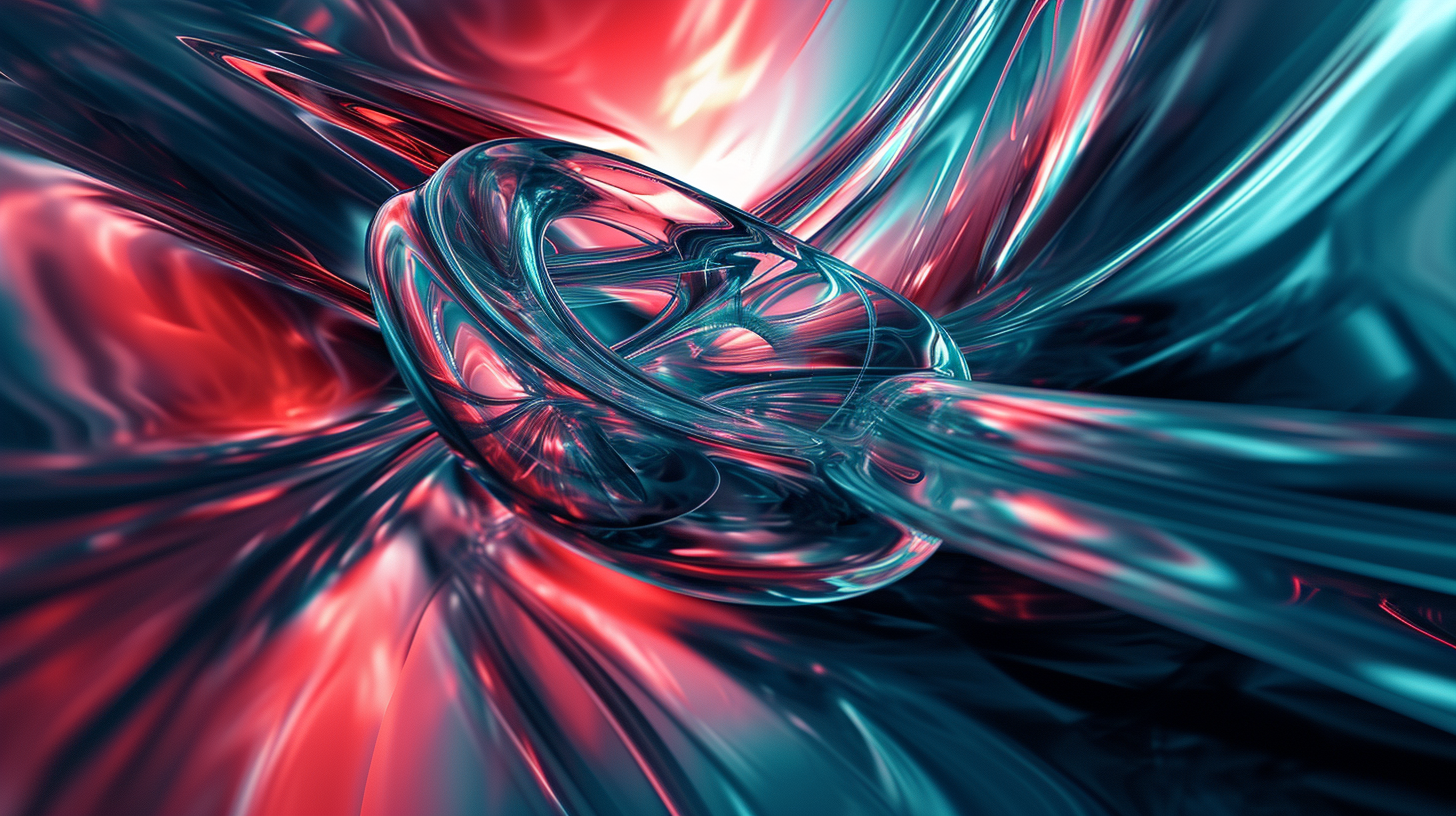
BSON, short for Binary JSON, is a binary representation of JSON-like documents. It’s the underlying data format used by MongoDB to store documents. Unlike JSON, which is text-based and requires more space, BSON is designed to be efficient for data storage and retrieval. It provides a more compact format, enabling faster processing of data due to its binary structure.
One of the main advantages of BSON is its support for additional data types beyond what is available in JSON. For instance, BSON supports types such as date, binary, and ObjectId, which allow for a more flexible representation of various data types. This flexibility is particularly useful when working with complex data structures.
BSON also includes a length prefix for each document, making it easier for MongoDB to determine the size of the document in memory. This can enhance the performance of read and write operations, as MongoDB can efficiently manage the location and size of documents.
Furthermore, BSON supports embedded documents and arrays, which enables developers to create rich data models that can capture the intricacies of real-world entities. This hierarchical nature of BSON documents aligns closely with the needs of applications that utilize NoSQL databases.
Here’s a brief overview of key aspects of BSON:
- BSON is a serialized binary format, making it smaller and faster to process compared to JSON.
- It supports a variety of data types, such as:
- A unique identifier for documents.
- Supports date and time values.
- Allows storage of raw binary data.
- BSON is specifically designed for MongoDB, optimizing both storage and retrieval.
- Enables nesting of documents and arrays for richer data structures.
The nature of BSON as a binary representation of JSON documents allows for efficient data storage, retrieval, and management, making it an ideal choice for working with MongoDB.
Common BSON Data Types and Their Uses
In this section, we will explore some of the most common BSON data types, their unique characteristics, and their typical applications within MongoDB and Pymongo.
- The ObjectId is a 12-byte unique identifier for documents in a MongoDB collection. It’s generated automatically by MongoDB when a document is created and is typically used as the default value for the
"_id"
field. The format includes a timestamp, machine identifier, process identifier, and a random value for uniqueness.
from bson.objectid import ObjectId # Example of creating an ObjectId new_id = ObjectId() print(new_id) # Output will be a new unique ObjectId
from datetime import datetime from bson import DateTime # Example of creating a BSON Date object current_date = DateTime(datetime.now()) print(current_date) # Output will show the current date in BSON format
from bson import Binary # Example of creating a BSON Binary object data = b'x00x01x02' # Example binary data binary_data = Binary(data) print(binary_data) # Output will show the binary representation of the data
from bson import Array # Example of creating a BSON Array my_array = Array([1, 2, 3, "example"]) print(my_array) # Output will show the BSON array representation
from bson import Document # Example of creating an embedded document embedded_doc = Document({ "name": "Frank McKinnon", "age": 30, "address": { "street": "123 Elm St", "city": "Springfield" } }) print(embedded_doc) # Output will show the embedded document
The diverse BSON data types enable developers to create comprehensive data models that accurately reflect the requirements of their applications. Understanding these types allows for more effective use of MongoDB’s capabilities in storing, retrieving, and manipulating data.
Working with BSON Data Types in Pymongo
With the understanding of BSON data types established, we can now delve into how to work with these data types using Pymongo, the official MongoDB driver for Python. Pymongo provides a simpler interface to interact with MongoDB, allowing developers to perform various operations on BSON documents seamlessly.
To get started with Pymongo, ensure that you have it installed in your Python environment. You can install it using pip:
pip install pymongo
Once Pymongo is installed, you can establish a connection to a MongoDB instance and start working with BSON data types. Below are some basic operations demonstrating how to use BSON types within Pymongo.
First, let’s create a connection to a MongoDB server:
from pymongo import MongoClient # Connect to MongoDB (running locally on the default port 27017) client = MongoClient('mongodb://localhost:27017/') db = client['mydatabase'] # Specify the database collection = db['mycollection'] # Specify the collection
Now that we have our database and collection set up, we can start inserting documents using various BSON data types. Below are examples of inserting different types of BSON documents:
from bson.objectid import ObjectId from bson.binary import Binary from bson import DateTime from datetime import datetime # Creating a document with different BSON types document = { "_id": ObjectId(), # Unique identifier "name": "Alice", "age": 28, "registered_on": DateTime(datetime.now()), # BSON Date "profile_picture": Binary(b'x89PNGrnx1an'), # Example binary data "skills": ["Python", "MongoDB", "Data Analysis"], # BSON Array "address": { # BSON Embedded Document "street": "456 Oak St", "city": "Metropolis", "zipcode": "12345" } } # Insert the document into the collection collection.insert_one(document) print("Document inserted:", document["_id"])
After the above operation, you will successfully insert a BSON document into your MongoDB collection with various data types included. To verify that the document was inserted, you can retrieve it and display its contents:
# Retrieve the document by its ObjectId inserted_document = collection.find_one({"_id": document["_id"]}) print("Retrieved Document:", inserted_document)
Pymongo makes it easy not only to insert but also to query and manipulate BSON data. You can use various filtering criteria to retrieve documents based on specific BSON data types. Here’s an example of how to retrieve documents based on a BSON Date:
from datetime import timedelta # Query documents registered after a certain date threshold_date = datetime.now() - timedelta(days=30) # 30 days ago recent_docs = collection.find({"registered_on": {"$gt": DateTime(threshold_date)}}) print("Recent Registrations:") for doc in recent_docs: print(doc)
This example demonstrates how to utilize BSON data types in queries, allowing for more sophisticated date comparisons directly within the MongoDB query language.
Working effectively with BSON data types in Pymongo allows developers to leverage the full power of MongoDB’s capabilities. By understanding how to manipulate various BSON types, one can create complex data models suited for a wide range of applications.
Converting Python Data Types to BSON
In this section, we will discuss how to convert standard Python data types into BSON format, facilitating seamless integration between Python applications and MongoDB. Given the differences between BSON and JSON, as well as the unique support BSON provides for certain data types, it’s crucial to handle conversions carefully to ensure data integrity and optimal performance.
Pymongo provides built-in methods for converting Python data types to BSON automatically when inserting documents into MongoDB. However, understanding the process of manual conversion can be beneficial, especially when preparing data before sending it to the database or when working with data fetched from external sources. Below are common Python data types and their corresponding BSON representations:
- Standard Python strings are easily mapped to BSON string type without conversion.
- Python integers and floating-point numbers are also directly convertible to BSON numeric types.
- The boolean values
True
andFalse
translate to BSON boolean type seamlessly. - Python lists are converted to BSON arrays, making it simpler to work with collections of items.
- Python dictionaries map to BSON embedded documents, allowing for nested structures.
- Python’s
datetime
objects must be explicitly converted using Pymongo’s BSON date utilities as shown below.
Here are a few examples of converting Python data types to BSON:
from bson import Binary, ObjectId, DateTime from datetime import datetime # Example conversions string_value = "Hello, MongoDB!" # Python string to BSON string int_value = 42 # Python integer to BSON int float_value = 3.14 # Python float to BSON float bool_value = True # Python boolean to BSON boolean list_value = [1, 2, 3, "Hello"] # Python list to BSON array dict_value = {"key": "value", "number": 100} # Python dict to BSON embedded document # Example of converting Python datetime to BSON DateTime current_time = DateTime(datetime.now()) # Example of creating BSON Binary from bytes binary_value = Binary(b'x01x02x03') # Print converted values print("Converted BSON values:") print("String:", string_value) print("Integer:", int_value) print("Float:", float_value) print("Boolean:", bool_value) print("List:", list_value) print("Dictionary:", dict_value) print("DateTime:", current_time) print("Binary:", binary_value)
When inserting documents into MongoDB using Pymongo, the conversion is handled automatically. For instance, here’s how a dictionary containing various data types can be inserted:
from pymongo import MongoClient # Connecting to MongoDB client = MongoClient('mongodb://localhost:27017/') db = client['mydatabase'] collection = db['mycollection'] # Create a document with various types document = { "greeting": "Hello, world!", "age": 30, "height": 5.9, "is_student": False, "hobbies": ["reading", "gaming"], "address": {"city": "Metropolis", "zip": "54321"}, "joined_on": DateTime(datetime.now()), "binary_data": Binary(b'x89PNGrn') # Binary data } # Insert the document into the collection collection.insert_one(document) print("Document inserted successfully.")
In this process, Pymongo automatically converts Python types into the corresponding BSON data types upon insertion. This automatic conversion capability makes it easy for developers to work with data in a flexible manner without needing to frequently manage data type conversions manually.
Understanding how to convert Python data types to BSON is essential for optimizing interactions with MongoDB. When developers are equipped with this knowledge, they can create robust applications that process and store a variety of data types more efficiently.
Best Practices for Using BSON with MongoDB
When working with BSON in MongoDB, it’s essential to follow best practices to ensure optimal performance and maintainability of your applications. Here are several key recommendations for effectively using BSON data types with MongoDB:
- Always choose the most suitable BSON data type for storing your data. For instance, if you need to store dates, use the BSON Date type instead of strings. This ensures better data integrity, indexing, and performance.
- While BSON supports embedded documents, excessive nesting can lead to complex queries and performance issues. Keep your documents as flat as possible while still maintaining the required structure and relationships.
- Use indexes wisely by indexing only the fields that are frequently queried. Sparse indexing helps optimize storage and speed by creating indices only on documents that contain the indexed field.
- MongoDB has a document size limit of 16 MB. Ensure that individual documents do not approach this size by splitting large objects into smaller, manageable parts or embedding data judiciously.
- When dealing with binary data, ensure it’s encoded correctly and avoids large binary objects that can degrade performance. Ponder using GridFS for storing large files instead.
- Implement schema validation to ensure that the documents conform to the desired structure and data types. This can help prevent data integrity issues and enforce rules within your collections.
- Use projection to retrieve only the necessary fields when querying documents. This minimizes the amount of data sent over the network and speeds up query performance.
- Regularly analyze the performance of your queries and the overall system. Use tools like MongoDB Atlas or built-in monitoring to identify slow queries, and optimize your data model and indexing strategies accordingly.
- Before deploying changes to your BSON structures or MongoDB queries, perform thorough testing and profiling to identify potential performance bottlenecks, ensuring a smooth user experience.
By adhering to these best practices, developers can leverage the full potential of BSON and MongoDB, ensuring efficient data handling, better performance, and a more robust application architecture.
Source: https://www.pythonlore.com/utilizing-bson-data-types-in-mongodb-with-pymongo/