to too many concurrent users.
SELECT COUNT(*) AS ActiveSessions FROM sys.dm_exec_sessions WHERE status = 'active';
Next, it is important to monitor the wait statistics, as they provide insights into the types of waits that are occurring in your system. This information can help identify potential performance issues.
SELECT wait_type, SUM(wait_time_ms) AS TotalWaitTime, SUM(wait_time_ms) / SUM(wait_count) AS AvgWaitTime, SUM(wait_count) AS WaitCount FROM sys.dm_os_wait_stats GROUP BY wait_type ORDER BY TotalWaitTime DESC;
Another useful query is to check the I/O statistics. This allows you to monitor how much data is being read from and written to the database, which is critical for performance tuning.
SELECT DB_NAME(database_id) AS DatabaseName, SUM(num_of_reads) AS TotalReads, SUM(num_of_writes) AS TotalWrites, SUM(size_on_disk_bytes) AS SizeOnDisk FROM sys.dm_io_virtual_file_stats(NULL, NULL) GROUP BY database_id;
Additionally, monitoring query performance through execution statistics can provide insights into slow-running queries that may need optimization. The following query retrieves information about queries that have executed in the last few minutes.
SELECT TOP 10 qs.total_elapsed_time / 1000 AS TotalElapsedTimeMS, qs.execution_count AS ExecutionCount, qs.total_worker_time / 1000 AS TotalCPUTimeMS, qs.total_logical_reads AS TotalLogicalReads, qs.total_physical_reads AS TotalPhysicalReads, qs.total_logical_writes AS TotalLogicalWrites, SUBSTRING(qt.text, (qs.statement_start_offset/2) + 1, ((CASE qs.statement_end_offset WHEN -1 THEN DATALENGTH(qt.text) ELSE qs.statement_end_offset END - qs.statement_start_offset)/2) + 1) AS QueryText FROM sys.dm_exec_query_stats AS qs CROSS APPLY sys.dm_exec_sql_text(qs.sql_handle) AS qt ORDER BY TotalElapsedTimeMS DESC;
Finally, it’s essential to keep an eye on the database transaction log. Monitoring the size and growth of the transaction log can help prevent issues related to disk space.
SELECT DB_NAME(database_id) AS DatabaseName, name AS LogFileName, size * 8 / 1024 AS SizeMB, growth * 8 / 1024 AS GrowthMB FROM sys.master_files WHERE type_desc = 'LOG';
These queries can serve as a fundamental toolkit for monitoring the performance of your database, allowing you to take necessary actions before performance issues escalate into significant problems.
Common Indicators of Database Health
Within the scope of database health, identifying common indicators is key to preemptively addressing potential issues. Various metrics can provide insight into the robustness and efficiency of your database. Understanding these indicators will arm you with the knowledge needed to maintain optimal performance.
One of the primary indicators of database health is the rate of blocked processes. Blocking occurs when one session holds a lock on a resource that another session is trying to access. Monitoring this can help you identify contention points within your system. You can use the following SQL query to check for currently blocked sessions:
SELECT blocking_session_id AS BlockingSessionID, session_id AS BlockedSessionID, wait_type, wait_time, wait_resource FROM sys.dm_exec_requests WHERE blocking_session_id 0;
Next, it is crucial to monitor the health of your indexes. Fragmented indexes can lead to inefficient query performance, so keeping track of their fragmentation levels is vital. Use the following query to assess the fragmentation percentage of indexes in your database:
SELECT dbschemas.name AS SchemaName, dbtables.name AS TableName, dbindexes.name AS IndexName, indexstats.avg_fragmentation_in_percent FROM sys.dm_db_index_physical_stats(DB_ID(), NULL, NULL, NULL, NULL) AS indexstats JOIN sys.tables AS dbtables ON indexstats.object_id = dbtables.object_id JOIN sys.schemas AS dbschemas ON dbtables.schema_id = dbschemas.schema_id JOIN sys.indexes AS dbindexes ON indexstats.object_id = dbindexes.object_id AND indexstats.index_id = dbindexes.index_id WHERE indexstats.avg_fragmentation_in_percent > 30;
Another important aspect to monitor is the memory usage of your database. Memory pressure can severely impact performance if not properly managed. The following query allows you to assess memory allocations for the SQL Server:
SELECT type AS MemoryType, SUM(allocated_memory_kb) / 1024 AS AllocatedMemoryMB FROM sys.dm_os_memory_clerks GROUP BY type ORDER BY AllocatedMemoryMB DESC;
Moreover, tracking the number of deadlocks occurring within your database is essential for maintaining performance. High deadlock rates can signify deeper issues in query design or transaction management. You can identify deadlock occurrences using this query:
SELECT deadlock_graph FROM sys.dm_tran_deadlock_history;
Lastly, examining the overall health of the database files is also critical. Monitoring the status and availability of database files can prevent unexpected outages. You can run this query to check on the status of your database files:
SELECT name AS FileName, state_desc AS FileState, size * 8 / 1024 AS SizeMB FROM sys.master_files WHERE database_id = DB_ID('YourDatabaseName');
Monitoring these common indicators allows database administrators to gain valuable insights into the performance and health of their databases. By regularly assessing these metrics, you can proactively mitigate risks and ensure your database remains robust and efficient.
Automating Health Check Procedures with SQL
Automating health check procedures with SQL can significantly enhance your ability to maintain database performance and reliability. By setting up automated routines, you can ensure that you consistently monitor key metrics without the need for manual intervention. This not only saves time but also helps detect issues before they escalate into critical problems.
To begin automating health checks, you can leverage SQL Server Agent jobs. This built-in feature allows you to schedule and execute SQL scripts at defined intervals. First, create a SQL script that embodies the health checks you want to automate. Here’s a simple example that combines various checks into one script:
-- Automating health checks BEGIN TRY -- Check for blocked sessions SELECT blocking_session_id AS BlockingSessionID, session_id AS BlockedSessionID, wait_type, wait_time, wait_resource FROM sys.dm_exec_requests WHERE blocking_session_id != 0; -- Check for index fragmentation SELECT dbschemas.name AS SchemaName, dbtables.name AS TableName, dbindexes.name AS IndexName, indexstats.avg_fragmentation_in_percent FROM sys.dm_db_index_physical_stats(DB_ID(), NULL, NULL, NULL, NULL) AS indexstats JOIN sys.tables AS dbtables ON indexstats.object_id = dbtables.object_id JOIN sys.schemas AS dbschemas ON dbtables.schema_id = dbschemas.schema_id JOIN sys.indexes AS dbindexes ON indexstats.object_id = dbindexes.object_id AND indexstats.index_id = dbindexes.index_id WHERE indexstats.avg_fragmentation_in_percent > 30; -- Other health checks can be added here END TRY BEGIN CATCH PRINT 'An error occurred while executing the health checks.'; PRINT ERROR_MESSAGE(); END CATCH
Once you have your SQL script ready, the next step is to create a new SQL Server Agent job. This job will execute your script at regular intervals. Here are the steps to create a SQL Server Agent job:
- Open SQL Server Management Studio (SSMS).
- Connect to your SQL Server instance.
- Expand the SQL Server Agent node in Object Explorer.
- Right-click on the “Jobs” folder and select “New Job…”.
- In the “New Job” window, give your job a name and description.
- Navigate to the “Steps” page and click on “New…”.
- In the “New Job Step” window, provide a step name, select “Transact-SQL script (T-SQL)” as the type, and paste your health check script into the command box.
- Go to the “Schedules” page, click on “New…”, and set the frequency and time for your job.
- Click “OK” to save the job.
In addition to running health checks, you can enhance your automation by integrating alerts and notifications. SQL Server Agent can send email alerts based on the results of your health checks. For instance, if your script detects a high level of index fragmentation or blocked sessions, it can trigger an alert to notify the database administrator.
To set up alerts, you will need to configure Database Mail in SQL Server, and then create an alert that corresponds to specific conditions. You can use the following SQL to create a sample alert:
-- Create a SQL Server alert EXEC msdb.dbo.sp_add_alert @name = N'High Index Fragmentation Alert', @message_id = 0, @severity = 0, @enabled = 1, @delay_between_responses = 0, @notification_message = N'High index fragmentation detected. Please review.', @database_name = N'YourDatabaseName', @include_event_description = 1;
By automating your database health checks and integrating alerting mechanisms, you can create a robust monitoring solution that proactively addresses issues and maintains database performance. This approach embodies the principles of efficiency and vigilance, so that you can focus on other vital areas of database management while ensuring that health checks are consistently performed.
Integrating Alerts and Notifications
Integrating alerts and notifications into your SQL health check procedures is essential for ensuring that you are immediately informed of potential problems before they escalate into critical issues. By using SQL Server Agent’s alerting capabilities, you can set up a system that automatically notifies you of significant events or thresholds being reached, which will allow you to take prompt action. This proactive approach can save time, reduce downtime, and ultimately enhance the reliability of your database systems.
To begin with, it’s important to configure Database Mail in SQL Server if you haven’t done so already. This service allows SQL Server to send emails. You’ll need to set up a mail profile and account that SQL Server can use to send notifications. Once Database Mail is configured, you can create alerts based on various conditions detected during your automated health checks.
Alerts can be created to notify you of specific performance metrics, such as when the number of deadlocks exceeds a predefined limit. You can create an alert for this condition using the following SQL code:
EXEC msdb.dbo.sp_add_alert @name = N'Deadlock Alert', @message_id = 0, @severity = 0, @enabled = 1, @delay_between_responses = 0, @notification_message = N'A deadlock has occurred. Please investigate.', @database_name = N'YourDatabaseName', @include_event_description = 1;
In addition to deadlocks, you might want to set up alerts for high index fragmentation levels or excessive blocking sessions. For instance, you could create an alert that triggers when the average fragmentation exceeds a certain threshold (e.g., 30% fragmentation). The alert configuration would look something like this:
EXEC msdb.dbo.sp_add_alert @name = N'High Index Fragmentation Alert', @message_id = 0, @severity = 0, @enabled = 1, @delay_between_responses = 0, @notification_message = N'High index fragmentation detected. Please review.', @database_name = N'YourDatabaseName', @include_event_description = 1;
Once you’ve created these alerts, you need to specify how you want to receive notifications when they’re triggered. You can do this by defining operators in SQL Server. An operator is simply a person or group who will receive alerts. To create an operator, use the following SQL command:
EXEC msdb.dbo.sp_add_operator @name = N'DBA Operator', @enabled = 1, @email_address = N'[email protected]', @pager_address = NULL;
After creating the operator, you can link the alerts you created to this operator, ensuring that notifications are sent directly to the responsible individual or team.
SQL Server Agent can also send notifications based on job outcomes. For example, if a job responsible for health checks detects an issue, you can configure it to notify the operator. This can be accomplished by modifying the job properties to include an alert notification for job failures. This approach provides an additional layer of security, ensuring that if something goes wrong during the execution of your health checks, the responsible parties are informed immediately.
By implementing a comprehensive alerting and notification system, you can create a robust safety net that keeps you informed about your database’s health. This not only enhances your ability to respond quickly to issues but also helps cultivate a culture of proactive maintenance, ultimately leading to improved database performance and reliability.
Best Practices for Regular Database Maintenance
Regular database maintenance is a cornerstone of ensuring optimal performance and reliability. By adhering to best practices, database administrators can proactively mitigate issues, enhance performance, and extend the lifespan of their database systems. Here are some key practices that should be part of any database maintenance strategy.
First and foremost, regularly backing up your databases is essential. Backups protect your data against loss due to hardware failures, natural disasters, or accidental deletions. For SQL Server, this can be easily set up with a scheduled job. Here’s an example of a T-SQL command to perform a full backup of a database:
BACKUP DATABASE YourDatabaseName TO DISK = 'C:BackupsYourDatabaseName_Full.bak' WITH FORMAT, MEDIANAME = 'SQLServerBackups', NAME = 'Full Backup of YourDatabaseName';
In addition to backups, regularly updating statistics very important for query optimization. SQL Server uses statistics to determine the most efficient way to execute a query. Outdated statistics can lead to suboptimal query plans. You can update statistics for a specific table using the following command:
UPDATE STATISTICS YourTableName;
Another best practice is to manage index fragmentation. Over time, indexes can become fragmented due to data modifications (inserts, updates, and deletes), which can severely impact query performance. Regular maintenance of indexes involves rebuilding or reorganizing them based on their fragmentation levels. Use the following queries to check the fragmentation levels and then decide on the appropriate action:
SELECT dbschemas.name AS SchemaName, dbtables.name AS TableName, dbindexes.name AS IndexName, indexstats.avg_fragmentation_in_percent FROM sys.dm_db_index_physical_stats(DB_ID(), NULL, NULL, NULL, NULL) AS indexstats JOIN sys.tables AS dbtables ON indexstats.object_id = dbtables.object_id JOIN sys.schemas AS dbschemas ON dbtables.schema_id = dbschemas.schema_id JOIN sys.indexes AS dbindexes ON indexstats.object_id = dbindexes.object_id AND indexstats.index_id = dbindexes.index_id WHERE indexstats.avg_fragmentation_in_percent > 30;
If fragmentation exceeds a threshold (commonly 30%), consider rebuilding the index:
ALTER INDEX YourIndexName ON YourTableName REBUILD;
Or, for lower fragmentation, you can reorganize the index:
ALTER INDEX YourIndexName ON YourTableName REORGANIZE;
Monitoring and managing database growth is also critical. Databases can grow unexpectedly, leading to potential issues with disk space. You should routinely check the size of your databases and files:
SELECT name AS DatabaseName, size * 8 / 1024 AS SizeMB FROM sys.master_files;
Additionally, implementing a proper maintenance plan that includes regular integrity checks using the DBCC CHECKDB command ensures that your database remains in a healthy state. Running this command periodically helps identify any corruption in database files:
DBCC CHECKDB ('YourDatabaseName');
Lastly, documentation and consistent monitoring are indispensable. Keeping a log of maintenance activities, performance metrics, and configurations allows for better decision-making and troubleshooting. You can automate the logging of maintenance activities through SQL Agent jobs, ensuring that you have a historical reference for performance trends and maintenance outcomes.
By incorporating these best practices into your regular database maintenance routine, you can enhance performance, extend the longevity of your databases, and significantly reduce the risk of downtime and data loss. Regular maintenance is not merely a task but a vital aspect of diligent database stewardship.
Source: https://www.plcourses.com/sql-for-automated-database-health-checks/