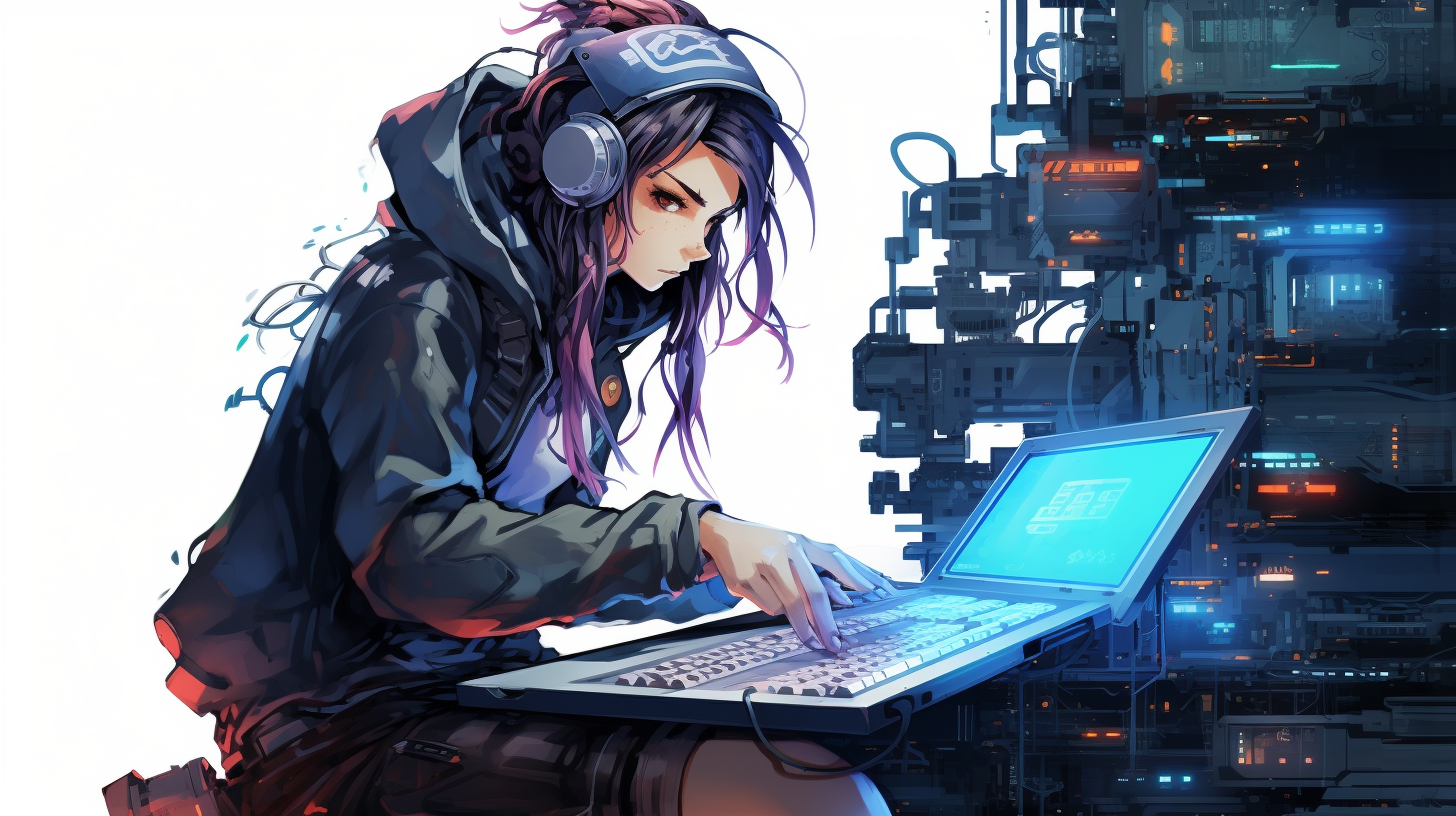
JavaScript Object Notation, or JSON, is a lightweight data interchange format this is easy for humans to read and write, and easy for machines to parse and generate. At its core, JSON is built around two primary structures: objects and arrays.
A JSON object is an unordered set of key/value pairs. The keys are strings, and the values can be strings, numbers, objects, arrays, booleans, or null. This flexibility allows for a rich data representation. For example:
{ "name": "Vatslav Kowalsky", "age": 30, "isStudent": false, "courses": ["Math", "Science"], "address": { "street": "123 Main St", "city": "Anytown" } }
In the example above, we have a JSON object that represents a person. The object contains several key/value pairs: the person’s name, age, whether they’re a student, a list of courses, and an address object containing its own key/value pairs.
On the other hand, a JSON array is an ordered collection of values. An array can contain objects, and those objects can contain any type of data as well. Here’s a simple example of a JSON array:
[ { "id": 1, "title": "Introduction to Java", "author": "Jane Smith" }, { "id": 2, "title": "Advanced Java", "author": "Nick Johnson" } ]
This array contains two objects, each representing a book with an id, title, and author. The structure of JSON allows for nested arrays and objects, enabling complex data structures.
It’s important to note that while JSON is primarily used as a data interchange format, its simplicity and readability make it a popular choice for configuration files and data storage as well. Understanding the fundamental structures of JSON is important for effective parsing and serialization in Java.
When interfacing with JSON in Java, you will frequently convert between JSON strings and Java objects. This conversion relies on the structure of JSON to determine how to map various data types to corresponding Java types, ensuring that the integrity of the data is maintained throughout the process.
Java Libraries for JSON Parsing
To parse JSON data in Java, there are several robust libraries available, each with its own strengths and weaknesses. Some of the most widely used libraries include Jackson, Gson, and org.json. Each of these libraries provides a set of tools and classes to facilitate the reading and writing of JSON data in a way that integrates seamlessly with Java objects.
Jackson is a powerful library that is often the go-to choice for developers needing to work with JSON. It is known for its speed and ease of use. Jackson provides a simpler API to convert Java objects to JSON and vice versa. Here’s a simple example of how to use Jackson for parsing JSON:
import com.fasterxml.jackson.databind.ObjectMapper; public class JacksonExample { public static void main(String[] args) { String jsonString = "{ "name": "Neil Hamilton", "age": 30 }"; ObjectMapper objectMapper = new ObjectMapper(); try { Person person = objectMapper.readValue(jsonString, Person.class); System.out.println(person.getName() + " is " + person.getAge() + " years old."); } catch (Exception e) { e.printStackTrace(); } } } class Person { private String name; private int age; // Getters and Setters public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } }
In this example, we define a simple `Person` class with fields for name and age. The `ObjectMapper` from the Jackson library is used to read a JSON string and convert it into a `Person` object.
Gson, developed by Google, is another popular library for converting Java objects to and from JSON. It is known for its simplicity and ease of integration. Here’s how you can use Gson to parse JSON:
import com.google.gson.Gson; public class GsonExample { public static void main(String[] args) { String jsonString = "{"name":"Jane Doe","age":25}"; Gson gson = new Gson(); Person person = gson.fromJson(jsonString, Person.class); System.out.println(person.getName() + " is " + person.getAge() + " years old."); } }
As seen above, Gson provides a very clean and concise syntax for parsing JSON. By using the `fromJson` method, we can easily convert a JSON string into a Java object.
Lastly, the org.json library is a lightweight library that can also be used for parsing and creating JSON data in Java. It provides a simple API for manipulating JSON objects and arrays. Here’s an example:
import org.json.JSONObject; public class JsonOrgExample { public static void main(String[] args) { String jsonString = "{"name":"Alice","age":28}"; JSONObject jsonObject = new JSONObject(jsonString); String name = jsonObject.getString("name"); int age = jsonObject.getInt("age"); System.out.println(name + " is " + age + " years old."); } }
With the `org.json` library, we can create a `JSONObject` directly from a JSON string, allowing us to easily access individual fields using methods like `getString` and `getInt`.
Each of these libraries serves a distinct purpose and can be chosen based on specific project requirements, such as the need for performance, simplicity, or additional features. Understanding how to leverage these libraries effectively will enhance your ability to handle JSON data in Java applications.
Serializing Java Objects to JSON
Serialization is the process of converting a Java object into a JSON string representation, allowing it to be easily transmitted or stored. This transformation helps maintain the structure, types, and hierarchy of data while providing a human-readable format. The ability to serialize Java objects to JSON especially important when developing applications that require data interchange with web services or APIs.
To serialize a Java object to JSON, you can utilize the same libraries mentioned earlier: Jackson, Gson, and org.json. Each library offers its own methods for handling serialization, making the process efficient and simpler.
Starting with Jackson, it provides a simple and powerful way to convert Java objects into their JSON representation using the `ObjectMapper` class. Below is an example of serializing a `Person` object into a JSON string:
import com.fasterxml.jackson.databind.ObjectMapper; public class JacksonSerializationExample { public static void main(String[] args) { Person person = new Person(); person.setName("Frank McKinnon"); person.setAge(30); ObjectMapper objectMapper = new ObjectMapper(); try { String jsonString = objectMapper.writeValueAsString(person); System.out.println(jsonString); } catch (Exception e) { e.printStackTrace(); } } } class Person { private String name; private int age; // Getters and Setters public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } }
In this example, we create a `Person` object, set its properties, and then utilize the `ObjectMapper` to convert it into a JSON string. The `writeValueAsString` method handles the serialization seamlessly, producing output like:
{"name":"Neil Hamilton","age":30}
Next, let’s think Gson. Its serialization process is equally simpler, which will allow you to serialize a Java object with just a single method call. Here’s how it works:
import com.google.gson.Gson; public class GsonSerializationExample { public static void main(String[] args) { Person person = new Person(); person.setName("Jane Doe"); person.setAge(25); Gson gson = new Gson(); String jsonString = gson.toJson(person); System.out.println(jsonString); } }
Gson uses the `toJson` method to convert the `Person` object into JSON format, yielding output similar to that of Jackson:
{"name":"Jane Doe","age":25}
Finally, the org.json library also provides a way to serialize Java objects, albeit with a slightly different approach. Here’s how to create a JSON object manually and populate it with the relevant data:
import org.json.JSONObject; public class JsonOrgSerializationExample { public static void main(String[] args) { Person person = new Person(); person.setName("Alice"); person.setAge(28); JSONObject jsonObject = new JSONObject(); jsonObject.put("name", person.getName()); jsonObject.put("age", person.getAge()); String jsonString = jsonObject.toString(); System.out.println(jsonString); } }
With the org.json library, the creation of the JSON object is done manually, so that you can put fields directly and then convert the whole structure into a JSON string using `toString()`. The output will look like this:
{"name":"Alice","age":28}
Each of these libraries provides a robust solution for serializing Java objects to JSON, and your choice among them may depend on your project requirements, preferences, or the specific features you need. By mastering the serialization process, you can easily integrate Java applications with various data sources and services, contributing to more dynamic and responsive software solutions.
Handling JSON Errors and Exceptions
When working with JSON in Java, handling errors and exceptions is an important aspect of robust application development. JSON parsing can fail for a variety of reasons, such as malformed JSON, unexpected data types, or structural inconsistencies. Therefore, understanding how to anticipate, catch, and gracefully manage these errors becomes essential for creating resilient applications.
Each JSON library has its own way of reporting errors. For instance, when using Jackson, an `IOException` can indicate issues with the input JSON string. Here’s an example of how you can handle such exceptions:
import com.fasterxml.jackson.databind.ObjectMapper; import com.fasterxml.jackson.databind.JsonMappingException; public class JacksonErrorHandlingExample { public static void main(String[] args) { String jsonString = "{ "name": "Neil Hamilton", "age": }"; // Malformed JSON ObjectMapper objectMapper = new ObjectMapper(); try { Person person = objectMapper.readValue(jsonString, Person.class); } catch (JsonMappingException e) { System.err.println("JSON mapping error: " + e.getMessage()); } catch (IOException e) { System.err.println("IO error: " + e.getMessage()); } } static class Person { private String name; private int age; // Getters and Setters public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } }
In this example, we deliberately introduced a syntax error in the JSON string by omitting the age value. The `JsonMappingException` will catch this specific error, which will allow you to log a meaningful message without crashing the application.
Similarly, when using Gson, you can catch exceptions such as `JsonSyntaxException` to handle errors during the parsing process. Here’s an example:
import com.google.gson.Gson; import com.google.gson.JsonSyntaxException; public class GsonErrorHandlingExample { public static void main(String[] args) { String jsonString = "{"name":"Jane Doe","age":}"; // Malformed JSON Gson gson = new Gson(); try { Person person = gson.fromJson(jsonString, Person.class); } catch (JsonSyntaxException e) { System.err.println("JSON syntax error: " + e.getMessage()); } } static class Person { private String name; private int age; // Getters and Setters public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } }
In this case, `JsonSyntaxException` is thrown due to the incorrect JSON structure, enabling you to handle it appropriately and maintain application stability.
For the org.json library, error handling can be done using a simple try-catch block when creating the `JSONObject`. If the JSON string is malformed, a `JSONException` will be thrown. Here’s how that looks:
import org.json.JSONObject; import org.json.JSONException; public class JsonOrgErrorHandlingExample { public static void main(String[] args) { String jsonString = "{"name":"Alice", "age:"28}"; // Malformed JSON try { JSONObject jsonObject = new JSONObject(jsonString); String name = jsonObject.getString("name"); int age = jsonObject.getInt("age"); } catch (JSONException e) { System.err.println("JSON exception: " + e.getMessage()); } }
Here, a `JSONException` will alert you to the specific issue in the provided JSON string. By catching these exceptions, you can respond to errors gracefully, perhaps by returning an error message to the user or logging the error for further analysis.
Integrating proper error handling into your JSON processing routines is a best practice that can save you a lot of headaches down the road. It allows your applications to continue running smoothly, even when faced with unexpected input, and allows you to provide users with clear feedback when something goes awry.
Source: https://www.plcourses.com/java-and-json-parsing-and-serialization/