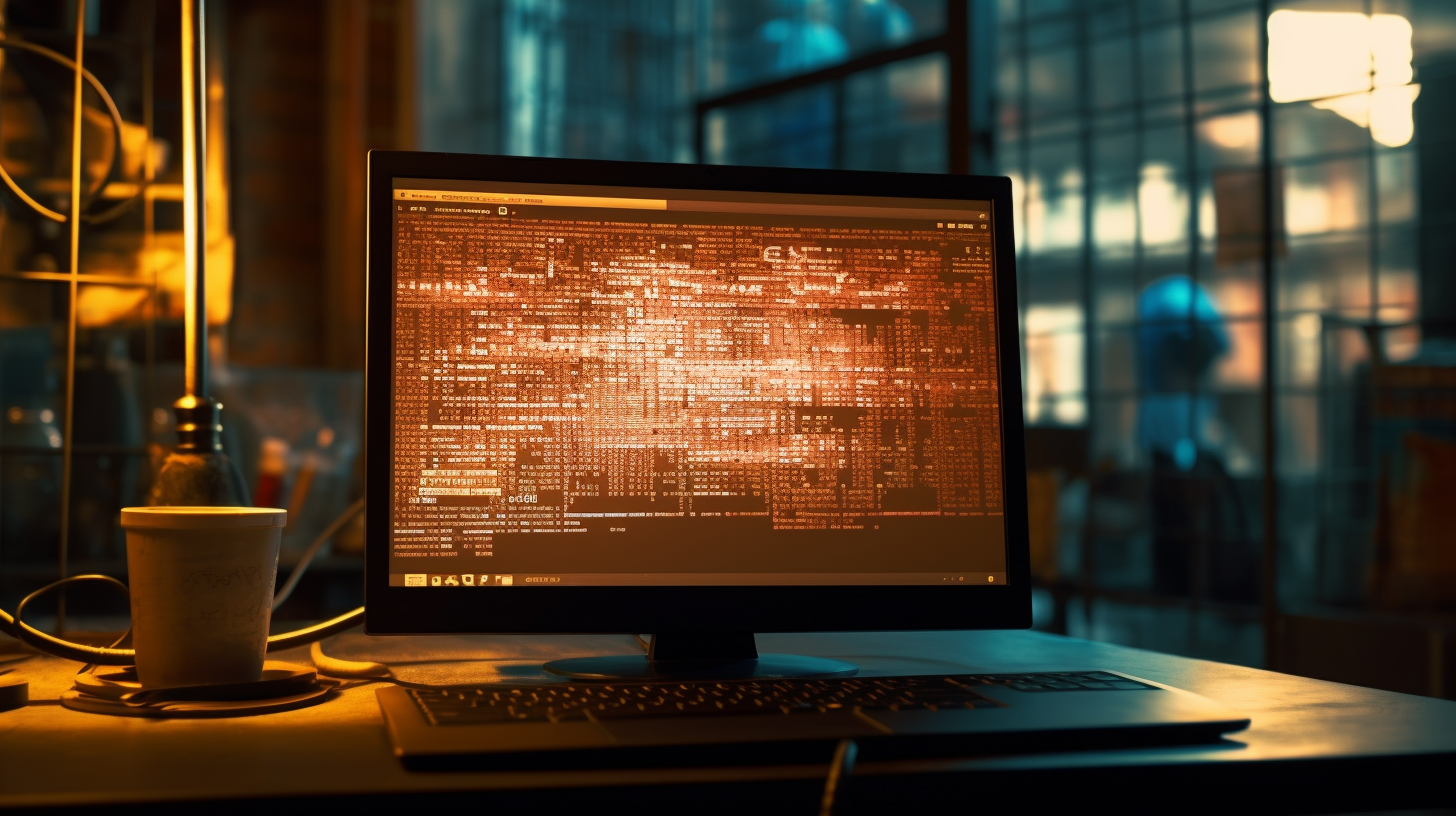
Object-oriented programming (OOP) in Java is a paradigm that revolves around the idea of “objects”, which can be thought of as instances of classes. These classes serve as blueprints for creating objects and encapsulate data as well as behaviors associated with that data. The principles of OOP are fundamental to the design and implementation of software this is both modular and easily maintainable.
At the core of OOP are four key principles: encapsulation, inheritance, polymorphism, and abstraction. Each principle plays a critical role in how developers structure their code, enabling a level of organization and reuse that’s essential for building robust applications.
Encapsulation is the practice of keeping the internal state of an object hidden from the outside world. That is achieved by restricting direct access to some of the object’s components, often through the use of access modifiers. By doing so, encapsulation helps maintain data integrity and enables the implementation of a clear interface for interacting with the object.
class BankAccount { private double balance; public BankAccount(double initialBalance) { balance = initialBalance; } public void deposit(double amount) { if (amount > 0) { balance += amount; } } public double getBalance() { return balance; } }
In the example above, the balance
variable is encapsulated within the BankAccount
class. It cannot be accessed directly from outside the class, ensuring that it can only be modified through the provided methods, deposit
and getBalance
.
Inheritance allows a new class to inherit properties and methods from an existing class. This creates a hierarchical relationship where the subclass can add or modify functionalities of the superclass, promoting code reuse and reducing redundancy.
class SavingsAccount extends BankAccount { private double interestRate; public SavingsAccount(double initialBalance, double interestRate) { super(initialBalance); this.interestRate = interestRate; } public void applyInterest() { double interest = getBalance() * interestRate; deposit(interest); } }
Here, the SavingsAccount
class extends the BankAccount
class, inheriting its properties and methods while also introducing a new method, applyInterest
. This demonstrates how inheritance can enhance the functionality of existing classes.
Polymorphism is another powerful aspect of OOP that allows methods to do different things based on the object that it is acting upon, even when they share the same name. This is typically accomplished through method overriding in subclasses.
class CheckingAccount extends BankAccount { public CheckingAccount(double initialBalance) { super(initialBalance); } @Override public void deposit(double amount) { if (amount > 0) { super.deposit(amount - 1); // Deducting a transaction fee } } }
In this example, the CheckingAccount
class overrides the deposit
method, demonstrating polymorphism. It provides a specialized behavior that differs from the base class, thus showcasing the adaptability of OOP.
Abstraction involves simplifying complex reality by modeling classes based only on essential attributes and behaviors. This principle enables developers to focus on interactions at a higher level while hiding the underlying complexity.
The principles of object-oriented programming provide a framework for building flexible, maintainable, and scalable software systems in Java. By understanding and applying these principles, developers can create code that’s not only effective but also elegant, reflecting the sophistication of the OOP paradigm.
Classes and Objects: The Building Blocks of Java
The foundational elements of object-oriented programming in Java are classes and objects. Classes serve as the templates or blueprints from which objects are instantiated. Each class can define its own data structures and methods, encapsulating both the state (data) and behavior (functions) relevant to the objects derived from it. Understanding how to utilize classes and objects effectively especially important for any Java developer.
In Java, a class is defined using the class
keyword, followed by the class name, which should start with an uppercase letter. The class body is enclosed in curly braces. Inside the class, you define fields (variables) and methods (functions) that dictate what the object can do and what data it can hold. Here is a simple example:
class Car { // Fields private String color; private String model; // Constructor public Car(String color, String model) { this.color = color; this.model = model; } // Method to display car details public void displayDetails() { System.out.println("Car Model: " + model + ", Color: " + color); } }
In the Car
class above, we have defined two fields: color
and model
. The constructor initializes these fields when a new object is created. The method displayDetails
serves to output the state of the object. To create an instance of the class, you can use the following code:
public class Main { public static void main(String[] args) { // Creating an object of Car Car myCar = new Car("Red", "Toyota Corolla"); myCar.displayDetails(); // Output: Car Model: Toyota Corolla, Color: Red } }
Once the Car
class is defined, you can create multiple objects of the Car
class, each with different states. This encapsulation of data allows you to create complex systems with unique behaviors while maintaining clear boundaries between different components.
Java also supports the concept of object references. When you create an object, you’re actually creating a reference to that object in memory. If you assign one object reference to another, both references point to the same object:
Car anotherCar = myCar; anotherCar.displayDetails(); // Output: Car Model: Toyota Corolla, Color: Red // Changing the state through another reference anotherCar = new Car("Blue", "Honda Civic"); anotherCar.displayDetails(); // Output: Car Model: Honda Civic, Color: Blue myCar.displayDetails(); // Still outputs: Car Model: Toyota Corolla, Color: Red
This behavior emphasizes the importance of understanding object references, as altering an object through one reference does not affect others unless they’re explicitly pointing to the same object.
Classes can also facilitate the creation of complex data types through the use of methods. Methods can be defined to manipulate the state of an object or to perform operations based on the object’s attributes. For example, you could enhance the Car
class by adding methods to change its color or model:
public void changeColor(String newColor) { this.color = newColor; }
By using classes and objects in Java, you create a structured way to represent real-world entities, encapsulating their properties and behaviors effectively. This not only enhances readability but also fosters the reusability and maintainability of your code, laying the groundwork for more advanced object-oriented concepts like inheritance and polymorphism.
Inheritance and Polymorphism: Enhancing Code Reusability
Inheritance and polymorphism are two core concepts that significantly enhance code reusability in Java programming. By enabling classes to share behaviors and characteristics, inheritance promotes a hierarchical organization of classes, allowing developers to write cleaner and more maintainable code. On the other hand, polymorphism introduces flexibility by allowing methods to operate on objects of different classes through a unified interface, embracing the power of abstraction.
When a class inherits from another, it gains access to the methods and fields of the parent class, or superclass. This means you can create specialized versions of a class without rewriting code, leading to more efficient development processes. Think a general class called Vehicle from which specific types of vehicles can inherit:
class Vehicle { protected String brand; public Vehicle(String brand) { this.brand = brand; } public void honk() { System.out.println("Beep, beep!"); } }
Here, we define a Vehicle class with a constructor that initializes the brand of the vehicle and a method honk that all vehicles can use. Now, let’s create two subclasses: Car and Bike:
class Car extends Vehicle { public Car(String brand) { super(brand); } @Override public void honk() { System.out.println(brand + " car says: Honk, honk!"); } } class Bike extends Vehicle { public Bike(String brand) { super(brand); } @Override public void honk() { System.out.println(brand + " bike says: Beep, beep!"); } }
In this example, Car and Bike are subclasses of Vehicle. Both classes inherit the honk method, but they override it to provide their unique implementations. That’s polymorphism in action: the same method name behaves differently based on the object invoking it. Let’s see how this works in practice:
public class Main { public static void main(String[] args) { Vehicle myCar = new Car("Toyota"); Vehicle myBike = new Bike("Honda"); myCar.honk(); // Output: Toyota car says: Honk, honk! myBike.honk(); // Output: Honda bike says: Beep, beep! } }
In this scenario, even though both myCar and myBike are declared as Vehicle types, they invoke their respective honk methods appropriate to their actual class instances. This demonstrates the power of polymorphism, as the same function can operate on different object types seamlessly.
Moreover, using inheritance and polymorphism together, you can create collections of objects that share a common superclass. This allows for more dynamic and flexible code. For example, you can maintain a list of various vehicles and call their respective methods without needing to know their exact types:
import java.util.ArrayList; public class VehicleTest { public static void main(String[] args) { ArrayList vehicles = new ArrayList(); vehicles.add(new Car("Ford")); vehicles.add(new Bike("Yamaha")); for (Vehicle vehicle : vehicles) { vehicle.honk(); // Calls the overridden method based on the object's actual type } } }
This versatility not only simplifies the code but also enhances the overall design, making it easier to introduce new vehicle types in the future. Should you wish to add a new type of vehicle, you would simply create a new subclass of Vehicle and override the relevant methods, thus adhering to the DRY (Don’t Repeat Yourself) principle.
Inheritance and polymorphism are powerful OOP mechanisms that facilitate code reusability and flexibility. They empower developers to create a cohesive and easily extensible architecture, essential for managing complex software systems. By understanding and effectively implementing these concepts, you can significantly improve your coding practices in Java.
Encapsulation and Abstraction: Protecting Data in Java
Encapsulation and abstraction are two fundamental principles of object-oriented programming that play an important role in protecting data and simplifying complex systems in Java. Encapsulation involves bundling the data (attributes) and methods (behaviors) that operate on that data into a single unit, a class. This practice helps safeguard an object’s internal state by restricting direct access to its data, thus preventing unintended interference and misuse.
To illustrate this, consider a simple example of a class representing a Person. The attributes of a person, such as name and age, are encapsulated within the class. Access to these attributes is controlled through public methods, often referred to as getters and setters:
class Person { private String name; private int age; public Person(String name, int age) { this.name = name; this.age = age; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { if (age > 0) { this.age = age; } } }
In this example, the name and age attributes are declared private, meaning they cannot be accessed directly from outside the Person class. Instead, the class provides public methods to get and set these values, thus controlling how the data is accessed and modified. This access control enhances data integrity, as it ensures that the age cannot be set to a negative value.
Abstraction complements encapsulation by allowing developers to focus on essential features of an object while hiding the underlying implementation details. By defining abstract classes or interfaces, you can create a blueprint for other classes without exposing the complexities of how certain behaviors are implemented. Think the following example using an abstract class:
abstract class Animal { abstract void makeSound(); } class Dog extends Animal { void makeSound() { System.out.println("Woof!"); } } class Cat extends Animal { void makeSound() { System.out.println("Meow!"); } }
In this scenario, the Animal class defines an abstract method makeSound that must be implemented by any subclass. The actual implementation details for the sounds made by Dog and Cat are encapsulated within those classes. Clients using the Animal class do not need to know how the sound is produced; they only need to call the makeSound method, which demonstrates abstraction:
public class Main { public static void main(String[] args) { Animal myDog = new Dog(); Animal myCat = new Cat(); myDog.makeSound(); // Output: Woof! myCat.makeSound(); // Output: Meow! } }
This approach not only leads to cleaner and more understandable code but also makes it easier to extend the system. If a new animal type needs to be added, you would simply create a new class that extends Animal and implements the makeSound method, thus adhering to the principles of extensibility and maintainability.
By effectively using encapsulation and abstraction, Java developers can create robust systems that protect data while simplifying interactions with complex object behavior. These principles foster an environment where changes can be made with minimal impact on other system components, ensuring that software remains adaptable and resilient over time.
Interfaces and Abstract Classes: Defining Contracts in OOP
In the sphere of object-oriented programming, interfaces and abstract classes define contracts that classes can implement or inherit, respectively. They provide a powerful mechanism to enforce certain behaviors while allowing flexibility in implementation. Understanding the distinction and appropriate use cases for interfaces and abstract classes is essential for designing robust, scalable, and maintainable Java applications.
An interface in Java is a reference type that can contain only constants, method signatures, default methods, static methods, and nested types. It cannot contain instance fields or constructors. The methods declared in an interface are abstract by default, meaning they do not have a body. Any class that implements an interface must provide concrete implementations for all its methods, thereby adhering to the contract laid out by the interface. That is particularly useful for defining a common behavior across disparate classes.
interface Animal { void makeSound(); void eat(); } class Dog implements Animal { public void makeSound() { System.out.println("Woof!"); } public void eat() { System.out.println("Dog is eating."); } } class Cat implements Animal { public void makeSound() { System.out.println("Meow!"); } public void eat() { System.out.println("Cat is eating."); } }
In the example above, the Animal
interface defines two methods: makeSound
and eat
. Both the Dog
and Cat
classes implement this interface, providing their own concrete behaviors for these methods. This allows for polymorphic behavior when using the interface type:
public class Main { public static void main(String[] args) { Animal myDog = new Dog(); Animal myCat = new Cat(); myDog.makeSound(); // Output: Woof! myCat.makeSound(); // Output: Meow! myDog.eat(); // Output: Dog is eating. myCat.eat(); // Output: Cat is eating. } }
Abstract classes, on the other hand, can provide a partial implementation of their methods. An abstract class can have both abstract methods (without a body) and concrete methods (with a body). This allows developers to provide default behavior that can be inherited or overridden by subclasses. Abstract classes are beneficial when you have a base class that should not be instantiated on its own, but still provides some shared functionality.
abstract class Shape { abstract void draw(); // Abstract method public void display() { // Concrete method System.out.println("Displaying shape."); } } class Circle extends Shape { void draw() { System.out.println("Drawing a circle."); } } class Rectangle extends Shape { void draw() { System.out.println("Drawing a rectangle."); } }
In this case, the Shape
abstract class provides a common method display
while requiring subclasses like Circle
and Rectangle
to implement the draw
method. This combination of abstract and concrete methods allows for a mixture of shared functionality and specific behavior:
public class Main { public static void main(String[] args) { Shape myCircle = new Circle(); Shape myRectangle = new Rectangle(); myCircle.draw(); // Output: Drawing a circle. myRectangle.draw(); // Output: Drawing a rectangle. myCircle.display(); // Output: Displaying shape. myRectangle.display(); // Output: Displaying shape. } }
When deciding whether to use an interface or an abstract class, consider the nature of the relationship you want to establish. Use interfaces when you want to define a contract that can be implemented by any class, regardless of its position in the class hierarchy. This is useful for achieving multiple inheritance of type, as a class can implement multiple interfaces. Use abstract classes when you want to share code among closely related classes and provide default behavior while still enforcing some level of restriction.
Interfaces and abstract classes are powerful tools in the Java developer’s toolkit, enabling the creation of flexible and extensible systems. By properly using these constructs, you can ensure your code adheres to principles of good design, such as the Open/Closed Principle, which encourages systems that are open for extension but closed for modification, ultimately leading to more maintainable and adaptable codebases.
Source: https://www.plcourses.com/object-oriented-programming-in-java/