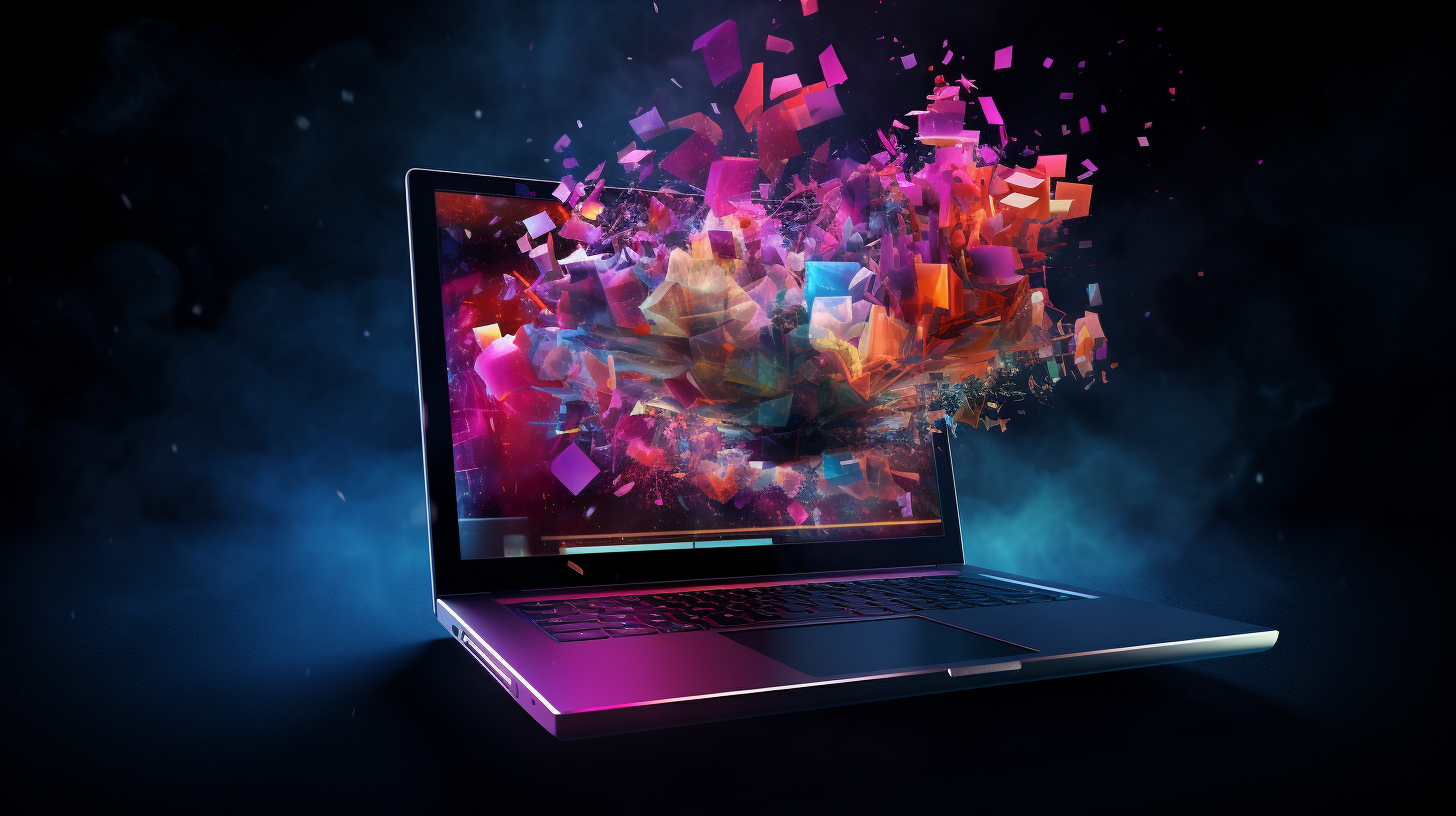
Materialized views in SQL serve as a powerful tool for optimizing query performance, particularly in environments where data is frequently queried but not always updated. Unlike regular views, which are simply stored queries that execute each time they are called, materialized views store the result set of a query physically on disk. This means that when a query is run against a materialized view, the database engine can retrieve the pre-computed results quickly, significantly speeding up response times.
To understand materialized views, it is essential first to grasp how they differ from standard views. A standard view does not store any data itself; it pulls data from underlying tables in real-time, which can lead to performance bottlenecks, especially with complex queries involving multiple joins and aggregations. On the other hand, a materialized view holds a snapshot of the data at a certain point in time. This snapshot can be refreshed periodically, either on demand or through scheduled intervals, depending on the needs of the application.
Here’s how to create a simple materialized view:
CREATE MATERIALIZED VIEW sales_summary AS SELECT product_id, SUM(quantity) as total_quantity, AVG(price) as average_price FROM sales GROUP BY product_id;
In this example, the materialized view named `sales_summary` aggregates sales data by `product_id`, calculating the total quantity sold and the average price. This pre-computation allows users to quickly access summarized data without needing to execute the underlying complex query.
Materialized views can be refreshed manually or automatically. Automatic refreshes can be configured to occur at specific intervals, ensuring that the view remains up-to-date with the underlying data. However, it’s crucial to balance the frequency of refreshes with the performance cost associated with these operations. Frequent refreshing can negate some of the performance benefits provided by the materialized view.
Here’s how to refresh a materialized view:
REFRESH MATERIALIZED VIEW sales_summary;
Understanding materialized views is critical for developers and database administrators who want to unlock the potential for efficient data retrieval in SQL databases. With the right implementation, materialized views can dramatically enhance the performance of read-heavy applications.
Benefits of Using Materialized Views for Query Performance
Using materialized views can lead to substantial improvements in query performance for several reasons. First and foremost, they reduce the computational overhead involved in executing complex queries. When the underlying data is large and the queries require extensive calculations, materialized views provide a shortcut by storing precomputed results. This allows the database engine to bypass the heavy lifting typically required to generate results on-the-fly.
For instance, consider a scenario where an organization frequently generates reports on sales data that involve calculating total sales, average sales prices, and product performance over time. Without a materialized view, the query must recompute these aggregates every time it runs, consuming valuable processing time and resources. By employing a materialized view, the organization can instantly retrieve the already computed results, thus significantly enhancing performance during report generation.
CREATE MATERIALIZED VIEW product_performance AS SELECT product_id, SUM(sale_amount) AS total_sales, COUNT(*) AS total_transactions, AVG(sale_amount) AS average_sale FROM sales GROUP BY product_id;
In this example, the `product_performance` materialized view encapsulates critical sales metrics for each product, enabling quick access to summary data. This not only speeds up the execution of reports but also decreases the load on the underlying sales table, leading to a more efficient database overall.
Furthermore, materialized views improve performance by minimizing the amount of data that needs to be scanned during query execution. For complex queries that filter, aggregate, or join large datasets, materialized views can provide a subset of data that’s much smaller and more manageable. This allows for faster query execution times and a more responsive user experience.
Another significant benefit of materialized views is their ability to optimize network usage. In distributed database systems, where queries might need to traverse multiple databases or servers, materialized views can act as a local cache of remote data. This means that instead of fetching large volumes of data across the network, a materialized view can provide the necessary information from a local perspective, reducing latency and bandwidth consumption.
Moreover, materialized views allow for better resource management during peak loads. In scenarios where multiple users are accessing the same data at once, a materialized view can serve as a dedicated resource, streamlining read requests and ensuring that the main tables are not bogged down by high read activity. That’s particularly beneficial in high-transaction environments such as e-commerce platforms or financial services.
CREATE MATERIALIZED VIEW user_activity_summary AS SELECT user_id, COUNT(*) AS activity_count FROM user_activity WHERE activity_date > NOW() - INTERVAL '30 days' GROUP BY user_id;
By employing the `user_activity_summary` materialized view, an application can quickly access user activity metrics over the past month. This allows for rapid analysis and reporting without placing undue stress on the transactional `user_activity` table.
The benefits of using materialized views for query performance are multi-faceted. They reduce computational costs, decrease data scanning needs, optimize network usage, and enhance resource management. When implemented strategically, materialized views can be a game-changing element for any SQL-based application, enabling quicker query responses and a more efficient overall system.
Strategies for Creating and Managing Materialized Views
Creating and managing materialized views effectively very important for maximizing their performance benefits. There are several strategies that developers and database administrators can employ to ensure that materialized views are both efficient and aligned with the needs of the application.
First, it’s essential to define clear objectives for what data the materialized view should provide. Understanding the types of queries that will leverage the materialized view is key. This informs the design of the view itself, including the selection of columns, the choice of aggregate functions, and the structure of any joins. For example, if a materialized view is designed to support sales reporting, it might include only the most critical metrics and filters that are frequently accessed.
Next, think the frequency of data refreshes. Depending on the application’s requirements, materialized views can be set to refresh automatically at regular intervals or manually at specific times. A strategy that balances the need for up-to-date information with the overhead of maintaining the view is critical. For instance, if the underlying data changes infrequently, a less frequent refresh may suffice:
CREATE MATERIALIZED VIEW sales_summary REFRESH COMPLETE START WITH SYSDATE NEXT SYSDATE + 1/24 AS SELECT product_id, SUM(quantity) AS total_quantity, AVG(price) AS average_price FROM sales GROUP BY product_id;
In this example, the `sales_summary` materialized view is configured to refresh every hour, allowing users to access relatively current data without overburdening the system with constant updates.
Another strategy involves indexing the materialized view. Indexes can significantly enhance query performance by allowing the database engine to locate rows more quickly. Creating indexes on columns that are frequently used in WHERE clauses or joins can lead to substantial performance gains. For instance:
CREATE INDEX idx_sales_summary_product ON sales_summary (product_id);
Additionally, it’s wise to monitor the performance of materialized views regularly. Use database performance metrics to evaluate how well a materialized view is serving its purpose. If a view is not being used as expected, ponder revising its structure or refresh strategy. Monitoring tools can also help identify if a view is becoming stale or if the underlying data is changing more frequently than anticipated, thus necessitating an adjustment in refresh frequency.
In environments with high data volatility or where real-time data access is critical, think implementing incremental refreshes. Incremental refreshes update only the changes since the last refresh rather than recomputing the entire view. This can lead to performance improvements and lower resource usage:
CREATE MATERIALIZED VIEW sales_summary REFRESH FAST ON COMMIT AS SELECT product_id, SUM(quantity) AS total_quantity, AVG(price) AS average_price FROM sales GROUP BY product_id;
The `REFRESH FAST` option here indicates that only the updates made in the underlying `sales` table since the last refresh will be applied to the materialized view. This can greatly reduce the computational load and time required to keep the view updated.
Finally, consider the implications of materialized views on overall database design. While they provide significant benefits, over-reliance on materialized views can lead to complexity, especially when managing dependencies and ensuring that all views remain consistent with the underlying data. It is essential to keep documentation updated and maintain a clear understanding of how each materialized view fits into the broader data architecture.
By strategically creating and managing materialized views through careful design, appropriate refresh strategies, use of indexing, and continuous performance monitoring, organizations can significantly enhance query performance and resource utilization within their SQL databases.
Best Practices for Query Optimization with Materialized Views
When it comes to effectively optimizing queries with materialized views, adhering to best practices is paramount. These practices not only enhance performance but also ensure maintainability and reliability within the database environment. One of the foundational steps is to assess the specific queries that will benefit from the materialized view. This involves analyzing query patterns to determine which aggregations, filters, and joins are commonly used. Tailoring the materialized view to these specific use cases can yield significant performance improvements.
Another critical best practice is the judicious use of indexing on materialized views. Just as with regular tables, appropriate indexing on frequently queried columns can dramatically speed up access times. It’s essential to identify the columns most often involved in WHERE clauses or JOIN conditions and create indexes to support these queries. For example:
CREATE INDEX idx_sales_summary_product ON sales_summary (product_id);
This index on the `sales_summary` materialized view will enable the database engine to retrieve rows associated with specific `product_id` values more efficiently, especially during filtered queries.
Moreover, the refresh strategy for materialized views should be considered carefully. Depending on the nature of the data and the application requirements, the decision between complete and incremental refreshes can significantly impact performance. For frequently changing datasets where real-time querying is critical, incremental refreshes can minimize the load by updating only the rows that have changed since the last refresh:
CREATE MATERIALIZED VIEW sales_summary REFRESH FAST ON COMMIT AS SELECT product_id, SUM(quantity) AS total_quantity, AVG(price) AS average_price FROM sales GROUP BY product_id;
Setting the materialized view to `REFRESH FAST` ensures that only changes are processed, which helps maintain performance without incurring the cost of recomputing the entire dataset.
It’s also advisable to regularly monitor the performance of materialized views. Using database metrics can help identify whether views are being underutilized or if performance is degrading over time. If a materialized view is found to not meet its intended performance goals, ponder revisiting its design or structure. This may involve adjusting the query logic, changing the refresh strategy, or even adding new indexes to boost performance.
Another important aspect of best practices revolves around the balance between using materialized views and the overall database architecture. While they provide substantial benefits, an over-reliance on them can lead to increased complexity, particularly regarding dependencies between different views and the underlying tables. Careful documentation and a clear understanding of the role each materialized view plays within the overall system can alleviate potential issues.
Finally, maintaining a test environment where different configurations of materialized views can be evaluated is highly beneficial. This allows for testing various refresh strategies, index configurations, and structural changes to measure their impacts on query performance. By iteratively refining materialized views based on empirical evidence from testing, developers can achieve optimal performance tailored to their specific workload.
Using materialized views effectively requires a strategic approach to their creation and management. By focusing on query optimization, employing indexing judiciously, selecting appropriate refresh strategies, monitoring performance, and maintaining clarity in database design, organizations can maximize the benefits of materialized views while minimizing associated complexities.
Case Studies: Success Stories of Materialized View Implementation
Within the scope of SQL databases, using materialized views for query optimization has proven successful across various industries. Several organizations have implemented materialized views with remarkable results, showcasing their ability to improve performance and streamline data retrieval. These case studies serve as insightful examples of how materialized views can transform data handling and improve user experience.
One notable example comes from a large retail company that faced challenges with generating sales reports from their vast database. The existing report generation process required complex queries involving multiple joins and aggregations, which resulted in slow response times during peak business hours. To address this, the company created a materialized view that summarized sales data by region and product category. The view pre-computed key metrics, such as total sales and average transaction values, enabling the reporting team to access this data almost instantaneously.
CREATE MATERIALIZED VIEW regional_sales_summary AS SELECT region, category, SUM(sales_amount) AS total_sales, AVG(sales_amount) AS average_sale FROM sales GROUP BY region, category;
By implementing this materialized view, the company reduced the time taken to generate sales reports from several minutes to mere seconds, significantly enhancing productivity and facilitating timely decision-making.
Another success story comes from a financial services firm that required real-time analytics on customer transactions. Their existing database architecture struggled to keep pace with the volume of transactions, leading to slow query responses during critical reporting periods. To optimize their workflow, they introduced a materialized view that aggregated customer transactions on a daily basis.
CREATE MATERIALIZED VIEW daily_transaction_summary AS SELECT customer_id, COUNT(*) AS transaction_count, SUM(transaction_amount) AS total_spent FROM transactions GROUP BY customer_id, transaction_date;
This materialized view allowed the firm to quickly access aggregated transaction data by customer, improving their ability to analyze spending patterns and make data-driven marketing decisions. The switch to a materialized view not only enhanced performance but also improved the overall data availability for the organization.
In the healthcare sector, a hospital network sought to enhance patient care by analyzing admission and discharge data across its facilities. The query load on their database was high, and generating reports for patient flow management took considerable time. They implemented a materialized view that captured and summarized patient admission and discharge metrics.
CREATE MATERIALIZED VIEW patient_flow_summary AS SELECT department_id, COUNT(*) AS total_admissions, COUNT(CASE WHEN discharge_date IS NOT NULL THEN 1 END) AS total_discharges FROM patient_records GROUP BY department_id;
This view allowed hospital administrators to monitor patient flow efficiently, facilitating better resource allocation and improving patient wait times. The ability to generate reports in real time empowered the staff to respond swiftly to patient needs, ultimately leading to enhanced care quality.
These case studies illustrate the transformative power of materialized views in optimizing query performance across various industries. By pre-computing complex aggregations and providing quick access to summarized data, organizations have successfully alleviated query load and improved response times. The strategic implementation of materialized views not only enhances performance but also supports better decision-making and operational efficiency.
Source: https://www.plcourses.com/sql-and-materialized-views-for-query-optimization/