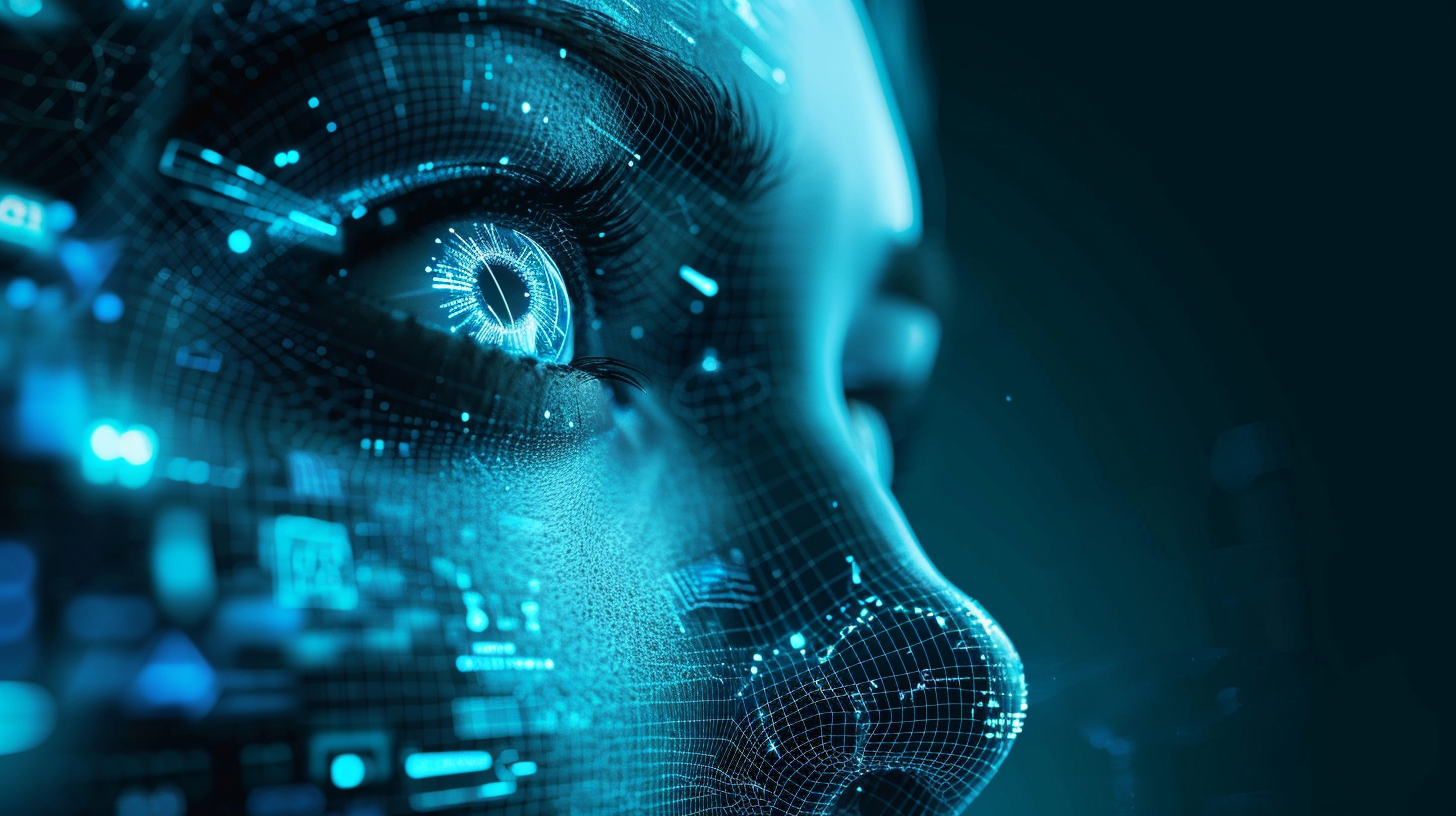
Structured arrays in NumPy are a powerful feature that enables users to handle heterogeneous data types in a single array. Unlike regular NumPy arrays that can only store elements of the same type, structured arrays allow you to create complex data structures that can hold multiple fields with different data types. This capability is particularly useful when dealing with datasets containing mixed types, such as records from a database or complex scientific data.
A structured array consists of multiple fields, each identified by a name and associated with a data type. These fields can be thought of as columns in a table, where each column may contain different types of data, including integers, floats, and strings.
Here is a basic example of creating a structured array:
import numpy as np # Define the structured data type dtype = np.dtype([ ('id', np.int32), ('name', 'U10'), # Unicode string with a max length of 10 ('age', np.int8), ('salary', np.float32) ]) # Create a structured array data = np.array([(1, 'Alice', 30, 70000.0), (2, 'Bob', 25, 50000.0), (3, 'Charlie', 35, 90000.0)], dtype=dtype) print(data)
In this example:
- id, name, age, and salary.
- Each field has a specific data type defined by the
dtype
object. - Data is organized as tuples that are then packed into the structured array.
Accessing fields within a structured array is intuitive. You can retrieve specific fields using their names, similar to accessing columns in a DataFrame. For instance, if you want to fetch the name field of all entries:
names = data['name'] print(names)
Structured arrays also support operations such as filtering and aggregation, allowing users to leverage NumPy’s efficient computation capabilities while handling complex datasets. As you continue exploring structured arrays, you will discover their extensive capabilities for managing and analyzing varied data types effectively.
Creating Structured Arrays in NumPy
Creating structured arrays in NumPy is simpler and allows for great flexibility when dealing with collections of heterogeneous data. To begin creating a structured array, you’ll need to define the data type for each field that will be included in the array. That is done using the `numpy.dtype()` function, where you specify the names and data types of the fields.
Below is a detailed example that illustrates how to create a structured array step by step:
import numpy as np # Define the structured data type dtype = np.dtype([ ('product_id', np.int32), ('product_name', 'U20'), # Unicode string with a max length of 20 ('price', np.float32), ('quantity', np.int32) ]) # Create a structured array with sample data products = np.array([(101, 'Laptop', 999.99, 50), (102, 'Smartphone', 499.99, 150), (103, 'Tablet', 299.99, 75)], dtype=dtype) # Print the structured array print(products)
In this example:
- product_id, product_name, price, and quantity.
- The dtype is set to define the appropriate data types for each field, such as
np.int32
for integers,np.float32
for floating-point numbers, and Unicode strings for text. - Data is provided as a list of tuples that fit within the defined structure, and this helps in ensuring that the data adheres to the specified types.
Structured arrays also allow for complex operations once they are created. For example, you can sort structured arrays based on specific fields. Here’s how to sort based on the price field:
# Sort the structured array by price sorted_products = np.sort(products, order='price') # Print the sorted array print(sorted_products)
When you run the code above, the structured array will be sorted in ascending order based on the price of the products.
In addition to sorting, structured arrays support a variety of operations, including filtering. For example, if you want to filter products that have a quantity greater than 100, you can do so as follows:
# Filter products with quantity greater than 100 filtered_products = products[products['quantity'] > 100] # Print the filtered array print(filtered_products)
This shows that creating structured arrays is not just about defining their structure; it also enables a range of operations that can be performed efficiently thanks to NumPy’s powerful array-processing capabilities.
Accessing and Modifying Data in Structured Arrays
Accessing and modifying data in structured arrays is simpler and provides a flexible way to interact with complex datasets. This section will delve into how you can efficiently retrieve and alter data within the fields of structured arrays.
To access the fields, you can use the field names directly, similar to how you would access columns in a Pandas DataFrame or attributes in an object. Here’s an example that shows how to access specific fields:
import numpy as np # Define the structured data type dtype = np.dtype([ ('employee_id', np.int32), ('name', 'U15'), ('position', 'U10'), ('salary', np.float32) ]) # Create a structured array employees = np.array([(1, 'Alice', 'Manager', 80000.0), (2, 'Bob', 'Developer', 60000.0), (3, 'Charlie', 'Designer', 70000.0)], dtype=dtype) # Accessing the 'name' field names = employees['name'] print(names) # Output: ['Alice' 'Bob' 'Charlie']
In the code above, the `names` variable contains the names of all employees extracted from the structured array. You can also access multiple fields at once by specifying a tuple of field names:
# Accessing multiple fields: 'name' and 'salary' name_salary = employees[['name', 'salary']] print(name_salary)
This outputs a 2D array of the ‘name’ and ‘salary’ fields. Accessing data in this manner allows for more intricate manipulations and analyses.
Modifying data in structured arrays is equally simpler. You can update a specific field using the same indexing techniques. For instance, if you want to give a raise to the ‘Developer’ Bob, you can do it like this:
# Increase Bob's salary by 10% employees['salary'][employees['name'] == 'Bob'] *= 1.10 # Verify the updated salary print(employees)
In this modification example, the salary of the employee with the name ‘Bob’ is increased by 10%. The condition `employees[‘name’] == ‘Bob’` creates a boolean array that allows precise targeting of the relevant entry.
You can also modify entire fields at once. For instance, if you decided to change the position of all employees, you might do the following:
# Change position of all employees to 'Employee' employees['position'][:] = 'Employee' # Verify the changes print(employees)
This example sets the ‘position’ field for all entries to ‘Employee’, demonstrating how you can efficiently update whole fields in one operation.
Accessing and modifying structured arrays is achieved through simpler syntax that utilizes field names, rendering it effortless to perform both retrieval and updates while maintaining the integrity of complex datasets.
Use Cases for Structured Arrays
Structured arrays are particularly useful in scenarios where you need to manage heterogeneous data types efficiently. They can be employed in various domains, including finance, scientific research, and data analysis. Here are some practical use cases that demonstrate their capabilities:
-
Database Records:
Structured arrays can be used to store records retrieved from databases, allowing for easy manipulation and analysis. For instance, you can represent user profiles in a structured array:
import numpy as np # Define the structured data type for user profiles user_dtype = np.dtype([ ('user_id', np.int32), ('username', 'U15'), ('email', 'U25'), ('age', np.int8) ]) # Create a structured array for user profiles user_profiles = np.array([(1, 'Alice', '[email protected]', 28), (2, 'Bob', '[email protected]', 34), (3, 'Charlie', '[email protected]', 22)], dtype=user_dtype) print(user_profiles)
-
Scientific Data Representation:
In scientific computing, structured arrays can represent complex data such as measurements from experiments. For instance, sensor readings can be stored in a structured array:
import numpy as np # Define the structured data type for sensor readings sensor_dtype = np.dtype([ ('timestamp', 'U25'), ('temperature', np.float32), ('humidity', np.float32), ('pressure', np.float32) ]) # Create a structured array for sensor data sensor_readings = np.array([('2023-10-01 10:00:00', 20.5, 30.1, 1012.0), ('2023-10-01 11:00:00', 21.0, 29.5, 1011.8), ('2023-10-01 12:00:00', 22.3, 28.9, 1012.2)], dtype=sensor_dtype) print(sensor_readings)
-
Financial Data Analysis:
Financial applications often require handling mixed types of data, such as transaction records. Structured arrays can simplify this process:
import numpy as np # Define the structured data type for financial transactions transaction_dtype = np.dtype([ ('transaction_id', np.int32), ('date', 'U10'), ('amount', np.float32), ('category', 'U15') ]) # Create a structured array for transactions transactions = np.array([(1001, '2023-09-01', 250.0, 'Groceries'), (1002, '2023-09-02', 150.0, 'Utilities'), (1003, '2023-09-03', 75.0, 'Entertainment')], dtype=transaction_dtype) print(transactions)
-
Data Analysis with Mixed Types:
Structured arrays allow for complex data manipulations, such as grouping or filtering based on different fields. This can be particularly useful in data analysis tasks:
# Filter transactions where amount is greater than 100 high_value_transactions = transactions[transactions['amount'] > 100] print(high_value_transactions)
These use cases illustrate the versatility of structured arrays in handling various types of data efficiently. Whether managing user information, analyzing scientific measurements, or processing financial transactions, structured arrays provide a robust solution for complex datasets.
Performance Considerations with Structured Arrays
When working with structured arrays in NumPy, performance considerations become paramount, especially when handling large datasets or performing complex operations. While structured arrays offer a high number of benefits for managing heterogeneous data, they come with inherent trade-offs in performance compared to traditional homogeneous NumPy arrays. Understanding these considerations can help you make informed decisions about when and how to use structured arrays.
Memory Usage
Structured arrays generally consume more memory than homogeneous arrays due to the overhead associated with storing multiple data types. Each field in a structured array may have different data types and sizes, which can lead to less efficient memory alignment and increased memory fragmentation. Consequently, if you’re working with extensive datasets, the memory overhead may become significant.
To highlight this difference, think the creation of a simple structured array versus a homogeneous array:
import numpy as np # Create a homogeneous array homogeneous_array = np.array([1, 2, 3, 4, 5]) print("Homogeneous array size:", homogeneous_array.nbytes) # Create a structured array structured_array = np.array([(1, 'Alice', 30.5), (2, 'Bob', 25.0)], dtype=[('id', np.int32), ('name', 'U10'), ('age', np.float32)]) print("Structured array size:", structured_array.nbytes)
This code snippet computes the memory size of both array types. The structured array’s size will typically be larger due to the overhead of multiple data types.
Access Speed
Access times for structured arrays can also be less efficient than those for homogeneous arrays. Because each field is stored separately, accessing a single field in a structured array might require additional computation compared to accessing elements of a homogeneous array. This means that operations on structured arrays might experience a performance hit, particularly when accessing numerous elements or performing bulk operations.
For example, comparing access times between a structured array and a homogeneous array would look like this:
import time # Create a structured array with large data large_structured_array = np.array([(i, 'person'+str(i), float(i)) for i in range(100000)], dtype=[('id', np.int32), ('name', 'U10'), ('age', np.float32)]) # Measure access time for a field in a structured array start_time = time.time() _ = large_structured_array['name'] structured_access_time = time.time() - start_time print("Structured array field access time:", structured_access_time) # Create a homogeneous array large_homogeneous_array = np.array([i for i in range(100000)]) # Measure access time for a homogeneous array start_time = time.time() _ = large_homogeneous_array[::2] # Accessing every second element homogeneous_access_time = time.time() - start_time print("Homogeneous array access time:", homogeneous_access_time)
In this snippet, we create large arrays and measure the time to access their elements. Structured arrays might exhibit longer access times here, especially when accessing multiple fields.
Vectorized Operations
One of the key advantages of using NumPy is its ability to perform vectorized operations that are highly optimized. However, structured arrays can sometimes complicate vectorization. Operations on structured arrays may require explicit iteration over fields, which defeats the purpose of using a library designed for efficient computation.
To illustrate, ponder filtering a structured array:
# Filter the structured array based on age filtered_array = large_structured_array[large_structured_array['age'] > 50] print(filtered_array)
This operation shows easy filtering based on a field, but depending on your needs, it could be less efficient than an equivalent operation on a homogeneous array.
Batch Operations
When performing operations on structured arrays, it’s often beneficial to batch processes together to minimize performance penalties. Instead of accessing fields one at a time, ponder summarizing data in a single pass. For instance, using aggregate functions across structured arrays can enhance performance:
# Calculate the average age average_age = np.mean(large_structured_array['age']) print("Average age:", average_age)
This example emphasizes the efficiency gained when using vectorized operations on structured arrays, demonstrating that applying functions directly can yield better performance than iterating through individual elements.
Structured arrays cater to valuable use cases where data types vary significantly. However, it is essential to stay mindful of their performance characteristics, including memory usage, access speeds, and the best practices for using vectorized operations. By applying these considerations strategically, you can maximize the performance benefits while using the rich features provided by structured arrays in NumPy.
Advanced Operations and Functions on Structured Arrays
In exploring the advanced operations and functions available for structured arrays in NumPy, we can leverage their rich capabilities to perform a wide range of tasks efficiently. Beyond basic creation and data retrieval, structured arrays support complex manipulations and calculations that can enhance data analysis workflows.
A common advanced operation is sorting structured arrays based on specific fields. For instance, if you want to sort employee records by salary, you can easily do this using the `np.sort` function with the specified order:
import numpy as np # Define the structured data type for employees dtype = np.dtype([ ('employee_id', np.int32), ('name', 'U15'), ('position', 'U10'), ('salary', np.float32) ]) # Create a structured array employees = np.array([(1, 'Alice', 'Manager', 80000.0), (2, 'Bob', 'Developer', 60000.0), (3, 'Charlie', 'Designer', 70000.0)], dtype=dtype) # Sort employees by salary sorted_employees = np.sort(employees, order='salary') # Print sorted employees print(sorted_employees)
This snippet will output the employee records sorted by the salary in ascending order, demonstrating how structured arrays can be flexibly sorted using a specific field.
Another powerful operation is aggregation, which can be performed across various fields in the structured array. For example, if you want to calculate the average salary of your employees, you can access the salary field directly and apply the `np.mean` function:
# Calculate the average salary average_salary = np.mean(employees['salary']) print("Average salary:", average_salary)
This method directly accesses the salary field of the structured array and computes the average, showcasing the effectiveness of NumPy’s vectorized operations.
Structured arrays also enable the use of boolean indexing for filtering data. For instance, if you want to find all employees who are ‘Designers’, you can use boolean indexing to filter the structured array:
# Filter employees who are Designers designers = employees[employees['position'] == 'Designer'] print(designers)
This operation allows you to extract the subset of data that meets specific criteria, making structured arrays a powerful tool for data selection.
Moreover, structured arrays allow the application of custom functions to the fields. For instance, if you need to apply a tax deduction to all employee salaries, you could define a function and use it in conjunction with a structured array:
def apply_tax(salary, tax_rate): return salary * (1 - tax_rate) # Apply a tax deduction of 20% tax_rate = 0.20 employees['salary'] = apply_tax(employees['salary'], tax_rate) # Print employees after applying tax print(employees)
In this example, the `apply_tax` function is used to update the salary of each employee, effectively modifying the structured array in one operation.
Lastly, advanced operations can also include combining multiple structured arrays using `np.concatenate` or `np.vstack`, which allows for creating larger datasets from smaller structured arrays.
# Create another structured array new_employees = np.array([(4, 'David', 'Developer', 65000.0)], dtype=dtype) # Combine the original and new employee structured arrays all_employees = np.concatenate((employees, new_employees)) # Print all employees print(all_employees)
This capability to combine arrays efficiently is instrumental when aggregating data from various sources.
By using these advanced operations and functions, structured arrays in NumPy become powerful allies for data scientists and analysts needing to manipulate heterogeneous information efficiently and effectively. This flexibility makes structured arrays a go-to choice for various data-intensive applications.
Source: https://www.pythonlore.com/exploring-structured-arrays-in-numpy/