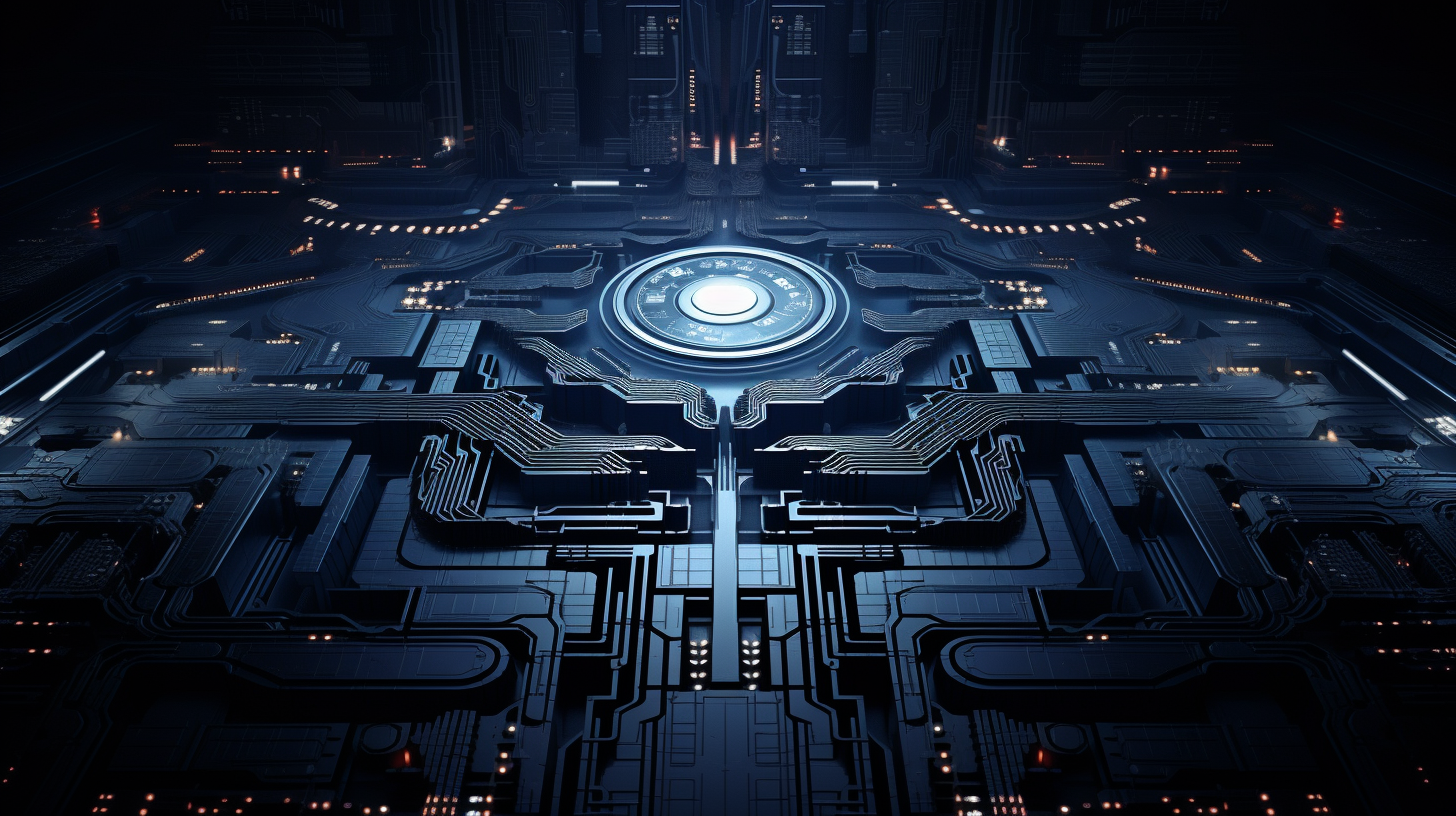
Java serialization is a mechanism that allows you to convert an object into a byte stream, which can then be persisted to a file, sent over a network, or stored in memory for later use. The primary purpose of serialization is to enable the easy transportation and storage of objects, particularly when they are complex structures or contain a lot of data. Understanding how this mechanism works especially important for any Java developer who needs to manage object states across different sessions or environments.
At its core, serialization involves transforming an object’s state into a format that can be easily reconstructed later. This transformation includes the object’s data fields, but it doesn’t include any transient fields, which are meant to be excluded from the serialization process. This feature allows developers to have fine-grained control over which parts of an object are serialized, ensuring that sensitive information or temporary states are not inadvertently stored or transmitted.
Java provides built-in support for serialization through the Serializable
interface, which marks a class as capable of being serialized. When an object of a class that implements this interface is serialized, Java uses reflection to inspect the fields of the object and convert their values into a byte stream. Conversely, during deserialization, Java reconstructs the object from this byte stream, restoring its state.
An important aspect of serialization is the serialVersionUID. This unique identifier is used to verify that the sender and receiver of a serialized object have loaded classes that are compatible with respect to serialization. If the serialVersionUID
does not match, a InvalidClassException is thrown during deserialization. This highlights the need for careful version management of classes that undergo serialization.
Considering its capabilities, serialization is extensively used in various applications, such as:
- Persisting objects to files for later retrieval.
- Sending objects over a network in distributed applications.
- Storing session data in web applications.
To give you an idea of how this works in practice, here’s a simple example of a class that implements the Serializable
interface:
import java.io.Serializable; public class User implements Serializable { private static final long serialVersionUID = 1L; private String username; private String password; // sensitive data, often marked as transient public User(String username, String password) { this.username = username; this.password = password; } // Getters and setters... }
In this example, the User
class is marked as serializable, allowing its instances to be converted into a byte stream. Note that the serialVersionUID
is defined to ensure compatibility across different versions of the class. The password
field, which could be sensitive, should ideally be marked as transient
if you do not want it serialized.
Understanding Java serialization equips developers with the necessary tools to handle object persistence and communication effectively. With this knowledge, you can implement robust applications that leverage the inherent capabilities of the Java serialization mechanism.
How Serialization Works in Java
Serialization in Java works through a systematic process that converts an in-memory object into a stream of bytes. This conversion not only allows for easy storage and transmission but also ensures that the object’s state can be reliably reconstructed later, even in a different environment. The core steps in this process involve writing and reading the object’s state to and from a stream.
When an object is serialized, the Java Virtual Machine (JVM) performs a series of operations. Initially, the serialization process starts with the ObjectOutputStream
class, which is responsible for converting the object into a byte stream. It uses the writeObject()
method, which is called on an instance of ObjectOutputStream
. The JVM traverses the object graph, serializing each field of the object. For every field, the JVM checks its accessibility and whether it can be serialized.
Any fields marked as transient
will be skipped during the serialization process. This allows developers to exclude sensitive information, like passwords or temporary states, from being serialized. However, if an object contains fields that reference other objects, those referenced objects must also be serializable, or a NotSerializableException
will be thrown.
Here’s a basic example demonstrating the serialization process:
import java.io.FileOutputStream; import java.io.IOException; import java.io.ObjectOutputStream; public class SerializationExample { public static void main(String[] args) { User user = new User("Alice", "password123"); try (FileOutputStream fileOut = new FileOutputStream("user.ser"); ObjectOutputStream out = new ObjectOutputStream(fileOut)) { out.writeObject(user); System.out.println("Serialized data is saved in user.ser"); } catch (IOException i) { i.printStackTrace(); } } }
In the above code, a User
object is created and serialized into a file named user.ser
. The ObjectOutputStream
handles the conversion of the object’s state into a byte stream and writes that stream to the specified file.
Deserialization, on the other hand, is the reverse process where the byte stream is converted back into a Java object. This is accomplished using the ObjectInputStream
class. When deserializing, the JVM reads the byte stream and reconstructs the object based on the serialized data. This process also involves checking for the serialVersionUID
to ensure compatibility between the sender and receiver classes.
Here’s how you would implement deserialization:
import java.io.FileInputStream; import java.io.IOException; import java.io.ObjectInputStream; public class DeserializationExample { public static void main(String[] args) { User user = null; try (FileInputStream fileIn = new FileInputStream("user.ser"); ObjectInputStream in = new ObjectInputStream(fileIn)) { user = (User) in.readObject(); System.out.println("Deserialized User: " + user.getUsername()); } catch (IOException | ClassNotFoundException e) { e.printStackTrace(); } } }
In this example, the User
object is read back from the user.ser
file, and the object’s state is restored. Note that the readObject()
method is used to read the serialized object, casting it back to the appropriate type.
This two-way transformation of objects into byte streams and back is pivotal in enabling Java’s serialization capabilities. Understanding this process allows developers to design applications that can save and restore state efficiently, enabling features like caching, state management, and data interchange in networked applications.
Java Serialization API Overview
The Java Serialization API provides the necessary tools and classes to facilitate the serialization and deserialization processes. At the heart of this API are two primary classes: ObjectOutputStream
and ObjectInputStream
. These classes are essential for converting objects into a byte stream and reading objects back from that stream, respectively.
ObjectOutputStream is utilized to serialize an object, writing its state to an output stream. This class extends OutputStream
and provides the writeObject()
method specifically designed for object serialization. When writeObject()
is invoked, the stream captures the entire object graph, writing each object’s state, including its primitive fields and references to other serializable objects.
On the other hand, the ObjectInputStream serves to deserialize objects, reconstructing them from the byte stream. This class extends InputStream
and offers the readObject()
method for reading the serialized object data. The readObject()
method returns an Object
, which needs to be cast back to the original type after reading.
Both classes rely on the serialization mechanism provided by the Serializable interface, allowing developers to mark their custom classes for serialization. Furthermore, they support the Externalizable interface, which gives developers even more control over the serialization process by allowing them to define the writeExternal()
and readExternal()
methods.
Here’s a quick rundown of the key methods and their purpose:
- Writes the specified object to the output stream.
- Reads an object from the input stream and reconstructs it.
- Writes an integer to the stream.
- Reads an integer from the stream.
- Writes a string in a modified UTF-8 format.
- Reads a string in a modified UTF-8 format.
Using these methods allows the developer to create complex serialization schemes tailored to their application’s needs. For instance, implementing the Externalizable interface gives you full control over the serialization process, allowing for custom byte formats and optimized serialization routines.
Here’s an example of a class implementing Externalizable:
import java.io.*; public class ExternalUser implements Externalizable { private static final long serialVersionUID = 1L; private String username; private String password; public ExternalUser() { // No-arg constructor required for Externalizable } public ExternalUser(String username, String password) { this.username = username; this.password = password; } @Override public void writeExternal(ObjectOutput out) throws IOException { out.writeUTF(username); out.writeUTF(password); } @Override public void readExternal(ObjectInput in) throws IOException { username = in.readUTF(); password = in.readUTF(); } // Getters and setters... }
In this example, the ExternalUser
class implements the Externalizable
interface, which requires defining custom methods for serialization. The writeExternal()
method specifies how to serialize the object’s fields, while readExternal()
specifies how to reconstruct the object’s state. This level of control can be crucial when dealing with legacy systems or when optimizing serialization performance.
Java’s Serialization API not only simplifies the task of persisting object states but also allows for customization and fine-tuning, ensuring that developers can meet specific requirements while maintaining data integrity and performance efficiency. By using these powerful tools, Java developers can create robust and scalable applications that can seamlessly handle serialization and deserialization tasks.
Implementing Serializable Interface
To implement the Serializable interface in your Java classes, you simply need to declare the class as implementing the interface. That’s a simpler process, but there are several nuances and best practices to think to ensure effective serialization. The Serializable interface is a marker interface, meaning it does not contain any methods. Its primary purpose is to signify that a class is eligible for serialization. Here’s a concise illustration of how you can implement it:
import java.io.Serializable; public class Person implements Serializable { private static final long serialVersionUID = 123456789L; // Unique identifier for this class private String name; private int age; public Person(String name, int age) { this.name = name; this.age = age; } // Getters and setters... }
In this example, the Person
class is marked with the Serializable
interface. The serialVersionUID
especially important here; it’s a unique version identifier for the class. If you make changes to the class structure (for instance, adding or removing fields), you should update the serialVersionUID
to maintain compatibility with previously serialized objects.
When implementing the Serializable interface, you should also ponder the fields present in your class. All non-transient fields will be serialized by default. However, if you have fields that should not be part of the serialization process—perhaps temporary values or sensitive information like passwords—you should declare them as transient
:
public class Account implements Serializable { private static final long serialVersionUID = 1L; private String accountNumber; private double balance; private transient String password; // This will not be serialized public Account(String accountNumber, double balance, String password) { this.accountNumber = accountNumber; this.balance = balance; this.password = password; } // Getters and setters... }
In the Account
class, the password
field is marked as transient, ensuring it will not be included in the serialization process. That’s particularly important for maintaining security and privacy.
Furthermore, if your class has references to other objects, those objects must also implement the Serializable interface. If any referenced object does not implement Serializable, a NotSerializableException
will be thrown during serialization. This requirement enforces a cascading serialization policy, emphasizing the importance of designing object graphs with serialization in mind.
As you implement serialization in your classes, remember that maintaining the integrity and compatibility of serialized objects is essential. The serialVersionUID plays a pivotal role in this regard, so consider it a best practice to define it explicitly in each Serializable class. This way, you can manage versioning effectively, especially when your application evolves over time.
Implementing the Serializable interface is a critical step in preparing your Java classes for serialization. It opens the door to various functionalities, such as saving the state of an object or transferring it across a network, all while giving you control over which fields get serialized. By adhering to these practices, you can ensure your application remains robust and adaptable to change.
Handling Serialization Exceptions
Handling exceptions during the serialization and deserialization process is an important aspect for any Java developer aiming to build robust applications. Serialization can often lead to various exceptions that need to be managed properly to maintain the integrity and stability of your application. Understanding these exceptions will allow you to write code that can gracefully handle issues that arise during these operations.
The most common exceptions related to serialization include NotSerializableException, InvalidClassException, and IOException. Each of these exceptions serves a specific purpose and indicates a distinct problem that can occur during serialization.
NotSerializableException is thrown when an object this is not serializable is attempted to be serialized. This often happens when a class does not implement the Serializable interface, or if one of its non-transient fields is an object of a class that also does not implement Serializable. To avoid this exception, ensure that all objects within the serializable object graph implement Serializable, or mark problematic fields as transient.
import java.io.Serializable; public class Car implements Serializable { private static final long serialVersionUID = 1L; private String model; private Engine engine; // Engine must also implement Serializable public Car(String model, Engine engine) { this.model = model; this.engine = engine; } // Getters and setters... } class Engine { private String type; // This class does not implement Serializable public Engine(String type) { this.type = type; } }
In this example, trying to serialize the Car object will result in a NotSerializableException because the Engine class does not implement Serializable. To fix this, you would need to make the Engine class serializable:
class Engine implements Serializable { private static final long serialVersionUID = 1L; private String type; public Engine(String type) { this.type = type; } }
Another exception that you might encounter is InvalidClassException. This exception arises when there is a mismatch between the serialVersionUID of the class in the current context and the serialized object. If the class definition has changed (e.g., fields added or removed), and the serialVersionUID remains the same, deserialization may fail, leading to an InvalidClassException. Always ensure to update the serialVersionUID when making changes to a class that has already been serialized.
public class User implements Serializable { private static final long serialVersionUID = 2L; // Incremented after changes private String username; private transient String password; // Sensitive data public User(String username, String password) { this.username = username; this.password = password; } // Getters and setters... }
Finally, IOException is a generic exception that can occur during both serialization and deserialization processes. It can happen due to various reasons, such as issues with I/O operations while writing to or reading from a stream, or file system errors. To handle IOExceptions effectively, you should wrap your serialization and deserialization logic in try-catch blocks and provide appropriate error handling.
public class SerializationExample { public static void main(String[] args) { User user = new User("Bob", "password456"); try (FileOutputStream fileOut = new FileOutputStream("user.ser"); ObjectOutputStream out = new ObjectOutputStream(fileOut)) { out.writeObject(user); } catch (IOException e) { System.err.println("IOException occurred: " + e.getMessage()); } } }
By catching the IOException, you can handle any file-related issues gracefully, ensuring that your application remains functional even when faced with unexpected I/O problems.
Effective handling of serialization exceptions is vital for building resilient Java applications. By anticipating potential issues and implementing robust error-handling mechanisms, you can ensure a smooth serialization process and maintain the integrity of your application’s data management capabilities.
Best Practices for Java Serialization
When dealing with Java serialization, adhering to best practices is important for ensuring that your application performs efficiently, remains maintainable, and upholds data integrity. Here are several best practices that can help you navigate the intricacies of serialization with greater confidence and precision.
1. Define serialVersionUID
Always specify a serialVersionUID
in your Serializable classes. This unique identifier allows the Java serialization mechanism to verify that the sender and receiver have compatible versions of the class. Failing to define this ID or neglecting to update it after modifying the class structure can lead to InvalidClassException
during deserialization.
private static final long serialVersionUID = 1L;
2. Use Transient Wisely
Be judicious about marking fields as transient
. This keyword indicates that a field should not be serialized. Common use cases include sensitive data like passwords or fields that can be easily recalculated. However, be cautious: transient fields will not be restored during deserialization, which may lead to issues if your application relies on this data being present.
private transient String password;
3. Prefer Composition Over Inheritance
When designing your classes, consider using composition over inheritance. If a superclass implements Serializable
and a subclass does not, you might inadvertently expose non-serializable fields. Instead, encapsulate non-serializable fields in a separate class, ensuring that only the necessary attributes are serialized.
class UserProfile { private Address address; // Composition with Address class }
4. Implement Externalizable for Fine Control
If you require precise control over the serialization process, ponder implementing the Externalizable
interface instead of Serializable
. This interface mandates that you define your own write and read methods, so that you can customize the serialization logic fully. This can yield performance benefits in certain scenarios where you can optimize the serialization format.
public class UserProfile implements Externalizable { @Override public void writeExternal(ObjectOutput out) throws IOException { out.writeUTF(name); // Customize serialization } @Override public void readExternal(ObjectInput in) throws IOException { name = in.readUTF(); // Customize deserialization } }
5. Be Cautious with Object References
When serializing an object that contains references to other objects, ensure that all referenced classes are also serializable. If any of these references are not serializable, a NotSerializableException
will be thrown. To avoid this, thoroughly review the entire object graph and verify that all necessary classes implement Serializable
.
class Car implements Serializable { private Engine engine; // Ensure Engine implements Serializable }
6. Handle Exceptions Gracefully
Implement robust exception handling during serialization and deserialization. Use try-catch blocks to catch specific exceptions like IOException
and ClassNotFoundException
, and provide meaningful error messages or fallback mechanisms. This practice not only enhances the user experience but also aids in debugging.
try { // Serialization logic } catch (IOException e) { System.err.println("Serialization error: " + e.getMessage()); }
7. Document Serialization Behavior
Always document the serialization behavior of your classes, including which fields are serialized, which are transient, and any other relevant information. This documentation aids other developers (and your future self) in understanding the implications of serialization and ensures that your code remains maintainable over time.
By following these best practices, you can wield Java serialization effectively and responsibly, paving the way for resilient applications that manage object states with finesse. The careful orchestration of serialization will not only enhance performance but also safeguard data integrity across application lifecycles.
Source: https://www.plcourses.com/java-serialization-object-to-byte-stream/