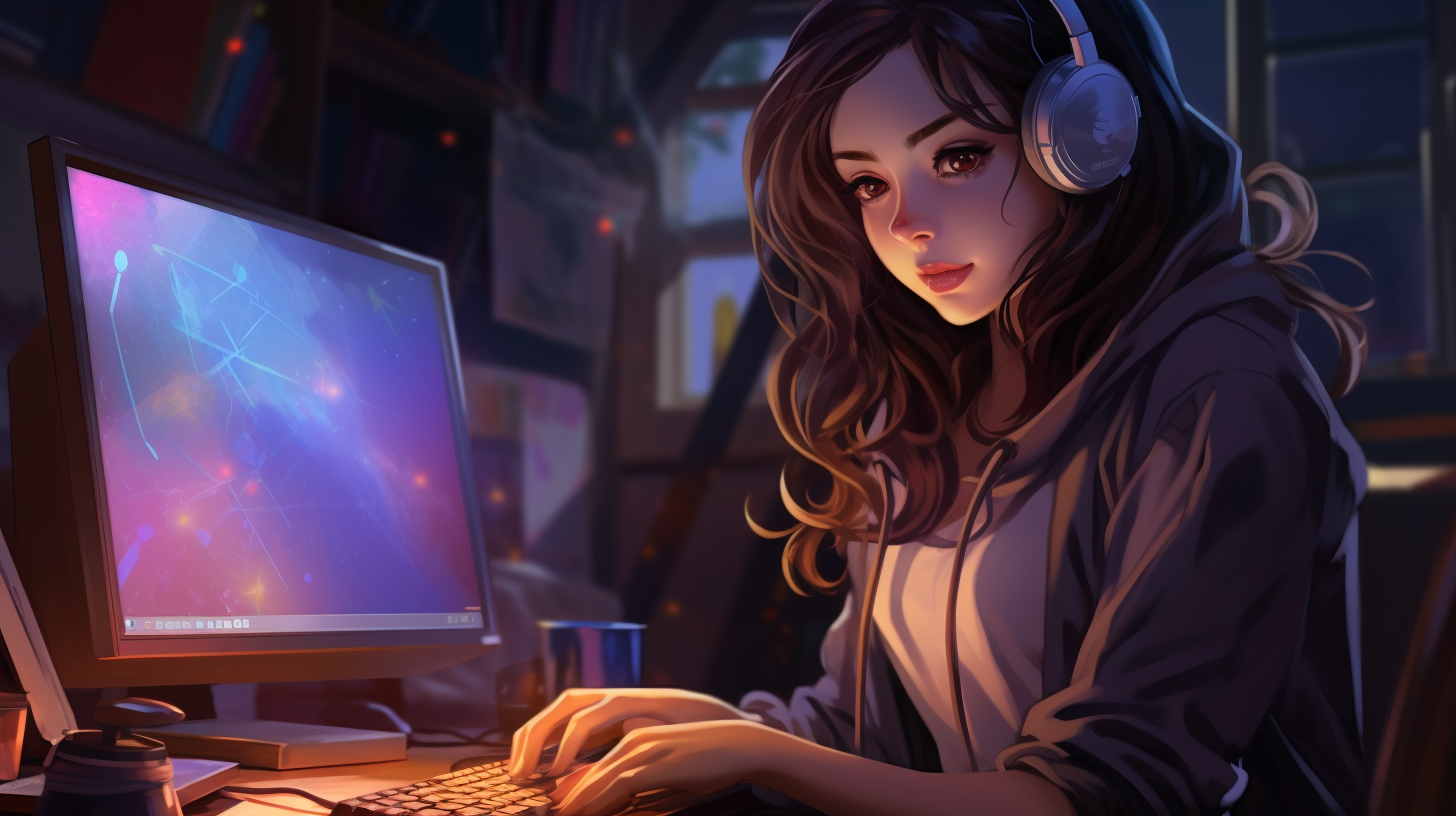
In Java, an enum (short for “enumeration”) is a special data type that enables a variable to be a set of predefined constants. It is a powerful feature of the Java programming language, introduced in Java 5, that allows for type-safe enumerations. By using enums, developers gain more control over the values a variable can hold, ensuring that only valid values are used throughout the code.
Enums help enhance code readability and maintainability by providing meaningful names to a set of related constants, rather than relying on arbitrary integers or strings. This not only reduces the likelihood of errors but also allows for easier integration with switch statements, providing a clearer intention of the code.
To understand enums better, ponder the following example, which defines an enum called Day that represents the days of the week:
public enum Day { SUNDAY, MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY }
In this example, the Day enum defines seven constants, each representing a day of the week. Enums can also have additional fields, methods, and constructors, making them quite flexible. This allows for associating values or behaviors with each constant, which can be particularly useful in complex applications.
Java enums are not just simple lists of constants; they are full-fledged classes that can implement interfaces and encapsulate behavior. For instance, we can associate a numeric value with each day, allowing us to retrieve that value when needed:
public enum Day { SUNDAY(1), MONDAY(2), TUESDAY(3), WEDNESDAY(4), THURSDAY(5), FRIDAY(6), SATURDAY(7); private final int dayNumber; Day(int dayNumber) { this.dayNumber = dayNumber; } public int getDayNumber() { return dayNumber; } }
In this enhanced version of the Day enum, each day is associated with a corresponding number. The constructor assigns the number to the dayNumber field, and the getDayNumber method allows retrieval of the number for any specific day.
This level of abstraction provided by enums makes it easier to manage sets of constants, eliminates the chance of using invalid values, and promotes a clear understanding of what each constant represents. Whether used in switch statements or as parameters to methods, enums ensure that your code remains clean and understandable, showcasing the elegance that Java brings to the table.
Creating and Defining Enums
To create an enum in Java, you simply define it using the enum
keyword followed by the name of the enum and a list of constants enclosed in curly braces. This simpler syntax is designed to be as intuitive as possible, enabling you to easily declare a set of related constants. Each constant in the enum is inherently public, static, and final, ensuring that these values remain constant throughout the program.
Here’s a basic example that illustrates how to create and define an enum representing the seasons of the year:
public enum Season { WINTER, SPRING, SUMMER, FALL }
In this Season
enum, we have four constants: WINTER
, SPRING
, SUMMER
, and FALL
. Each constant represents a specific season, providing a clear and meaningful representation of seasonal values.
Moreover, enums can contain additional fields and methods, enabling you to associate data and behaviors with each constant. Let’s enhance our Season
enum by adding an associated temperature range and a method to retrieve that range:
public enum Season { WINTER(-5, 5), SPRING(5, 20), SUMMER(20, 35), FALL(10, 20); private final int minTemperature; private final int maxTemperature; Season(int minTemperature, int maxTemperature) { this.minTemperature = minTemperature; this.maxTemperature = maxTemperature; } public int getMinTemperature() { return minTemperature; } public int getMaxTemperature() { return maxTemperature; } }
In this version, each season is associated with a minimum and maximum temperature. The constructor initializes these values, and the getMinTemperature
and getMaxTemperature
methods provide access to these associated temperatures.
In addition to constructors and methods, you can also override the toString()
method to provide a more descriptive string representation of each constant. For instance, you might want to include the temperature range in the output:
@Override public String toString() { return this.name() + " (" + minTemperature + "°C to " + maxTemperature + "°C)"; }
This allows you to call System.out.println(Season.SUMMER);
and get a meaningful output like SUMMER (20°C to 35°C)
.
Creating and defining enums in Java is a simpler yet powerful way to manage sets of related constants. Enums can encapsulate data and behavior, making them versatile tools in your programming arsenal. Their design ensures type safety, improves code readability, and reduces the risk of errors, showcasing the elegance and functionality that Java enums bring to software development.
Methods and Properties of Enums
When working with enums in Java, it is crucial to understand the methods and properties that they offer. An enum in Java can not only hold a set of constants but can also have fields, methods, and even implement interfaces. This elevates enums from mere collections of constants to fully-fledged objects with rich behaviors.
Initially, when you define an enum, you can ponder of it as a class with a few default properties. Each constant you define in an enum behaves like an instance of that class. For example, consider the earlier defined Season
enum. In addition to the constants themselves, the enum can contain fields for storing specific properties, such as temperature ranges, as well as methods to access these properties.
public enum Season { WINTER(-5, 5), SPRING(5, 20), SUMMER(20, 35), FALL(10, 20); private final int minTemperature; private final int maxTemperature; Season(int minTemperature, int maxTemperature) { this.minTemperature = minTemperature; this.maxTemperature = maxTemperature; } public int getMinTemperature() { return minTemperature; } public int getMaxTemperature() { return maxTemperature; } @Override public String toString() { return this.name() + " (" + minTemperature + "°C to " + maxTemperature + "°C)"; } }
In this example, the constants are now more informative because they not only represent the seasons but also encapsulate relevant data about each season’s temperature. The methods getMinTemperature
and getMaxTemperature
provide a simpler way to access those values, while the overridden toString
method enhances the output readability, making debugging and logging much easier.
Moreover, enums can also have static methods that provide utility functionality related to the enum itself. For instance, you might want to create a static method that returns an enum constant based on a specific attribute, such as the average temperature:
public static Season fromTemperature(int temperature) { for (Season season : values()) { if (temperature >= season.getMinTemperature() && temperature <= season.getMaxTemperature()) { return season; } } throw new IllegalArgumentException("No season found for temperature: " + temperature); }
This method iterates through the defined enum constants and finds which season falls within the specified temperature range. If no match is found, it throws an exception, ensuring that the program behaves predictably.
Another important aspect of enums is their capability to implement interfaces. This feature allows enums to be utilized polymorphically, meaning you can treat an enum constant as if it were an object of an interface type. Here’s an example where the Season
enum implements a Describable
interface:
public interface Describable { String getDescription(); } public enum Season implements Describable { WINTER(-5, 5), SPRING(5, 20), SUMMER(20, 35), FALL(10, 20); // Previous fields and methods @Override public String getDescription() { return "Season: " + this.toString(); } }
Now, each season can provide a description via the getDescription
method, allowing for a consistent way to represent the enum values across different contexts.
Overall, the methods and properties of Java enums allow you to encapsulate data and behavior within a single, coherent structure, enhancing code readability and maintainability. The ability to define additional fields, implement interfaces, and provide meaningful methods ensures that enums are not just a convenient way to represent a set of constants but a powerful tool for structuring your Java applications.
Best Practices for Using Enums in Java
<= season.getMaxTemperature()) {
return season;
}
}
throw new IllegalArgumentException(“No season found for temperature: ” + temperature);
}
This static method iterates over the defined enum constants using the values()
method, which returns an array of all the enum constants. It checks each season’s temperature range and returns the corresponding season for a given temperature. If no match is found, it throws an exception, which is a good practice to indicate an invalid input.
When using enums in your Java applications, it is important to adhere to best practices to maximize their effectiveness and maintainability.
1. Use Enums for Fixed Sets of Constants: Enums are ideal for representing fixed sets of constants, such as days of the week, colors, or application states. Avoid using them for dynamic sets or values that may change frequently.
2. Leverage Enum Methods: Take advantage of the capability to define methods within your enums. Whether you’re adding utility functions or providing descriptive outputs through overridden methods, encapsulating behavior within an enum enhances its usability.
3. Implement Interfaces: If your enum needs to behave polymorphically, think implementing interfaces. This allows you to define common methods that all enum constants must implement, which is particularly useful in complex systems where different behaviors are needed.
4. Use EnumSet for Collections: When you need to handle groups of enum constants, utilize EnumSet
. This specialized collection class is optimized for use with enums and offers performance benefits over traditional collections like HashSet
.
5. Avoid Adding State to Enums: While enums can have fields, it’s advisable to keep their state simple and immutable. Enums should represent a fixed set of constants without adding mutable state, which can lead to complications and reduced reliability.
6. Use Switch Statements Wisely: Enums integrate seamlessly with switch statements, allowing for cleaner and more maintainable code. However, be cautious about covering all enum constants in your switch cases to avoid potential issues with unhandled cases.
7. Document Your Enums: Proper documentation is vital for maintaining clarity. Each constant in the enum should be well-documented, especially if they represent complex or domain-specific values. This practice helps other developers (or your future self) understand the intent and usage of each constant.
By following these best practices, you can ensure that your usage of enums in Java is not only effective but also maintains the high standards of readability and maintainability that are hallmarks of good programming. The elegance of enums, combined with a thoughtful approach to their design and usage, contributes significantly to the overall quality of your codebase.
Source: https://www.plcourses.com/java-enums-definition-and-usage/