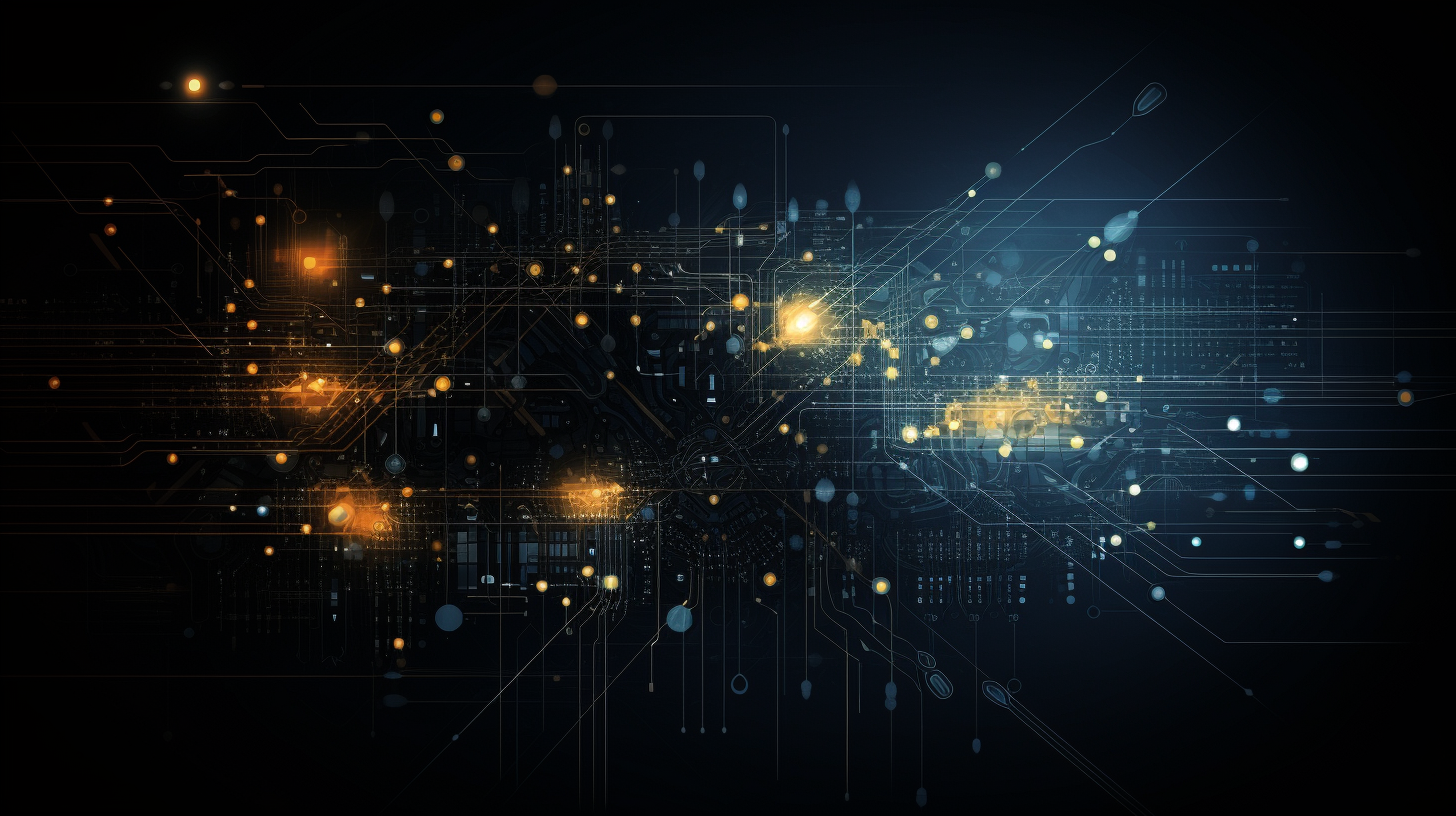
The SQL UPDATE statement is pivotal in data management, enabling the modification of existing records within a database table. Understanding its structure and functionality is important for efficient database interactions.
The basic syntax of the UPDATE statement is straightforward:
UPDATE table_name SET column1 = value1, column2 = value2, ... WHERE condition;
In this syntax:
- The name of the table where you want to update the records.
- Indicates which columns should be updated along with their new values.
- A critical clause that specifies which records to update. Omitting this clause will update all records in the table, which can lead to unintended data changes.
To illustrate, consider a table named employees with columns first_name, last_name, and salary. If we want to give a raise to an employee named ‘John’, we can execute the following UPDATE statement:
UPDATE employees SET salary = salary * 1.10 WHERE first_name = 'John';
This command increases John’s salary by 10%. The importance of the WHERE clause cannot be overstated; without it, every employee’s salary would be increased by 10%.
Moreover, it’s possible to update multiple columns at once. For instance, if John gets a promotion along with a raise, the statement would look like this:
UPDATE employees SET salary = salary * 1.10, last_name = 'Doe' WHERE first_name = 'John';
In this example, we not only update John’s salary but also change his last name to ‘Doe’.
Another vital aspect is the ability to use subqueries within the UPDATE statement. This can be particularly useful for setting a column value based on the result of a query. For instance, if we want to set the salary of all employees to the average salary of the company, we can do so as follows:
UPDATE employees SET salary = (SELECT AVG(salary) FROM employees);
In this case, all employees will have their salary updated to the calculated average, demonstrating the flexibility and power of the SQL UPDATE statement.
Batch Updates: Strategies and Techniques
Batch updates in SQL are essential when dealing with large datasets or when multiple records need to be modified at the same time. They allow for efficient data management by minimizing the number of separate update operations needed, which can lead to reduced server load and improved performance.
One effective strategy for batch updates is to leverage the CASE statement within the UPDATE command. This approach allows you to specify different new values for different records in a single statement, saving time and resources. For example, if we want to update the salaries of several employees based on their department, we can do it as follows:
UPDATE employees SET salary = CASE WHEN department = 'Sales' THEN salary * 1.10 WHEN department = 'Engineering' THEN salary * 1.15 WHEN department = 'HR' THEN salary * 1.05 ELSE salary END WHERE department IN ('Sales', 'Engineering', 'HR');
In this example, we use the CASE statement to apply different salary increases based on the employee’s department. This method is particularly powerful because it allows you to consolidate multiple updates into a single SQL command.
Another technique for batch updates is using temporary tables or common table expressions (CTEs). These structures can temporarily hold the new values to be updated and allow for more complex logic in determining which records to update. Here is an example using a temporary table:
CREATE TEMPORARY TABLE new_salaries ( employee_id INT, new_salary DECIMAL(10, 2) ); INSERT INTO new_salaries (employee_id, new_salary) VALUES (1, 60000.00), (2, 75000.00), (3, 50000.00); UPDATE employees SET salary = ns.new_salary FROM new_salaries ns WHERE employees.id = ns.employee_id;
This method allows for a more organized approach to batch updates, particularly when the new values are sourced from an external calculation or file. By inserting the new values into a temporary table, we can then perform a JOIN operation in the UPDATE statement, ensuring that each employee’s salary is updated accurately.
Moreover, when working with very large datasets, it can be beneficial to perform updates in smaller batches to avoid locking issues and to maintain database performance. This can be achieved using a loop structure in procedural SQL or by using a limit clause in the update query. For example:
DO $$ DECLARE batch_size INT := 1000; BEGIN LOOP UPDATE employees SET salary = salary * 1.05 WHERE id IN (SELECT id FROM employees WHERE salary < 50000 LIMIT batch_size) RETURNING id INTO some_variable; EXIT WHEN NOT FOUND; -- Exit loop if no rows were updated END LOOP; END $$;
This approach allows the database to handle smaller chunks of data at a time, which can be particularly important in high-traffic environments. By employing these strategies and techniques, developers can optimize their batch update processes, ensuring both efficiency and effectiveness in their SQL operations.
Using Joins for Efficient Updates
When it comes to updating records efficiently, using joins in SQL can significantly enhance performance and accuracy. By integrating updates with join operations, we can modify multiple records across different tables based on related data, which is particularly useful in complex relational databases.
The syntax for updating with joins can vary slightly depending on the SQL dialect, but the core concept remains consistent: we combine the UPDATE statement with a JOIN clause to link the target table with another table from which we derive the new values. This approach minimizes the need for subqueries and enhances readability and maintainability.
Think an example where we have two tables: employees and departments. The employees table contains employee details, while the departments table holds information about departmental budgets for salary adjustments. If we need to increase the salaries of employees based on their respective department’s budget, we can perform a join in the update statement:
UPDATE employees SET salary = salary * 1.10 FROM departments WHERE employees.department_id = departments.id AND departments.budget > 100000;
In this command, we effectively link the employees table with the departments table. The update is executed only for those employees whose corresponding department’s budget exceeds $100,000. This method not only simplifies the update process but also ensures that the updates are contextually relevant, enhancing the integrity of the data.
Another scenario could be updating an employee’s position based on their performance rating stored in a separate performance_reviews table. We can use a join to establish this relationship:
UPDATE employees SET position = 'Senior ' || position FROM performance_reviews WHERE employees.id = performance_reviews.employee_id AND performance_reviews.rating = 'Excellent';
In this case, employees who received an ‘Excellent’ rating will have ‘Senior ‘ prefixed to their current position, reflecting their performance and promoting organizational growth. The use of concatenation here demonstrates how we can manipulate string values during an update operation.
It is imperative to ponder the execution plan for such operations, as joins can sometimes lead to performance bottlenecks if not handled carefully. Indexing the join columns—like department_id and employee_id—can significantly improve the speed of the update operation, especially with large datasets.
Moreover, when performing updates with joins, always ensure that the WHERE clause is adequately defined to prevent unintentional updates across the entire dataset. In many cases, it may be beneficial to first run a SELECT statement to review the affected rows:
SELECT employees.id, employees.salary, departments.budget FROM employees JOIN departments ON employees.department_id = departments.id WHERE departments.budget > 100000;
This step ensures clarity and provides insight into which records will be modified, safeguarding against unwanted changes.
Using joins for updates not only streamlines the SQL operations but also allows for more dynamic and context-aware modifications. By using relationships between tables, we can efficiently manage data updates, ensuring that the operations are both effective and aligned with business logic.
Best Practices for Performance Optimization
Optimizing performance in SQL updates is important for maintaining database efficiency, especially as the size and complexity of your datasets grow. There are several best practices developers can adopt to ensure that their update operations run smoothly and swiftly.
1. Use Indexes Wisely
Indexes play a significant role in enhancing update performance. When you update records, SQL must locate them quickly based on the WHERE clause conditions. If appropriate indexes are in place, the database engine can identify and access the records to be updated with greater efficiency. However, be cautious; while indexes speed up read operations, they can slow down write operations, including updates. Therefore, it’s essential to strike a balance between read and write performance by indexing only those columns that are frequently used in WHERE clauses.
2. Minimize the Scope of Updates
To reduce the amount of data that needs to be processed during an update, always limit the scope of your update statements. Employ precise WHERE clauses to target only the records that genuinely need updating. For example:
UPDATE employees SET salary = salary * 1.05 WHERE last_review_date < CURRENT_DATE - INTERVAL '1 year';
This statement ensures that only employees who haven’t had a review in over a year will receive a salary increase, thus minimizing unnecessary updates.
3. Batch Updates
When dealing with large datasets, consider batching your updates. Updating too many records simultaneously can lead to locks and contention in the database, which might degrade performance. By breaking down updates into smaller chunks, you can maintain better overall performance and reduce the chance of locking issues. This technique can be implemented using a loop or a similar structure:
DO $$ DECLARE batch_size INT := 1000; BEGIN LOOP UPDATE employees SET salary = salary * 1.05 WHERE id IN (SELECT id FROM employees WHERE salary < 50000 LIMIT batch_size); EXIT WHEN NOT FOUND; -- Exit loop if no rows were updated END LOOP; END $$;
4. Avoid Unnecessary Updates
Before executing an update, it is wise to check if the new value differs from the current value. This prevents unnecessary write operations and can significantly enhance performance. You can accomplish this by incorporating an additional condition in the WHERE clause:
UPDATE employees SET salary = salary * 1.10 WHERE salary < 50000 AND last_review_date < CURRENT_DATE - INTERVAL '1 year';
Here, the update proceeds only if the salary is below a certain threshold, effectively preventing unnecessary updates for employees already earning more than $50,000.
5. Optimize Transaction Management
When performing updates within a transaction, aim to keep the transaction scope as narrow as possible. This reduces lock contention and allows the database to manage concurrent transactions more effectively. Commit the transaction as soon as you have completed the necessary updates:
BEGIN; UPDATE employees SET salary = salary * 1.10 WHERE department_id = 2; COMMIT;
This approach ensures that your database remains responsive and that other operations can proceed without undue delay.
6. Analyze Query Performance
Lastly, regularly analyze the performance of your update queries using tools provided by your database management system. Most DBMSs offer execution plans that allow you to see how the database engine processes your SQL statements. By examining these plans, you can identify potential bottlenecks, such as missing indexes or inefficient joins, and take corrective actions accordingly.
By adhering to these best practices for performance optimization, SQL developers can ensure that their update operations are not only efficient but also maintain the integrity and responsiveness of their databases over time.
Source: https://www.plcourses.com/efficiently-updating-multiple-rows-with-sql/