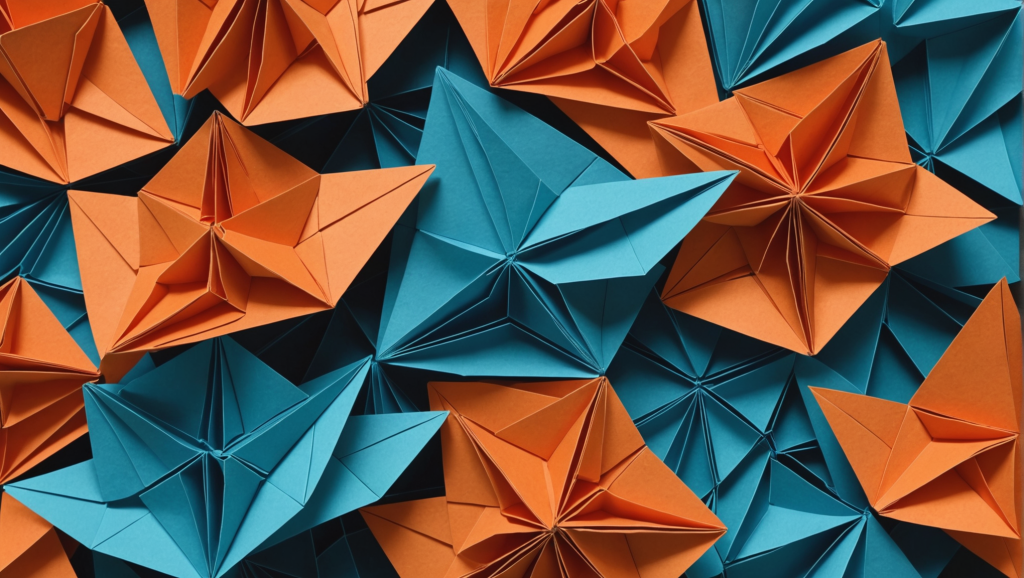
Within the scope of database design, the idea of model relationships serves as the bedrock upon which complex data structures are built. The ability to represent real-world scenarios through these relationships very important for the efficiency and efficacy of any database system. The three primary types of relationships in databases are one-to-one, one-to-many, and many-to-many, each offering a unique way of connecting entities.
A one-to-one relationship signifies that one entity in a database corresponds to exactly one entity in another table. This relationship is less common but can be useful in scenarios where specific attributes of an entity need to be isolated into a separate table for clarity or security.
The one-to-many relationship is perhaps the most prevalent. In this case, a single entity can relate to multiple entities in another table. For instance, think a library system where one author can have multiple books. Here, the authors can be linked to their respective books through a foreign key in the books table, effectively encapsulating the relationship.
On the other hand, the many-to-many relationship presents a more intricate structure, where multiple entities in one table can relate to multiple entities in another. This necessitates the use of a junction table, which holds foreign keys that reference the primary keys of both entities. A classic example would be a scenario involving students and courses; a student can enroll in multiple courses, while a course can have multiple students.
Understanding these relationship types is paramount as they dictate how data is organized, queried, and managed. The choice of relationship impacts not only the integrity of the data but also the performance of database operations. Let us delve deeper into these intricate connections by examining how we can implement them effectively in Python.
Many-to-Many Relationships Explained
In the sphere of many-to-many relationships, it is pivotal to comprehend the dual connections that can exist between two entities. Unlike the simpler one-to-one and one-to-many relationships, many-to-many relationships necessitate a more sophisticated approach to data management within relational databases. Here, each instance of an entity can be associated with an arbitrary number of instances from another entity, and vice versa. This reciprocal linkage is typically represented in databases through the utilization of a junction table, which acts as an intermediary to facilitate these connections.
To illustrate this concept, ponder a simplified model involving students and courses within a university system. A student may enroll in multiple courses throughout their academic career, and at once, a single course may accommodate numerous students. To effectively model this relationship, a junction table—commonly referred to as an enrollment table—comes into play. This enrollment table will contain foreign keys referencing the primary keys of both the students and courses tables.
In practice, this relationship can be realized through the following three tables:
Students Table: - student_id (Primary Key) - student_name Courses Table: - course_id (Primary Key) - course_name Enrollment Table (Junction Table): - student_id (Foreign Key referencing Students) - course_id (Foreign Key referencing Courses)
Each entry in the Enrollment Table represents a unique pairing of a student to a course, thus capturing the essence of a many-to-many relationship. Managing such relationships requires careful attention to detail, as the database must enforce referential integrity, ensuring that every enrollment corresponds to a valid student and course.
In Python, particularly when using an Object-Relational Mapping (ORM) framework like SQLAlchemy, we can express this many-to-many relationship succinctly. The SQLAlchemy library permits the definition of these relationships through its declarative base. Below is an exemplar code illustrating how one might construct such a model:
from sqlalchemy import create_engine, Column, Integer, String, ForeignKey from sqlalchemy.orm import relationship, backref from sqlalchemy.ext.declarative import declarative_base Base = declarative_base() class Student(Base): __tablename__ = 'students' student_id = Column(Integer, primary_key=True) student_name = Column(String) courses = relationship('Enrollment', back_populates='student') class Course(Base): __tablename__ = 'courses' course_id = Column(Integer, primary_key=True) course_name = Column(String) students = relationship('Enrollment', back_populates='course') class Enrollment(Base): __tablename__ = 'enrollment' student_id = Column(Integer, ForeignKey('students.student_id'), primary_key=True) course_id = Column(Integer, ForeignKey('courses.course_id'), primary_key=True) student = relationship(Student, back_populates='courses') course = relationship(Course, back_populates='students') engine = create_engine('sqlite:///:memory:') Base.metadata.create_all(engine)
In the code above, we define three classes, each representing a table in the database. The Student and Course classes encapsulate the student and course entities, while the Enrollment class models the junction table. The bidirectional relationships are established through the `relationship()` function, which allows for both navigating from students to their courses and from courses back to their enrolled students.
This implementation exemplifies how many-to-many relationships can be effectively managed within Python, enabling rich interactions between entities while maintaining a structured and coherent database design. It is imperative, however, to ensure that the design adheres to the principles of normalization to avoid redundancy and maintain data integrity.
Implementing Many-to-Many Relationships in Python
When it comes to implementing many-to-many relationships in Python, using an Object-Relational Mapping (ORM) library such as SQLAlchemy not only simplifies the process but also increases the expressiveness of the code. Notably, SQLAlchemy’s declarative syntax allows for the creation of relationships that are both intuitive and reflective of the underlying database schema.
Let us revisit our student-course relationship, which serves as a common illustration of many-to-many associations. To flesh this out further, ponder the scenario where we want to not only enroll students in courses but also harness the capability to manage the enrollments programmatically. For this, we might want to incorporate additional attributes into our enrollment table, such as the enrollment date or grade. This necessitates a slight enhancement to our implementation.
from sqlalchemy import create_engine, Column, Integer, String, ForeignKey, Date from sqlalchemy.orm import relationship, backref from sqlalchemy.ext.declarative import declarative_base from datetime import date Base = declarative_base() class Student(Base): __tablename__ = 'students' student_id = Column(Integer, primary_key=True) student_name = Column(String) enrollments = relationship('Enrollment', back_populates='student') class Course(Base): __tablename__ = 'courses' course_id = Column(Integer, primary_key=True) course_name = Column(String) enrollments = relationship('Enrollment', back_populates='course') class Enrollment(Base): __tablename__ = 'enrollment' student_id = Column(Integer, ForeignKey('students.student_id'), primary_key=True) course_id = Column(Integer, ForeignKey('courses.course_id'), primary_key=True) enrollment_date = Column(Date, default=date.today) grade = Column(String) student = relationship(Student, back_populates='enrollments') course = relationship(Course, back_populates='enrollments') engine = create_engine('sqlite:///:memory:') Base.metadata.create_all(engine)
In this enhanced model, the Enrollment
class now includes two additional attributes: enrollment_date
and grade
. The enrollment_date
field is automatically set to the current date upon the creation of an enrollment instance, thanks to the default=date.today
functionality.
By using this model, we can easily manage enrollments with added context. For example, let’s create a function to enroll a student in a course, handling both the instantiation of the enrollment and the management of the relationships appropriately:
from sqlalchemy.orm import sessionmaker Session = sessionmaker(bind=engine) session = Session() def enroll_student(student_id, course_id): enrollment = Enrollment(student_id=student_id, course_id=course_id) session.add(enrollment) session.commit() # Example usage: enroll_student(1, 2)
This simple function encapsulates the logic for enrolling a student in a course. With the session management inherent to SQLAlchemy, we efficiently handle data persistence, ensuring the integrity of our many-to-many relationships is maintained throughout.
Thus, by carefully constructing our models and functions, Python and SQLAlchemy enable us to implement many-to-many relationships with elegance and clarity, reflective of the complexities inherent in real-world applications. It is worth noting, however, that as the complexity of relationships increases, additional considerations for performance, indexing, and data integrity may become necessary to uphold the principles of robust database design.
One-to-Many Relationships and Their Use Cases
In contrast to many-to-many relationships, the one-to-many relationship serves as a vital construct in the landscape of database design, often acting as the primary mechanism for associating a single entity with multiple related entities. This relationship manifests when a record in one table can be related to many records in another table, while each record in the second table corresponds solely to a single record in the first. The prevalence of this relationship type arises from its natural applicability to a variety of domain models, making it indispensable for modeling hierarchical data structures.
To illustrate this relationship, consider the scenario of a company with a high number of employees. Each employee can be associated with only one department, yet a department can encompass multiple employees. This illustrates a quintessential one-to-many relationship, where the “Department” entity relates to multiple “Employee” entities. In database terminology, the “Department” table will include a primary key, while the “Employee” table will incorporate a foreign key that references this primary key.
The schema for such a relationship may look as follows:
-- Department Table CREATE TABLE departments ( department_id INT PRIMARY KEY, department_name VARCHAR(100) ); -- Employee Table CREATE TABLE employees ( employee_id INT PRIMARY KEY, employee_name VARCHAR(100), department_id INT, FOREIGN KEY (department_id) REFERENCES departments(department_id) );
In the aforementioned design, the department_id
serves as a foreign key in the employees
table, establishing the linkage to the departments
table. This binary relationship effectively encapsulates the hierarchal structure whereby a department can be viewed as a parent entity, whilst the employees represent child entities.
From a programmatic perspective, implementing a one-to-many relationship in Python using an ORM like SQLAlchemy is simpler. Below, we define the relevant data models using SQLAlchemy’s declarative base. This allows us to express the relationship clearly and concisely:
from sqlalchemy import create_engine, Column, Integer, String, ForeignKey from sqlalchemy.orm import relationship, declarative_base Base = declarative_base() class Department(Base): __tablename__ = 'departments' department_id = Column(Integer, primary_key=True) department_name = Column(String) employees = relationship('Employee', back_populates='department') class Employee(Base): __tablename__ = 'employees' employee_id = Column(Integer, primary_key=True) employee_name = Column(String) department_id = Column(Integer, ForeignKey('departments.department_id')) department = relationship(Department, back_populates='employees') engine = create_engine('sqlite:///:memory:') Base.metadata.create_all(engine)
In this code, we define the Department
class, which encapsulates the properties and relationships relevant to the departments table. The Employee
class similarly represents its table, with a foreign key that establishes its relationship to the department. The relationship()
function is employed to set up a bidirectional connection between the two entities, allowing us to navigate easily from departments to their employees and vice versa.
Practical usage of this relationship model can be illustrated through a simple example. Think the task of adding new employees to a department:
from sqlalchemy.orm import sessionmaker Session = sessionmaker(bind=engine) session = Session() def add_employee(employee_name, department_id): new_employee = Employee(employee_name=employee_name, department_id=department_id) session.add(new_employee) session.commit() # Example usage: add_employee('Albert Lee', 1)
This function efficiently encapsulates the logic for adding an employee to a particular department, ensuring that the relationship remains intact. As we observe, the implementation of one-to-many relationships in Python is not merely a matter of technology, but a reflection of the structured way in which data can be interrelated to mirror real-world scenarios.
As we delve deeper into one-to-many relationships, it’s essential to recognize their broad applicability across various domains, such as in e-commerce for products and categories, and in academic settings with courses and enrollments. Each instance of the relationship underscores the need for careful design, ensuring that data integrity and the principles of normalization are maintained throughout the entire lifecycle of the models. Thus, the one-to-many relationship stands not only as a foundational component of relational databases but also as a framework for understanding the hierarchical and categorical nature of data in application development.
Building One-to-Many Relationships in Python
Building a one-to-many relationship in Python can be seamlessly achieved with the aid of an Object-Relational Mapping (ORM) library, such as SQLAlchemy. This allows developers to express the complexities of relational data through simple class definitions, effectively bridging the gap between the database and application logic. To expand upon our previous discussion of one-to-many relationships, consider a scenario wherein we need to manage the interactions between a bookstore and its inventory of books.
In this example, a single bookstore can possess multiple books, but each book is uniquely associated with one bookstore. This structure exemplifies the one-to-many relationship, with the Bookstore representing the “one” side and the book as the “many” side. Accordingly, our data model will consist of two tables: one for the bookstores and another for the books, with the latter table containing a foreign key that references the primary key of the bookstore table.
The database schema for this relationship might be delineated as follows:
-- Bookstore Table CREATE TABLE bookstores ( bookstore_id INT PRIMARY KEY, bookstore_name VARCHAR(100) ); -- Book Table CREATE TABLE books ( book_id INT PRIMARY KEY, book_title VARCHAR(100), bookstore_id INT, FOREIGN KEY (bookstore_id) REFERENCES bookstores(bookstore_id) );
Here, the bookstore_id
in the books table serves as a foreign key, establishing a direct association with the bookstores table. This design not only reinforces the linkage between books and their respective bookstores but also facilitates queries that can efficiently retrieve all books belonging to a specific bookstore.
To express this relationship in Python using SQLAlchemy, we will utilize its declarative base to define our data models. Below is an illustration of how one might implement this structure:
from sqlalchemy import create_engine, Column, Integer, String, ForeignKey from sqlalchemy.orm import relationship, declarative_base Base = declarative_base() class Bookstore(Base): __tablename__ = 'bookstores' bookstore_id = Column(Integer, primary_key=True) bookstore_name = Column(String) books = relationship('Book', back_populates='bookstore') class Book(Base): __tablename__ = 'books' book_id = Column(Integer, primary_key=True) book_title = Column(String) bookstore_id = Column(Integer, ForeignKey('bookstores.bookstore_id')) bookstore = relationship(Bookstore, back_populates='books') engine = create_engine('sqlite:///:memory:') Base.metadata.create_all(engine)
In this code, we define two classes, Bookstore
and Book
, each corresponding to a table in our database. The relationship is established using the relationship()
function, which allows us to navigate from a bookstore to its books and vice versa with ease.
To exemplify practical usage, let us consider a function to add a new book to a bookstore. This function encapsulates the logic required to maintain the integrity of the one-to-many relationship:
from sqlalchemy.orm import sessionmaker Session = sessionmaker(bind=engine) session = Session() def add_book(book_title, bookstore_id): new_book = Book(book_title=book_title, bookstore_id=bookstore_id) session.add(new_book) session.commit() # Example usage: add_book('The Art of Computer Programming', 1)
This function takes in a book_title
and a bookstore_id
, creating a new Book
instance linked to the specified bookstore. Upon invoking the function, the book is seamlessly added to our database, maintaining the structural integrity of our one-to-many relationship.
The capacity to manage one-to-many relationships in Python is invaluable, particularly as applications grow in complexity. The clear representation of relational data through ORM facilitates better organization of code and data, ensuring that the relationships mirror real-world instances accurately.
Moreover, as the data model evolves, developers must remain vigilant about enforcing data integrity and adhering to best practices in database design. Thus, the one-to-many relationship not only serves to fulfill our immediate data requirements but also lays the groundwork for scalable and maintainable application architecture.
Best Practices for Managing Complex Relationships
As we venture into the realm of complex model relationships, it becomes essential to adopt best practices for managing these intricate connections. The effective handling of many-to-many and one-to-many relationships is critical for maintaining the integrity and performance of our database systems. Herein, we will explore several paramount practices that can aid in the management of such relationships across various scenarios.
1. Use Junction Tables Wisely
In many-to-many relationships, the introduction of a junction table serves as a necessary intermediary to link the related entities. Proper design of this junction table is paramount; ensure it not only contains foreign keys that reference the primary keys of the related tables but also incorporates other relevant attributes that may enrich the relationship. For instance, in an academic context with a student-course relationship, adding a timestamp for enrollment or a grade field can vastly improve the utility of the junction table.
CREATE TABLE enrollment ( student_id INT, course_id INT, enrollment_date DATE, grade VARCHAR(2), FOREIGN KEY (student_id) REFERENCES students(student_id), FOREIGN KEY (course_id) REFERENCES courses(course_id), PRIMARY KEY (student_id, course_id) );
2. Enforce Referential Integrity
The enforcement of referential integrity is fundamental in relational databases. This principle ensures that foreign keys in the child table correlate directly to valid primary keys in the parent table. In practice, this can be achieved using database constraints, which will prevent the insertion of orphan records that do not correspond to existing entities. Using ORM features like those in SQLAlchemy can simplify this enforcement, as relationships specified in the model will automatically generate the necessary constraints at the database level.
class Enrollment(Base): __tablename__ = 'enrollment' student_id = Column(Integer, ForeignKey('students.student_id'), primary_key=True) course_id = Column(Integer, ForeignKey('courses.course_id'), primary_key=True)
3. Optimize Queries
With complex relationships comes the potential for inefficient queries that can drastically affect performance. Utilize joins judiciously and prefer SQLAlchemy’s eager loading techniques to pre-fetch related entities. This reduces the number of database hits and enhances performance by allowing you to load all necessary related data in a single query rather than multiple subsequent queries.
from sqlalchemy.orm import joinedload students_with_courses = session.query(Student).options(joinedload(Student.courses)).all()
4. Maintain Clear and Consistent Naming Conventions
The implementation of clear naming conventions for tables and fields aids significantly in maintaining readability and usability of the database schema. Adopting a consistent naming strategy that reflects the nature of the relationships and the role of each entity can prevent confusion as models proliferate in complexity. For instance, prefixing a junction table with the names of the two related entities (e.g., StudentCourse) can provide immediate context about its purpose.
5. Document Relationships
As designs grow increasingly intricate, documenting the relationships and their corresponding rationale becomes ever more necessary. This documentation may take the form of an Entity-Relationship Diagram (ERD) or inline comments within function definitions. Including descriptions of each table, its purpose, and the relationships involved can demystify the structure for any developer who might interact with the codebase in the future.
6. Regularly Review and Refactor
Think the growth of the application and regularly assess the database schema for instances of redundancy or inefficiency. As requirements evolve, the original design may require adjustments to maintain optimal performance and usability. Refactoring schema, normalizing tables where necessary, and reviewing how relationships are managed can yield significant performance benefits while enhancing maintainability.
Adhering to these best practices fosters a robust infrastructure for managing complex relationships in databases. The conscientious application of these principles ensures that our data models remain efficient, clear, and reflective of the underlying requirements of the application, thereby paving the way for sustainable development and scalability.
Real-World Applications of Advanced Model Relationships
In the practical realm of data management, the application of advanced model relationships unveils a plethora of possibilities for enhancing application functionality and user experience. By using the intricacies of both many-to-many and one-to-many relationships, one can effectively model real-world scenarios that exhibit a degree of complexity often encountered in various domains. Let us ponder a few notable applications where these relationships come to life, showcasing their utility and impact.
One prominent application lies within the domain of e-commerce platforms. In such systems, a product can belong to multiple categories, and conversely, categories can encompass a variety of products. This characteristic aptly illustrates a many-to-many relationship, effectively managed through a junction table linking products to categories. By implementing this model, e-commerce sites can offer users an efficient navigation experience, empowering them to filter products across multiple categories, thereby enhancing accessibility and discoverability.
from sqlalchemy import create_engine, Column, Integer, String, ForeignKey from sqlalchemy.orm import relationship, declarative_base Base = declarative_base() class Product(Base): __tablename__ = 'products' product_id = Column(Integer, primary_key=True) product_name = Column(String) categories = relationship('ProductCategory', back_populates='product') class Category(Base): __tablename__ = 'categories' category_id = Column(Integer, primary_key=True) category_name = Column(String) products = relationship('ProductCategory', back_populates='category') class ProductCategory(Base): __tablename__ = 'product_category' product_id = Column(Integer, ForeignKey('products.product_id'), primary_key=True) category_id = Column(Integer, ForeignKey('categories.category_id'), primary_key=True) product = relationship(Product, back_populates='categories') category = relationship(Category, back_populates='products') engine = create_engine('sqlite:///:memory:') Base.metadata.create_all(engine)
In addition, the social media landscape is replete with examples of sophisticated relationship modeling. Think a scenario where users can follow multiple other users, and those users can reciprocate the following action. Here, the relationship between users can be depicted as many-to-many, necessitating a junction table to persist user follow relationships. This model not only underpins the functionality of social networks but also supports algorithmic features such as recommendations and content feeds based on user interactions.
class User(Base): __tablename__ = 'users' user_id = Column(Integer, primary_key=True) username = Column(String) followers = relationship('Follow', foreign_keys='Follow.following_id', back_populates='following') following = relationship('Follow', foreign_keys='Follow.follower_id', back_populates='follower') class Follow(Base): __tablename__ = 'follows' follower_id = Column(Integer, ForeignKey('users.user_id'), primary_key=True) following_id = Column(Integer, ForeignKey('users.user_id'), primary_key=True) follower = relationship(User, foreign_keys=[follower_id], back_populates='followers') following = relationship(User, foreign_keys=[following_id], back_populates='following') engine = create_engine('sqlite:///:memory:') Base.metadata.create_all(engine)
Moreover, in educational environments, the relationship between students, courses, and instructors epitomizes the necessity of both many-to-many and one-to-many relationships. Students can enroll in multiple courses while each course can be taught by multiple instructors. Additionally, each instructor is typically assigned to one or more courses, establishing a one-to-many relationship. This layered structure facilitates the management of educational data, enabling efficient tracking of enrollments, course offerings, and instructor assignments.
class Instructor(Base): __tablename__ = 'instructors' instructor_id = Column(Integer, primary_key=True) instructor_name = Column(String) courses = relationship('Course', back_populates='instructor') class Course(Base): __tablename__ = 'courses' course_id = Column(Integer, primary_key=True) course_name = Column(String) instructor_id = Column(Integer, ForeignKey('instructors.instructor_id')) students = relationship('Enrollment', back_populates='course') instructor = relationship(Instructor, back_populates='courses') class Enrollment(Base): __tablename__ = 'enrollment' student_id = Column(Integer, ForeignKey('students.student_id'), primary_key=True) course_id = Column(Integer, ForeignKey('courses.course_id'), primary_key=True) student = relationship(Student, back_populates='courses') course = relationship(Course, back_populates='students') engine = create_engine('sqlite:///:memory:') Base.metadata.create_all(engine)
Thus, the integration of advanced model relationships serves not merely as an academic exercise but as a vital component across various sectors, enhancing the functionality and effectiveness of applications. The ability to model these complex interactions allows for a richer representation of the real world, enabling systems to respond dynamically to user needs and behaviors. As we embrace the intricacies of these relationships in our designs, we pave the way for creating applications that are as versatile as they’re powerful, echoing the complexity and beauty of the environments they are intended to serve.
Source: https://www.pythonlore.com/advanced-model-relationships-many-to-many-one-to-many/