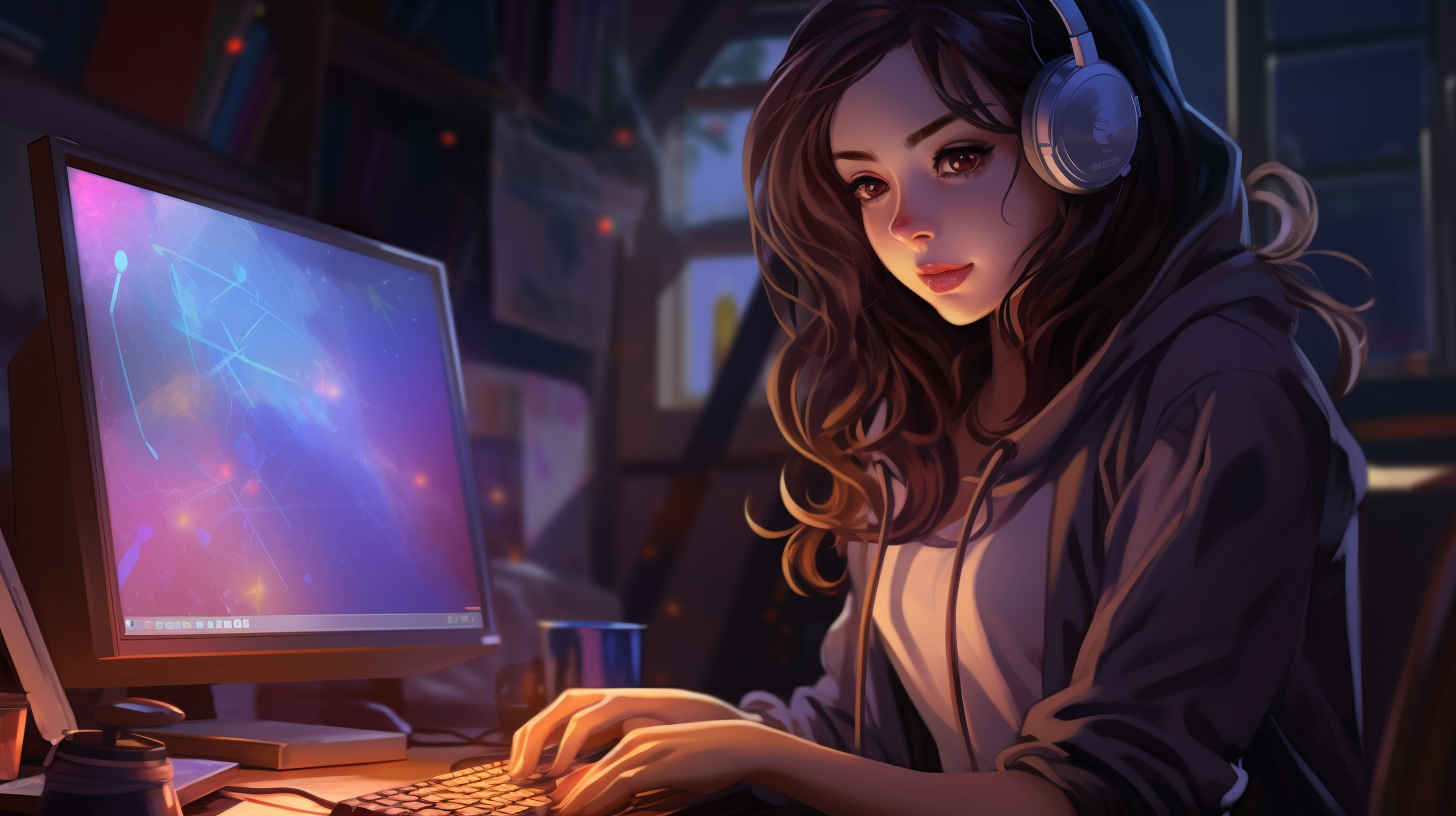
Java serves as the backbone of Android development, providing a robust and versatile programming environment. At its core, Java is an object-oriented programming language, which means it encourages the use of classes and objects to design and implement software. This paradigm aligns closely with the architectural patterns used in Android applications, making Java a natural fit for the platform.
One of the key fundamentals of Java is its syntax, which is both readable and structured. This eases the learning curve for new developers and allows seasoned programmers to express complex ideas succinctly. Java’s strong typing system also helps catch errors at compile time, providing a level of reliability that is important for mobile applications where performance and stability are paramount.
In Android development, Java code is typically organized into Activities and Fragments. An Activity represents a single screen with a user interface, while a Fragment is a modular section of an Activity. Together, they form the backbone of your application’s UI. Understanding how to create and manipulate these components is essential for building functional Android apps.
Another fundamental aspect of Java is its extensive standard library, which provides a plethora of built-in functions for tasks ranging from data manipulation to networking. This library is enriched by the Android SDK, which introduces specific classes and methods tailored for mobile development. For example, handling user inputs and managing UI elements are streamlined through the Android framework.
Here’s a simple Java code example that demonstrates creating a basic Activity in an Android app:
import android.app.Activity; import android.os.Bundle; import android.widget.TextView; public class MainActivity extends Activity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); TextView textView = new TextView(this); textView.setText("Hello, Android!"); setContentView(textView); } }
This code snippet showcases how we define an `Activity` and set a simple `TextView` to display a message. It illustrates the simpler yet powerful nature of Java when applied in the Android ecosystem.
Additionally, error handling in Java is facilitated through exceptions, allowing developers to manage runtime issues gracefully. The use of try-catch blocks enables a robust way to handle errors without crashing the application, ensuring a smoother user experience.
Moreover, Java’s emphasis on code reusability through inheritance and polymorphism helps in creating scalable applications. By defining base classes and extending them, developers can build upon existing code bases without redundancy. This is particularly useful in Android, where you might have multiple Activities that share common behaviors.
Mastering Java fundamentals is pivotal for anyone venturing into Android development. Its object-oriented nature, coupled with a plethora of libraries and frameworks, makes it a powerful tool for creating versatile and efficient mobile applications.
Setting Up Your Development Environment
Setting up your development environment for Android development is an important step to ensure that you can efficiently write, test, and debug your applications. Android development primarily relies on Android Studio, an integrated development environment (IDE) that provides all the tools you’ll need to build Android apps. Here, we will delve into the setup process and the essential configurations required.
To begin, you need to download and install Android Studio. This process is straightforward:
# Download the Android Studio installer for your operating system https://developer.android.com/studio # Follow the installation instructions specific to your OS
After installing Android Studio, you will be prompted to download the necessary components, including the Android SDK (Software Development Kit). The SDK is a collection of tools that allow you to develop Android applications, including libraries, emulators, and debugging tools.
During the installation, Android Studio will also install the Java Development Kit (JDK) if it isn’t already present on your system. The JDK is essential as it provides the Java compiler and runtime environment needed to run Java applications, including your Android apps. It’s important to ensure that you have a compatible version of the JDK. Generally, JDK 8 or later is recommended for working with Android Studio.
Once everything is installed, you can start Android Studio and create a new project. The IDE offers a project wizard to help you set up your workspace. Here’s how to create a new project:
# 1. Open Android Studio # 2. Click on "Start a new Android Studio project" # 3. Choose a project template (e.g., Empty Activity) # 4. Configure your project details (Name, Package name, Save location, Language, etc.) # 5. Click "Finish" to create your project
After your project is created, it is essential to familiarize yourself with the structure of an Android project. Key components include:
- Contains your Java code, organized into packages.
- Houses resources like layouts, strings, and images.
- The manifest file where you declare components and permissions for your app.
To run your application, you’ll need to set up an Android Virtual Device (AVD) if you do not have a physical Android device for testing. The AVD allows you to emulate different devices and Android versions. You can create an AVD by following these steps:
# 1. Open the AVD Manager in Android Studio # 2. Click on "Create Virtual Device" # 3. Select the device type and configuration (screen size, orientation, etc.) # 4. Choose a system image (the Android version you want to emulate) # 5. Click "Finish" to create the AVD
With your AVD set up, you can run your application by selecting the AVD from the run configuration dropdown and clicking the run button. The application will compile, and you will see it running on the emulator, which completes the foundation of your Android development environment.
Setting up your development environment involves installing Android Studio, configuring the Android SDK, and creating a new project. Understanding the project structure and how to use the AVD is essential to streamline your development process. With these components in place, you are ready to explore Android development using Java.
Creating Your First Android App
Creating your first Android app is an exciting and foundational step in your journey as an Android developer. This process not only solidifies your understanding of Java but also introduces you to the Android framework’s components. The following sections will guide you through building a simple app that displays a greeting message. We’ll be using the Activity class, which is critical in Android’s architecture.
To get started, ensure you have your development environment set up as discussed earlier. Once you have Android Studio open and a new project configured, you can focus on the core elements of your app. For this example, we’ll create an app that shows a “Hello, World!” message on the screen.
The first step is to define your main activity. In Android, each screen of your app is represented by an Activity class. You can create the MainActivity as follows:
import android.app.Activity; import android.os.Bundle; import android.widget.TextView; public class MainActivity extends Activity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); TextView textView = new TextView(this); textView.setText("Hello, Android!"); setContentView(textView); } }
In this code snippet, we define our MainActivity
by extending the Activity
class. The onCreate
method is called when the activity is starting. Inside this method, we create a new TextView
object, set its text to “Hello, Android!”, and then call setContentView
to display it on the screen.
After defining the activity, you need to declare it in the AndroidManifest.xml
file. This file is essential as it provides the Android system with information about your app, including its components and permissions. Here’s how you can specify your MainActivity
:
By adding the activity
tag, we inform the Android framework about our MainActivity
. The intent-filter
allows this activity to be launched as the main entry point of the application.
Once you have set up your activity and declared it in the manifest, you’re ready to run your app. Select the emulator or physical device from the run configuration in Android Studio and click the run button. If everything is set up correctly, you should see your app displaying the message “Hello, Android!” on the screen.
This simple example illustrates the essence of Android development using Java. You’ve just created an Activity, manipulated UI components, and configured your app to run. As you progress, you’ll encounter more complex scenarios involving user interactions, data management, and using additional Android components.
Creating your first Android app involves defining an Activity, configuring the AndroidManifest.xml, and running your application. This foundational experience will serve as a stepping stone for more advanced concepts and functionalities in Android development.
Understanding Android Components
Understanding Android components is important for effective Android development, as these elements form the building blocks of any Android application. Each component serves a specific purpose, and knowing how to work with them is essential for creating functional and responsive apps.
At the heart of Android’s architecture are four main types of components: Activities, Services, Broadcast Receivers, and Content Providers. Let’s take a closer look at each of these components and their roles.
Activities are the most visible components of an Android app. They represent a single screen with a user interface and are responsible for interacting with the user. Each Activity is derived from the Activity
class and must implement key lifecycle methods such as onCreate()
, onStart()
, onResume()
, and onPause()
. Understanding the Activity lifecycle is paramount because it defines how an Activity behaves in response to user interactions and system events.
Here’s an example of defining an Activity with lifecycle methods:
import android.app.Activity; import android.os.Bundle; import android.util.Log; public class ExampleActivity extends Activity { private static final String TAG = "ExampleActivity"; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_example); Log.d(TAG, "Activity Created"); } @Override protected void onStart() { super.onStart(); Log.d(TAG, "Activity Started"); } @Override protected void onResume() { super.onResume(); Log.d(TAG, "Activity Resumed"); } @Override protected void onPause() { super.onPause(); Log.d(TAG, "Activity Paused"); } @Override protected void onStop() { super.onStop(); Log.d(TAG, "Activity Stopped"); } @Override protected void onDestroy() { super.onDestroy(); Log.d(TAG, "Activity Destroyed"); } }
The example above logs messages at each stage of the Activity’s lifecycle, which will allow you to see how the system manages its state.
Services are another critical component in Android. They run in the background to perform long-running operations without a user interface. Services are perfect for tasks such as playing audio, downloading files, or handling network transactions. Services can be started or bound to an Activity, and they can run in the foreground or background based on the app’s requirements.
Here’s a brief example of a simple Service:
import android.app.Service; import android.content.Intent; import android.os.IBinder; import android.util.Log; public class ExampleService extends Service { private static final String TAG = "ExampleService"; @Override public void onCreate() { super.onCreate(); Log.d(TAG, "Service Created"); } @Override public int onStartCommand(Intent intent, int flags, int startId) { Log.d(TAG, "Service Started"); // Perform your long-running task here return START_STICKY; } @Override public void onDestroy() { super.onDestroy(); Log.d(TAG, "Service Destroyed"); } @Override public IBinder onBind(Intent intent) { return null; // Return null as that's a started service } }
Next, we have Broadcast Receivers. They are responsible for listening to and reacting to broadcast messages from other applications or from the system itself. This can include system-wide broadcasts announcing events like battery low, network connectivity changes, or custom broadcasts from your own app. Implementing a BroadcastReceiver allows your app to respond to these events accordingly.
Here’s how you can define a simple BroadcastReceiver:
import android.content.BroadcastReceiver; import android.content.Context; import android.content.Intent; import android.util.Log; public class ExampleReceiver extends BroadcastReceiver { private static final String TAG = "ExampleReceiver"; @Override public void onReceive(Context context, Intent intent) { Log.d(TAG, "Broadcast Received: " + intent.getAction()); } }
Finally, Content Providers allow you to manage app data and share it between applications. They provide a standardized interface for data access, making it easy for other apps to query and modify your app’s data in a controlled way. Content Providers are essential when you want to share data across different applications or when handling large datasets.
Understanding these Android components gives you the foundational knowledge to structure your applications effectively. Each component interacts with others to create a cohesive user experience, and mastering them will enable you to build robust and scalable Android applications. As you continue your journey in Android development, using these components efficiently will be key to your success.
Best Practices for Java and Android Programming
When it comes to writing Java and Android applications, adhering to best practices very important for maintaining clean, efficient, and scalable code. These practices not only enhance code readability but also ensure that applications perform well and can be easily maintained or expanded upon in the future.
One of the first best practices is to adhere to the principles of object-oriented programming (OOP). This means using encapsulation, inheritance, and polymorphism effectively. Encapsulation allows you to bundle data and its operations, reducing the risk of unintentional interference. For example, by declaring member variables as private and providing public getter and setter methods, you ensure that your classes maintain control over their internal state. Here’s a simple example:
public class User { private String name; private String email; public String getName() { return name; } public void setName(String name) { this.name = name; } public String getEmail() { return email; } public void setEmail(String email) { this.email = email; } }
Additionally, using inheritance allows you to create a hierarchy of classes that can share common behavior, reducing redundancy in your code. For instance, if you have a base class called `Animal`, you can derive specific types like `Dog` and `Cat` from it:
public class Animal { public void eat() { System.out.println("This animal eats food."); } } public class Dog extends Animal { public void bark() { System.out.println("Woof!"); } }
Another cornerstone of best practices is to keep your code modular. This involves separating concerns and ensuring that each class or method has a single responsibility. For example, if you have an Activity that handles both user input and data processing, think creating separate classes for each responsibility. This modularity enhances testability and maintainability:
public class UserInputHandler { public void collectInput() { // Logic to collect user input } } public class DataProcessor { public void processData() { // Logic to process data } }
In Android development, managing resources efficiently is paramount. Leverage the Android resource management system to separate UI elements from your code. By placing strings, colors, and dimensions in resource files, you make it easier to localize your application and update UI elements without altering the codebase:
// strings.xml My Application // Java code String appName = getString(R.string.app_name);
Another best practice is to handle exceptions properly. Use try-catch blocks to manage potential failure points in your application. This approach helps maintain a smooth user experience, as unhandled exceptions can lead to app crashes:
try { // Potentially risky operation } catch (Exception e) { Log.e("Error", "An error occurred: " + e.getMessage()); }
Furthermore, testing should be an integral part of your development workflow. Writing unit tests for your classes and methods ensures that you can catch bugs early and verify that your code behaves as expected. Android provides testing frameworks like JUnit and Espresso for this purpose:
import org.junit.Test; import static org.junit.Assert.assertEquals; public class UserTest { @Test public void testGetName() { User user = new User(); user.setName("Neil Hamilton"); assertEquals("Neil Hamilton", user.getName()); } }
Lastly, stay updated with the latest Android development practices and libraries, such as using Android Jetpack components. These tools can help simplify common tasks and improve the overall architecture of your applications.
By following these best practices, you will be well-equipped to write robust Java and Android applications that are maintainable, efficient, and adaptable to changes in requirements or technology. Adopting a disciplined approach to coding can significantly enhance both your productivity and the quality of your projects.
Source: https://www.plcourses.com/java-and-android-development-basics/