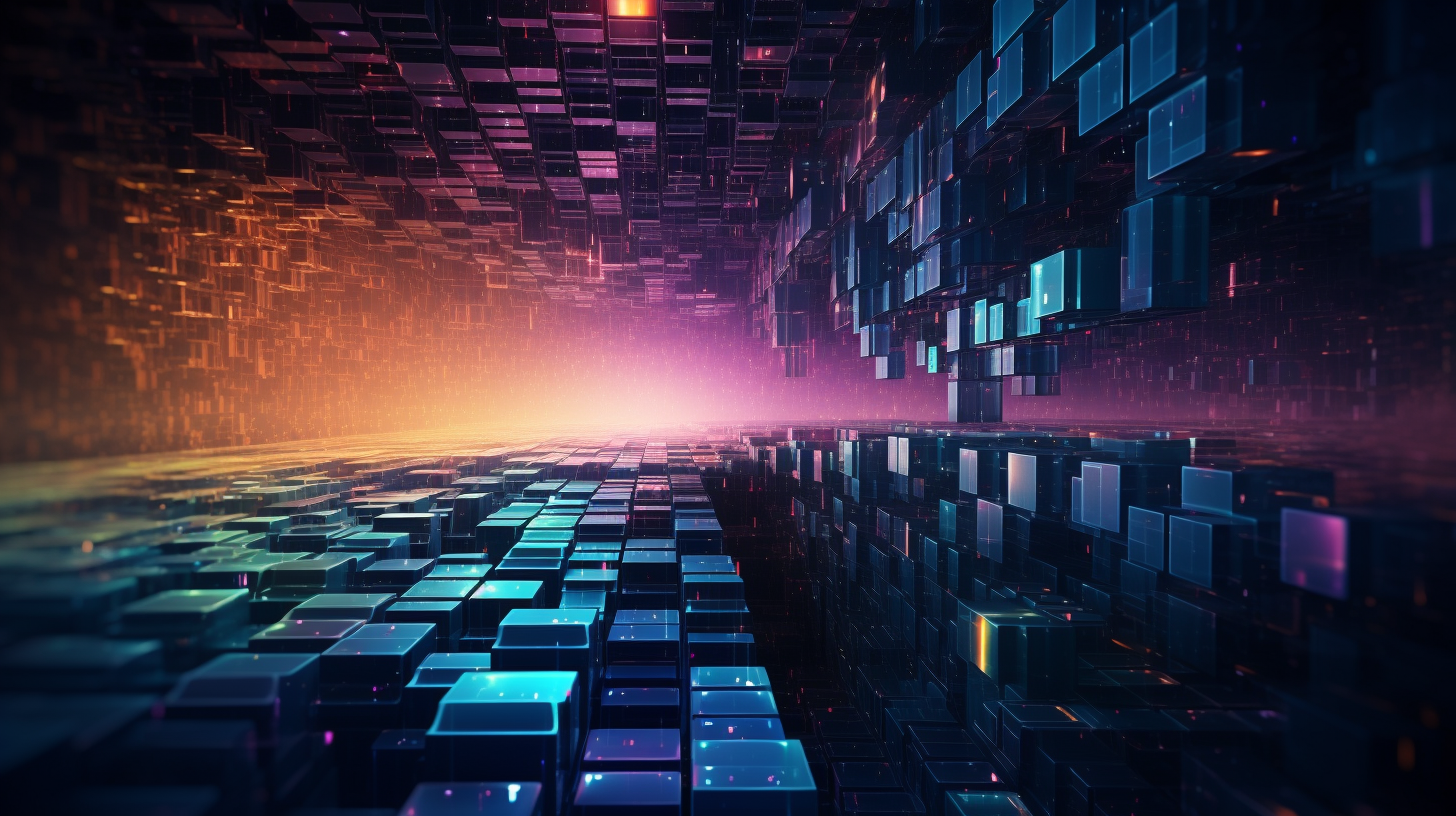
The Lightweight Directory Access Protocol (LDAP) is an application protocol used to access and maintain distributed directory information services over an Internet Protocol (IP) network. At its core, LDAP is designed for efficient querying and modification of directory services, which often include user information, organizational structures, and access controls.
LDAP operates on a client-server model, where the client requests information from an LDAP server. The server, in turn, processes these requests and returns the necessary data from its directory. A key feature of LDAP is its directory information tree (DIT), which organizes data in a hierarchical structure. This tree structure allows for efficient searching and retrieval of entries, which can represent users, groups, resources, or any other entities within a network.
Each entry in an LDAP directory is defined by attributes, each of which has an associated type and value. For instance, a user entry may have attributes such as cn
(common name), sn
(surname), and mail
(email address). The use of standard schema definitions enables consistent representation of data across different LDAP implementations, ensuring interoperability.
LDAP uses a variety of operations to interact with the directory. These operations include:
- Authenticating the client to the directory server.
- Querying the directory for specific entries.
- Adding new entries to the directory.
- Updating existing entries.
- Removing entries from the directory.
One notable aspect of LDAP is its use of a simple textual representation for communication, which makes it accessible for various programming languages and scripting environments, including Bash. The ability to issue LDAP commands directly from the command line or within scripts allows for automation and integration into larger systems.
To illustrate a basic LDAP operation, ponder the following command to search for a user in the directory:
ldapsearch -x -b "dc=example,dc=com" "(uid=jdoe)"
This command performs an anonymous search (-x
) starting at the base distinguished name (DN) dc=example,dc=com
for an entry with the user ID jdoe
. The results can then be processed or manipulated further using Bash scripting, using the power of Unix utilities to handle the output.
Understanding LDAP’s structure and operations is essential for effective integration with Bash. It opens up a wide array of possibilities for managing directory data directly from the command line, allowing for streamlined workflows and enhanced administrative capabilities.
Setting Up an LDAP Server
Setting up an LDAP server involves several key steps, and the process can vary depending on the specific implementation you choose. One of the most popular choices is OpenLDAP, a free software implementation of the LDAP protocol. Below are the general steps you would follow to install and configure an LDAP server using OpenLDAP on a Linux system.
First, ensure that your system is up to date and has the necessary packages installed. On a Debian-based system, you can use the following command to install OpenLDAP and its utilities:
sudo apt-get update sudo apt-get install slapd ldap-utils
During the installation, you will be prompted to set an administrator password for the LDAP directory. It is essential to choose a strong password, as this will control access to your LDAP server.
Next, you will need to configure the LDAP server. The configuration file is typically found at /etc/ldap/slapd.conf or /etc/ldap/slapd.d. The configuration can also be done using a directory management tool such as ldapmodify
. A simple configuration might look like this:
# Example ldif file for setting up the root domain dn: dc=example,dc=com objectClass: domain dc: example dn: cn=admin,dc=example,dc=com objectClass: organizationalRole cn: admin
Once you have created this LDIF file (let’s call it base.ldif
), you can add it to your LDAP directory using the following command:
ldapadd -x -D "cn=admin,dc=example,dc=com" -W -f base.ldif
This command binds to the directory with the admin DN, prompts for the admin password, and imports the contents of base.ldif
into the directory. After this step, you’ll have a basic structure in place.
It’s also important to ensure that your LDAP server is running and configured to start on boot. You can check the status of the slapd service with:
sudo systemctl status slapd
If the service is not running, you can start it with:
sudo systemctl start slapd
Finally, to facilitate secure communication, you may want to enable TLS. This typically involves creating a self-signed certificate or obtaining one from a certificate authority, updating your slapd configuration to include TLS directives, and ensuring your clients can connect securely. You can test your setup using the ldapsearch
command to verify that your LDAP server is operational:
ldapsearch -x -b "dc=example,dc=com" -D "cn=admin,dc=example,dc=com" -W
By following these steps, you will have a functioning LDAP server that can be integrated with Bash for various directory operations. The flexibility of using LDAP alongside Bash scripts allows for powerful automation and management of user and organizational data.
Integrating Bash with LDAP Commands
Integrating Bash with LDAP commands opens a world of possibilities for administrators and developers, allowing them to leverage the power of scripting for automation and management of directory services. With Bash, you can seamlessly execute LDAP commands, manipulate their output, and create dynamic workflows that interact with your LDAP server.
To begin integrating Bash with LDAP, you should familiarize yourself with the primary LDAP command-line tools. The most commonly used tools include ldapsearch
, ldapadd
, ldapmodify
, and ldapdelete
. Each of these commands serves a specific purpose:
- Retrieves entries from the LDAP directory based on specified criteria.
- Adds new entries to the directory.
- Updates existing entries.
- Removes entries from the directory.
Here’s an example of using ldapsearch
in a Bash script to find all users in a specific organizational unit (OU). This script can be extended or modified to suit specific needs:
#!/bin/bash # Base DN for searching BASE_DN="ou=users,dc=example,dc=com" # Search filter SEARCH_FILTER="(objectClass=inetOrgPerson)" # Perform the LDAP search ldapsearch -x -b "$BASE_DN" "$SEARCH_FILTER" cn mail | grep '^cn:|^mail:'
This script sets the base DN to search in the OU for users and uses a filter to find entries of type inetOrgPerson
. The output is then filtered to display only the common name (cn) and email address (mail) of each user.
Moreover, integrating Bash with LDAP commands allows for easy error handling and output manipulation. You can capture the exit status of LDAP commands to determine if they executed successfully. For instance:
ldapsearch -x -b "$BASE_DN" "$SEARCH_FILTER" if [ $? -ne 0 ]; then echo "LDAP search failed." exit 1 fi
In this snippet, the exit status of the ldapsearch
command is checked immediately after execution. If the command fails, an error message is printed, and the script exits with a non-zero status.
When it comes to adding or modifying entries with Bash, you can utilize the ldapadd
and ldapmodify
commands combined with here documents to streamline input. Here’s an example of adding a new user:
#!/bin/bash # New user details USER_DN="uid=jdoe,ou=users,dc=example,dc=com" USER_ATTRIBUTES="dn: $USER_DN objectClass: inetOrgPerson cn: Luke Douglas sn: Doe mail: [email protected] uid: jdoe userPassword: password123" # Add the user to LDAP echo "$USER_ATTRIBUTES" | ldapadd -x -D "cn=admin,dc=example,dc=com" -W
This script defines the new user attributes in a here document format and pipes the output directly into the ldapadd
command. This streamlined approach makes it easy to manage user data through scripts.
For modifying existing entries, similar techniques can be applied using ldapmodify
. The key is to format the changes correctly in an LDIF style:
#!/bin/bash # User DN to modify USER_DN="uid=jdoe,ou=users,dc=example,dc=com" MODIFICATIONS="dn: $USER_DN changetype: modify replace: mail mail: [email protected]" # Modify the user in LDAP echo "$MODIFICATIONS" | ldapmodify -x -D "cn=admin,dc=example,dc=com" -W
In this example, the ldapmodify
command is used to change the email address of the specified user. The modifications are also formatted as a here document and passed through a pipe.
Integrating Bash with LDAP commands not only enhances administrative capabilities but also promotes automation, reducing the potential for human error. By embracing the power of scripting, you can create flexible and efficient processes for managing your LDAP directory.
Performing LDAP Queries Using Bash
#!/bin/bash # Base DN for searching users BASE_DN="dc=example,dc=com" # Search filter for specific attributes SEARCH_FILTER="(objectClass=inetOrgPerson)" # Execute the LDAP search ldapsearch -x -b "$BASE_DN" "$SEARCH_FILTER" cn mail | grep '^cn:|^mail:'
Performing LDAP queries using Bash opens up substantial pathways for automation and integration within various administrative tasks. The primary command for querying LDAP directories is ldapsearch
, which can be tailored to fit a multitude of search criteria, which will allow you to extract exactly the information you need.
To illustrate, consider you want to find all users within a specific organizational unit (OU). The following script performs a search for entries of the inetOrgPerson
class, which typically represents users, and retrieves their common names and email addresses. The results are then filtered using grep
for clarity.
#!/bin/bash # Base DN for searching BASE_DN="ou=users,dc=example,dc=com" # Search filter for users SEARCH_FILTER="(objectClass=inetOrgPerson)" # Perform the LDAP search ldapsearch -x -b "$BASE_DN" "$SEARCH_FILTER" cn mail | grep '^cn:|^mail:'
This use of ldapsearch
allows you to issue a simpler query that interacts directly with your LDAP directory. The base DN specifies where to start the search, and the filter determines which entries to retrieve based on their attributes. The output can be quite verbose, so using grep
ensures that only the relevant parts of the response are displayed.
For more advanced queries, you can dynamically construct search filters and utilize Bash variables to make your scripts more versatile. For instance, if you want to search for users by their email or name, you could modify the search filter based on user input or other script logic.
#!/bin/bash # Read user input for dynamic search read -p "Enter the email or name to search: " USER_INPUT # Create a dynamic search filter SEARCH_FILTER="(|(mail=$USER_INPUT)(cn=$USER_INPUT))" # Perform the LDAP search with dynamic filter ldapsearch -x -b "$BASE_DN" "$SEARCH_FILTER" cn mail | grep '^cn:|^mail:'
This script prompts the user to enter an email address or name, constructs a search filter that looks for either matching email or name, and then executes the query. Such flexibility enables administrators to quickly find entries based on various criteria without hardcoding multiple queries.
Furthermore, when dealing with sensitive operations like searches, it’s a good practice to handle errors gracefully. You can leverage the exit status of ldapsearch
to determine whether your query was successful or if it encountered issues. For example:
#!/bin/bash # Base DN and search filter BASE_DN="dc=example,dc=com" SEARCH_FILTER="(objectClass=inetOrgPerson)" # Execute the LDAP search ldapsearch -x -b "$BASE_DN" "$SEARCH_FILTER" cn mail if [ $? -ne 0 ]; then echo "Error: LDAP search failed." exit 1 fi
In this snippet, after executing the ldapsearch
command, the script checks if the exit status is non-zero, indicating a failure, and then prints an appropriate error message before exiting. This method of error handling is essential for robust scripts, especially in production environments.
By combining these techniques—constructing searches dynamically, filtering output, and managing errors—you can create powerful, flexible Bash scripts that interact seamlessly with your LDAP directory. The ability to automate queries not only saves time but also minimizes human error, enhancing the efficiency of directory management tasks.
Managing LDAP Entries with Bash Scripts
#!/bin/bash # New user details USER_DN="uid=jdoe,ou=users,dc=example,dc=com" USER_ATTRIBUTES="dn: $USER_DN objectClass: inetOrgPerson cn: Nick Johnson sn: Doe mail: [email protected] uid: jdoe userPassword: password123" # Add the user to LDAP echo "$USER_ATTRIBUTES" | ldapadd -x -D "cn=admin,dc=example,dc=com" -W
Managing LDAP entries through Bash scripts is a powerful approach that streamlines administrative tasks, allowing for efficient handling of user data. The integration of Bash and LDAP commands facilitates the automation of adding, modifying, and deleting entries, thus reducing the likelihood of manual errors and saving time.
To add a new user to the LDAP directory, the ldapadd
command is essential. This command requires a formatted input that specifies the user’s attributes, typically in LDIF (LDAP Data Interchange Format). For example, to add a user named Mitch Carter, you would define the relevant attributes and pipe them into ldapadd
. The script provided above illustrates this process clearly.
When it comes to modifying existing entries, ldapmodify
is your go-to command. Similar to ldapadd
, it also requires input formatted in LDIF style. The process involves defining what changes you want to make, such as updating an email address or changing a user’s name. Here’s how you can modify a user’s email address:
#!/bin/bash # User DN to modify USER_DN="uid=jdoe,ou=users,dc=example,dc=com" MODIFICATIONS="dn: $USER_DN changetype: modify replace: mail mail: [email protected]" # Modify the user in LDAP echo "$MODIFICATIONS" | ldapmodify -x -D "cn=admin,dc=example,dc=com" -W
This script changes the email address of the user identified by the distinguished name (DN) provided. The key here is to format the changetype
and specify whether you’re adding, deleting, or replacing attributes. The ability to programmatically alter user information greatly enhances the manageability of your directory.
Deleting entries follows a similar pattern with the ldapdelete
command. Specifying the DN of the user to be deleted is all that’s needed. For instance:
#!/bin/bash # User DN to delete USER_DN="uid=jdoe,ou=users,dc=example,dc=com" # Delete the user from LDAP ldapdelete -x -D "cn=admin,dc=example,dc=com" -W "$USER_DN"
In this script, the user “jdoe” is removed from the LDAP directory by providing their DN to the ldapdelete
command. This simplicity allows for effective management of user entries and can be integrated into larger scripts to automate routine cleanup tasks.
Moreover, error handling plays an important role in managing LDAP entries. After executing any of the LDAP commands, checking the exit status is vital to ensure success:
# Attempt to add a user if echo "$USER_ATTRIBUTES" | ldapadd -x -D "cn=admin,dc=example,dc=com" -W; then echo "User added successfully." else echo "Failed to add user." exit 1 fi
This snippet demonstrates how to check if the user addition was successful and handle failures gracefully. Applying such error handling practices across your scripts ensures robust and reliable directory management.
In summary, managing LDAP entries with Bash scripts allows for significant automation and efficiency in handling user data. By using commands like ldapadd
, ldapmodify
, and ldapdelete
, along with proper error management, administrators can streamline their workflows and maintain a clean and organized directory structure.
Error Handling and Debugging LDAP Operations
Error handling and debugging are critical aspects of working with LDAP operations in Bash. When automating directory management tasks, encountering errors during execution is not uncommon. Understanding how to effectively handle these errors is essential for creating robust and reliable scripts that interact with your LDAP server.
The first step in error handling is to capture the exit status of LDAP commands. In Bash, the special variable $?, which holds the exit status of the last executed command, is invaluable for this purpose. A zero exit status indicates success, while a non-zero value signifies an error. This mechanism can be used to check the outcome of your LDAP operations immediately after they’re executed.
ldapsearch -x -b "dc=example,dc=com" "(objectClass=inetOrgPerson)" if [ $? -ne 0 ]; then echo "Error: LDAP search failed." exit 1 fi
In this snippet, if the ldapsearch command fails, an error message is displayed, and the script exits with a non-zero status. This simple approach can be applied to any LDAP command, providing a simpler way to handle errors as they arise.
Moreover, incorporating detailed logging in your scripts can also significantly aid in debugging. By redirecting output and errors to log files, you can maintain a clear record of operations and diagnose issues after the fact. For example, you could modify your commands as follows:
ldapsearch -x -b "dc=example,dc=com" "(objectClass=inetOrgPerson)" > ldap_search.log 2>&1 if [ $? -ne 0 ]; then echo "Error: LDAP search failed. Check ldap_search.log for details." exit 1 fi
In this case, both standard output and error messages are captured in the ldap_search.log file, enabling you to inspect what went wrong during the execution. This practice is particularly useful for long-running scripts or those that process large amounts of data.
Another crucial consideration is the context of the commands you are executing. Depending on your LDAP server’s configuration and the privileges of the user executing the commands, you may encounter permissions-related errors. To address this, ensure that the credentials used for binding to the LDAP server have the necessary permissions for the operations being performed. You can specify the bind DN and password directly in your script or prompt for them securely:
read -p "Enter LDAP admin DN: " LDAP_ADMIN_DN read -sp "Enter LDAP admin password: " LDAP_ADMIN_PASSWORD echo ldapsearch -x -D "$LDAP_ADMIN_DN" -w "$LDAP_ADMIN_PASSWORD" -b "dc=example,dc=com" "(objectClass=inetOrgPerson)" if [ $? -ne 0 ]; then echo "Error: LDAP search failed. Check your credentials and access rights." exit 1 fi
In addition to error handling, debugging can involve examining the LDAP server logs for additional context when an error occurs. Most LDAP servers maintain logs that provide insight into failed operations, authentication issues, or configuration problems. Accessing these logs can help you pinpoint the source of the problem beyond what is reported by the LDAP command-line tools.
Furthermore, testing your scripts in a controlled environment before deploying them in a production environment can minimize disruptions. By simulating various scenarios and validating your scripts against expected outcomes, you can identify potential points of failure and bolster your error handling strategies accordingly.
By implementing these practices for error handling and debugging LDAP operations in your Bash scripts, you enhance the reliability and maintainability of your directory management tasks. With thoughtful error checks, logging, and testing, you can effectively manage your LDAP environment and reduce the risk of unexpected issues during execution.
Source: https://www.plcourses.com/bash-and-ldap-integration/